C++ 中的 boost::split 函数
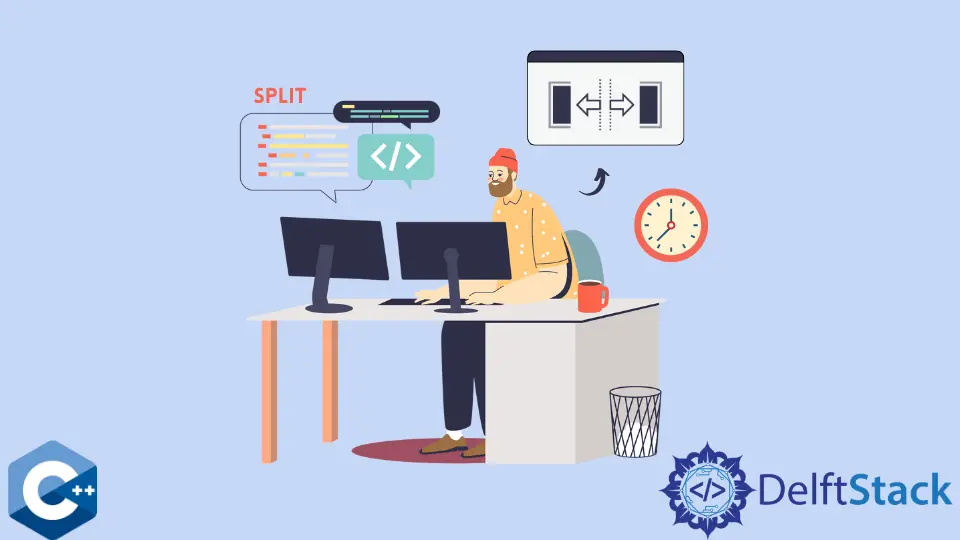
本文将演示如何在 C++ 中使用 boost::split
函数。
使用 boost::split
函数来标记给定的字符串
Boost 提供了强大的工具,可以使用成熟且经过良好测试的库来扩展 C++ 标准库。本文探讨了 boost::split
函数,它是 Boost 字符串算法库的一部分。后者包括几种字符串操作算法,如修剪、替换等。
boost::split
函数将给定的字符串序列拆分为由分隔符分隔的标记。用户应提供将定界符标识为第三个参数的谓词函数。如果给定元素是分隔符,则提供的函数应返回 true
。
在下面的示例中,我们指定了一个 isspace
函数对象来标识给定文本中的空格并将它们拆分为标记。boost::split
还需要一个目标序列容器来存储标记化的子字符串。请注意,目标容器作为第一个参数传递,并且在函数调用后会覆盖其先前的内容。
#include <boost/algorithm/string/split.hpp>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
boost::split(words, text, isspace);
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
Lorem; ipsum; ; dolor; sit; amet,; consectetur; adipiscing; elit.;
当两个或多个分隔符彼此相邻时,前面代码片段中的 boost::split
调用会存储空字符串。不过,我们可以指定第四个可选参数 - boost::token_compress_on
并且相邻的分隔符将被合并,如下例所示。请注意,如果你想成功运行这两个代码片段,则必须在系统上安装 Boost 库。
#include <boost/algorithm/string/split.hpp>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
boost::split(words, text, isspace, boost::token_compress_on);
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
Lorem; ipsum; dolor; sit; amet,; consectetur; adipiscing; elit.;
使用 stringstream
和 getline
函数使用分隔符拆分字符串
或者,可以使用 stringstream
类和 getline
函数用给定的字符分隔符拆分文本。在这种情况下,我们只使用 STL 工具,不需要包含 Boost 头文件。请注意,此代码版本较大,需要额外的步骤来合并相邻的分隔符。
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
char space_char = ' ';
stringstream sstream(text);
string word;
while (std::getline(sstream, word, space_char)) {
words.push_back(word);
}
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
Lorem; ipsum; ; dolor; sit; amet,; consectetur; adipiscing; elit.;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn