在 C++ 中设置浮点数的精度
-
使用
std::setprecision
设置 C++ 中浮点数的精度 -
使用
std::floor
和std::ceil
修改浮点数的精度 -
使用
std::round
和std::lround
修改浮点数的精度
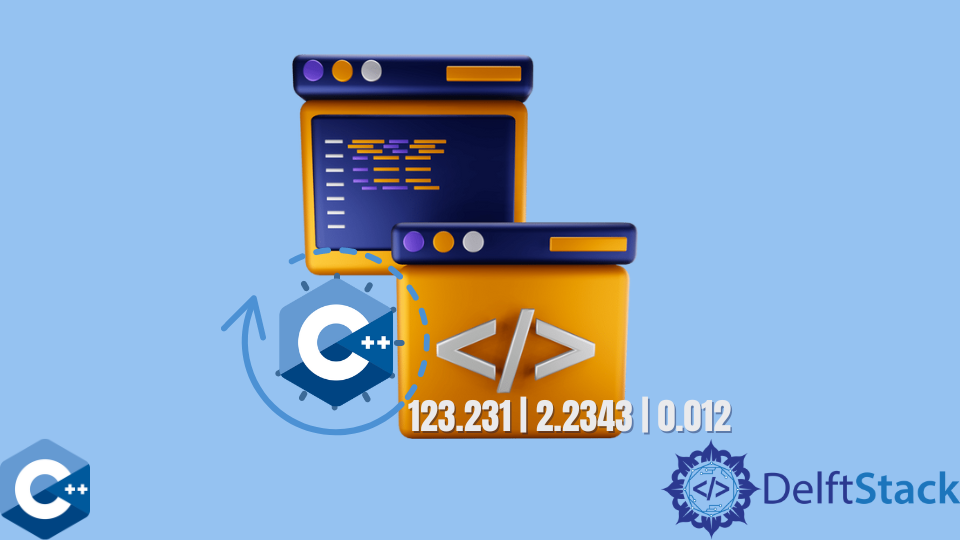
本文将说明几种如何在 C++ 中设置浮点数精度的方法。
使用 std::setprecision
设置 C++ 中浮点数的精度
std::setprecision
是 STL I/O 操作器库的一部分,可用于格式化输入/输出流。setprecision
更改浮点数的精度,并且仅采用一个整数参数来指定要在小数点后显示的数字位数。即,对于浮点数隐式假定的默认精度是逗号后的六位数。但是,当数量太少并且没有使用机械手时,有时可能会用科学记数法来显示浮点数。请注意,这样的数字可能会丢失所有有效数字并显示为零,如以下示例代码所示。
#include <iostream>
#include <iomanip>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::fixed;
using std::setprecision;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << setprecision(3) << i << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
123.231 | 2.234 | 0.012 | 26.949 | 113.000 | 0.000 |
使用 std::floor
和 std::ceil
修改浮点数的精度
std::floor
和 std::ceil
函数由 <cmath>
头文件提供,该头文件最初是在 C 标准库中实现的。ceil
函数计算大于或等于作为唯一参数传递的浮点数的最小整数值。另一方面,floor
会计算小于或等于参数的最大整数值。这些函数是针对 float
,double
和 long double
类型定义的。
#include <iostream>
#include <iomanip>
#include <vector>
#include <cmath>
using std::cout; using std::endl;
using std::vector; using std::fixed;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::ceil(i) << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::floor(i) << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
124.000000 | 3.000000 | 1.000000 | 27.000000 | 113.000000 | 1.000000 |
123.000000 | 2.000000 | 0.000000 | 26.000000 | 113.000000 | 0.000000 |
使用 std::round
和 std::lround
修改浮点数的精度
另外,std::round
和 std::round
可以用来计算最接近零的整数值。这些函数可能会引发与浮点算术相关的错误,这些错误会在页面上进行详细讨论。
#include <iostream>
#include <iomanip>
#include <vector>
#include <cmath>
using std::cout; using std::endl;
using std::vector; using std::fixed;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::round(i) << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::lround(i) << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
123.000000 | 2.000000 | 0.000000 | 27.000000 | 113.000000 | 0.000000 |
123 | 2 | 0 | 27 | 113 | 0 |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn