C++ 中基于范围的 for 循环
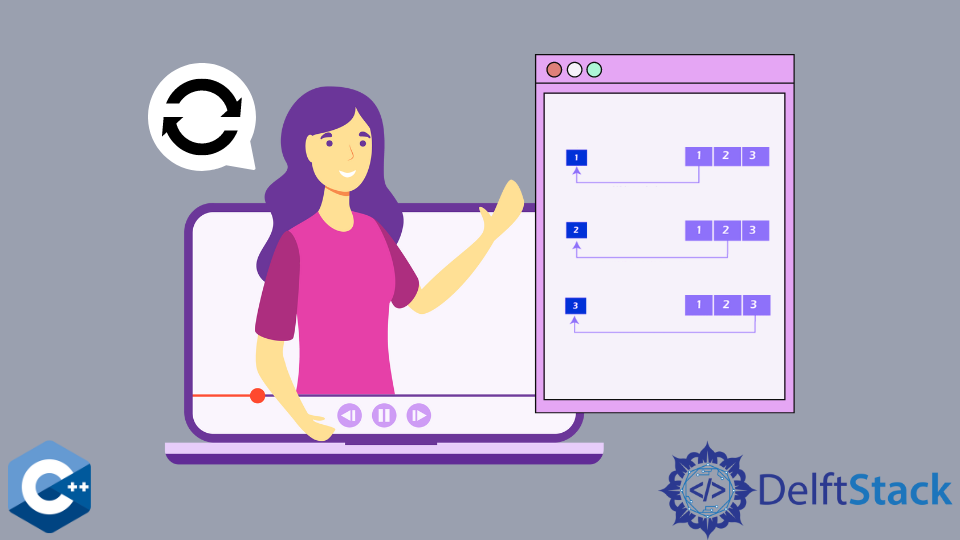
本文将介绍如何在 C++ 中使用基于范围的 for
循环的多种方法。
在 C++ 中使用基于范围的 for
循环来打印 std::map
的元素
基于范围的 for
循环与常规 for
循环的可读性更高。它可用于遍历数组或具有 begin
和 end
成员函数的任何其他对象。请注意,我们使用 auto
关键字和对该元素的引用来访问它。在这种情况下,item
指的是 std::map
的单个元素,恰好是 std::pair
类型的元素。因此,访问键值对需要特殊的点表示法以使用 first
/second
关键字访问它们。
#include <iostream>
#include <map>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::map;
int main() {
map<int, string> fruit_map = {{1, "Apple",},
{2, "Banana",},
{3, "Mango",},
{4, "Cocoa",}};
for (auto &item : fruit_map) {
cout << "[" << item.first << "," << item.second << "]\n";
}
return EXIT_SUCCESS;
}
输出:
[1,Apple]
[2,Banana]
[3,Mango]
[4,Cocoa]
或者,基于范围的循环可以使用结构化绑定符号访问元素,并使代码块更简洁。在下面的示例中,我们演示了这种绑定用法,用于访问 std::map
对。
#include <iostream>
#include <map>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::map;
int main() {
map<int, string> fruit_map = {{1, "Apple",},
{2, "Banana",},
{3, "Mango",},
{4, "Cocoa",}};
for (const auto& [key, val] : fruit_map) {
cout << "[" << key << "," << val << "]\n";
}
return EXIT_SUCCESS;
}
输出:
[1,Apple]
[2,Banana]
[3,Mango]
[4,Cocoa]
使用基于范围的 for
循环在 C++ 中打印 struct
的成员
当遍历的元素表示具有多个数据成员的相对较大的结构时,结构化绑定可能非常有用。如下面的示例代码所示,这些成员被声明为标识符列表,然后直接引用而没有 struct.member
符号。请注意,大多数 STL 容器都可以使用基于范围的 for
循环遍历。
#include <iostream>
#include <list>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::list;
struct Person{
string name;
string surname;
int age;
};
int main() {
list<Person> persons = {{"T", "M", 11},
{"R", "S", 23},
{"J", "R", 43},
{"A", "C", 60},
{"D", "C", 97}};
for (const auto & [n, s, age] : persons) {
cout << n << "." << s << " - " << age << " years old" << endl;
}
return EXIT_SUCCESS;
}
输出:
T.M - 11 years old
R.S - 23 years old
J.R - 43 years old
A.C - 60 years old
D.C - 97 years old
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn