在 C++ 中计算一个数字的位数
-
使用
std::to_string
和std::string::size
函数在 C++ 中对数字中的位数进行计数 -
C++ 中使用
std::string::erase
和std::remove_if
方法对数字进行计数
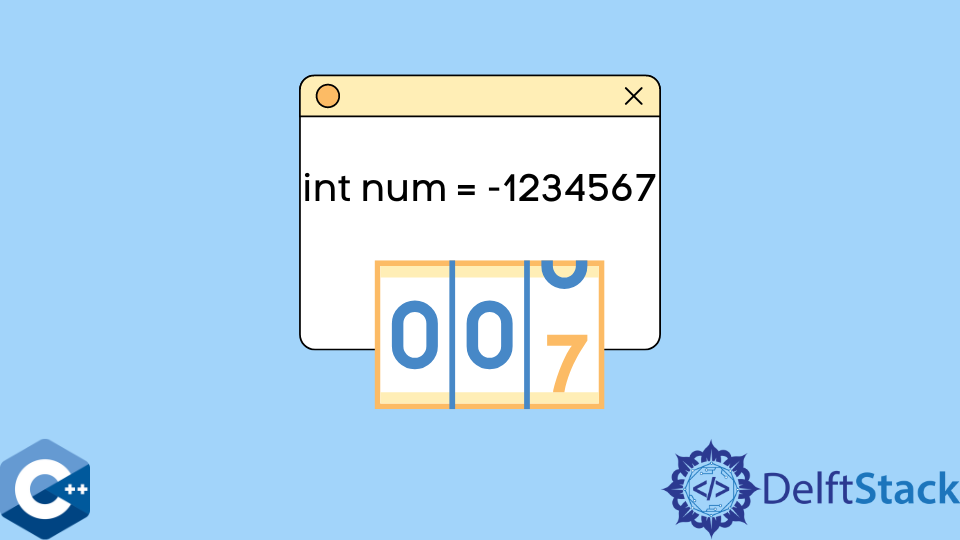
本文将演示有关如何计算 C++ 数字位数的多种方法。
使用 std::to_string
和 std::string::size
函数在 C++ 中对数字中的位数进行计数
计算数字中位数的最直接方法是将其转换为 std::string
对象,然后调用 std::string
的内置函数以检索计数数字。在这种情况下,我们实现了一个单独的模板函数 countDigits
,该函数采用单个参数,该参数假定为整数类型,并以整数形式返回大小。请注意,下面的示例为负整数输出不正确的数字,因为它也计算符号。
#include <iostream>
#include <vector>
#include <string>
using std::cout; using std::cerr;
using std::endl; using std::string;
using std::to_string;
template<typename T>
size_t countDigits(T n)
{
string tmp;
tmp = to_string(n);
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
输出:
number of digits in 1234567 = 7
number of digits in -1234567 = 8
为了弥补之前实现函数 countDigits
的缺陷,我们将添加一个 if
语句来评估给定数字是否为负,并返回少一的字符串大小。请注意,如果数字大于 0
,则返回字符串大小的原始值,如以下示例代码中所实现的。
#include <iostream>
#include <vector>
#include <string>
using std::cout; using std::cerr;
using std::endl; using std::string;
using std::to_string;
template<typename T>
size_t countDigits(T n)
{
string tmp;
tmp = to_string(n);
if (n < 0)
return tmp.size() - 1;
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
输出:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
C++ 中使用 std::string::erase
和 std::remove_if
方法对数字进行计数
前面的示例为上述问题提供了完全足够的解决方案,但是可以使用 std::string::erase
和 std::remove_if
函数组合对 countDigits
进行过度设计,以删除所有非数字符号。还要注意,此方法后续可用来实现可与浮点值一起使用的函数。请注意,以下示例代码与浮点值不兼容。
#include <iostream>
#include <vector>
#include <string>
using std::cout; using std::cerr;
using std::endl; using std::string;
using std::to_string;
template<typename T>
size_t countDigits(T n)
{
string tmp;
tmp = to_string(n);
tmp.erase(std::remove_if(tmp.begin(), tmp.end(), ispunct), tmp.end());
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
输出:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn