如何在 C++ 中使用 setprecision
-
使用
setprecision()
方法为浮点数设置自定义精度 -
使用
setprecision()
和std::fixed()
为浮点数设置自定义精度 -
使用
setprecision()
和std::fixed()
将浮点数对齐到小数点
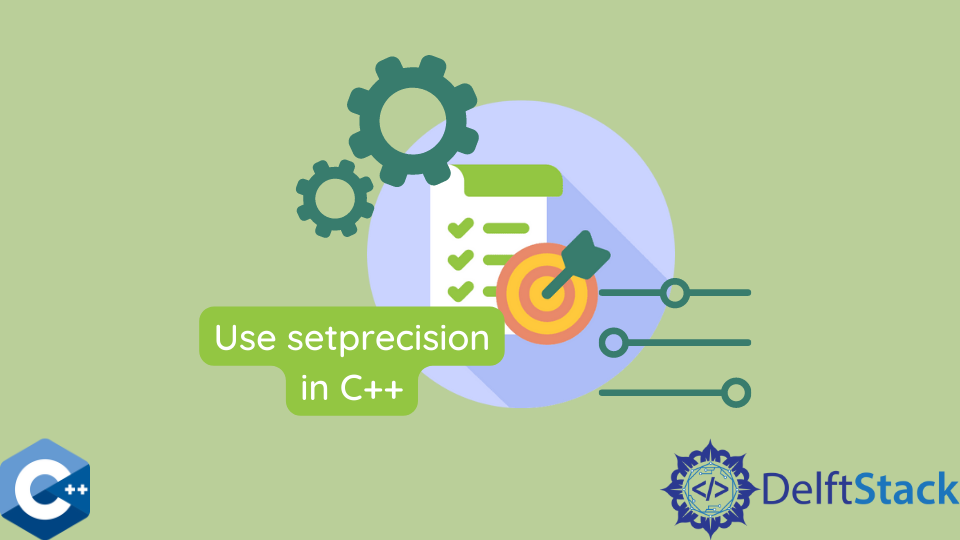
本文将演示关于如何在 C++ 中使用 setprecision
方法的多种方法。
使用 setprecision()
方法为浮点数设置自定义精度
setprecision()
是输入/输出操纵器库 <iomanip>
的一部分,可以用来修改浮点数的默认精度。setprecision()
通常用于带 I/O 流的表达式中。
下面的例子显示了如何为 cout
输出流对象设置浮点数精度。注意,setprecision()
适用于整数(整数部分和分数部分),并且当数字的大小大于指定的精度时会采用科学计数法。
#include <iostream>
#include <iomanip>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::fixed;
using std::setprecision;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013,
92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << setprecision(3) << i << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123 | 2.23 | 0.324 | 0.012 | 26.9 | 1.1e+04 | 92 | 2.34e-07 |
使用 setprecision()
和 std::fixed()
为浮点数设置自定义精度
另外,我们还可以使用 setprecision()
和 fixed()
流操作器联合打印小数点后相同位数的浮点数。fixed()
方法将数字的小数部分设置为固定长度,默认为 6 位。在下面的代码示例中,我们输出到 cout
流,并在将数字插入到输出中之前调用两个操作器。
#include <iostream>
#include <iomanip>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::fixed;
using std::setprecision;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013,
92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << fixed << setprecision(3) << i << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123.231 | 2.234 | 0.324 | 0.012 | 26.949 | 11013.000 | 92.001 | 0.000 |
使用 setprecision()
和 std::fixed()
将浮点数对齐到小数点
最后,我们可以结合 setw
、right
、setfill
、fixed
和 setprecision
操作器来输出向小数点对齐的浮点数。setw
方法以传递的字符数为参数指定输出流宽度。setfill
方法设置一个字符,将未使用的空格填满,right
方法告诉 cout
填充操作适用的一侧。
#include <iostream>
#include <iomanip>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::fixed;
using std::setprecision;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013,
92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << std::setw(10) << std::right
<< std::setfill(' ') << fixed
<< setprecision(4) << i << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
123.2310
2.2343
0.3240
0.0120
26.9491
11013.0000
92.0011
0.0000
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn