如何在 C++ 中初始化向量
Jinku Hu
2020年10月15日
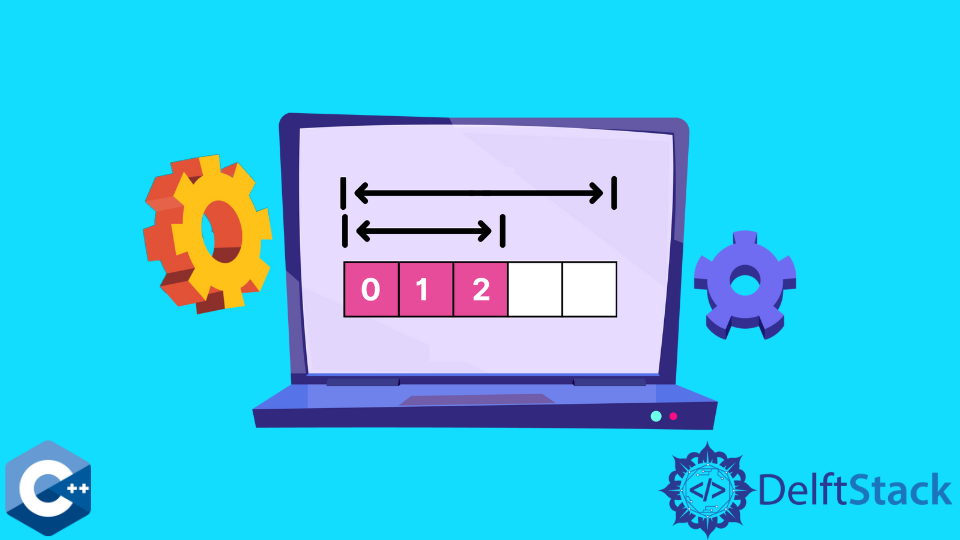
本文将介绍如何在 C++ 中用常量值初始化向量。
在 C++ 中使用初始化器列表符号为向量元素赋定值
这个方法从 C++11 风格开始就被支持,相对来说,它是恒定初始化 vector
变量的最易读的方法。值被指定为一个 braced-init-list
,它的前面是赋值运算符。这种记法通常被称为复制列表初始化。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
int main() {
vector<string> arr = {"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
输出:
one; two; three; four;
另一个方法是在声明 vector
变量时使用初始化-列表构造函数调用,如下面的代码块所示。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
int main() {
vector<string> arr{"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
预处理程序宏是实现初始化列表符号的另一种方式。在本例中,我们将常量元素值定义为 INIT
对象类宏,然后将其赋值给向量 vector
变量,如下所示。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
#define INIT {"one", "two", "three", "four"}
int main() {
vector<string> arr = INIT;
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
C++11 的初始化列表语法是一个相当强大的工具,可用于实例化复杂结构的向量,下面的代码示例就证明了这一点。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
struct cpu {
string company;
string uarch;
int year;
} typedef cpu;
int main() {
vector<cpu> arr = {{"Intel", "Willow Cove", 2020},
{"Arm", "Poseidon", 2021},
{"Apple", "Vortex", 2018},
{"Marvell", "Triton", 2020}};
for (const auto &item : arr) {
cout << item.company << " - "
<< item.uarch << " - "
<< item.year << endl;
}
return EXIT_SUCCESS;
}
输出:
Intel - Willow Cove - 2020
Arm - Poseidon - 2021
Apple - Vortex - 2018
Marvell - Triton - 2020
Author: Jinku Hu
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn