在 C++ 中生成斐波那契数
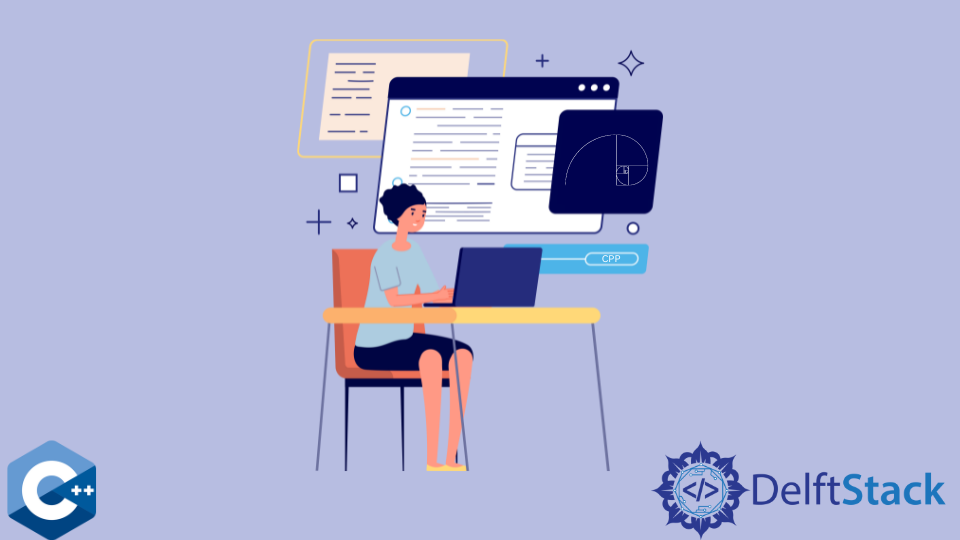
本文将演示如何在 C++ 中生成斐波那契数的多种方法。
C++ 使用迭代方法来打印斐波那契数列中的前 n
项
斐波那契数在数学上通常被称为数字序列,其中每个数字都是从 0
和 1
开头的前两数字之和。在此示例代码中,我们将用户输入作为整数 n
并生成斐波那契数列中的前 n
数字。解决方案是简单的迭代,在此我们初始化起始值并迭代 n-3
次以求和先前的值。每次迭代,总和被打印到 cout
流中,并且存储的值被移动一个数字窗口。注意,实现了 if
条件,该条件检查输入整数 0
并打印相应的值。第一个 cout
语句处理 n
等于 2
时的情况,因为 for
循环条件的结果为 false
,而不会继续。
#include <iostream>
using std::cout; using std::cin;
using std::endl; using std::string;
void generateFibonacci(unsigned long long n)
{
if (n == 1) {
cout << 0 << endl;
return;
}
unsigned long long a = 0;
unsigned long long b = 1;
unsigned long long c;
cout << a << " " << b;
for (unsigned long long i = 3; i <= n; i++)
{
c = a + b;
cout << " " << c;
a = b;
b = c;
}
}
int main()
{
unsigned long long num;
cout << "Enter the number of items to generate Fibonacci series: ";
cin >> num;
generateFibonacci(num);
return EXIT_SUCCESS;
}
输出:
Enter the number of items to generate Fibonacci series: 10
0 1 1 2 3 5 8 13 21 34
C++ 使用迭代方法打印斐波那契数列中的第 n 个项
另外,我们可以实现一个函数,该函数返回斐波那契数列中的第 n 个数字。注意,我们将用户输入存储在 unsigned long long
中,因为我们希望能够输出内置 C++ 类型可以存储的最大数字。该函数以 if-else
语句开始,以检查前三种情况并返回硬编码值。另一方面,如果 n
大于 3
,我们将循环循环并迭代直到获得序列中的第 n 个数字。
#include <iostream>
using std::cout; using std::cin;
using std::endl; using std::string;
unsigned long long generateFibonacci(unsigned long long n)
{
if (n == 1) {
return 0;
} else if (n == 2 || n == 3) {
return 1;
}
unsigned long long a = 1;
unsigned long long b = 1;
unsigned long long c;
for (unsigned long long i = 3; i < n; i++)
{
c = a + b;
a = b;
b = c;
}
return c;
}
int main()
{
unsigned long long num;
cout << "Enter the n-th number in Fibonacci series: ";
cin >> num;
cout << generateFibonacci(num);
cout << endl;
return EXIT_SUCCESS;
}
输出:
Enter the n-th number in Fibonacci series: 10
34
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn