在 C++ 中使用构造函数对分数进行算术运算
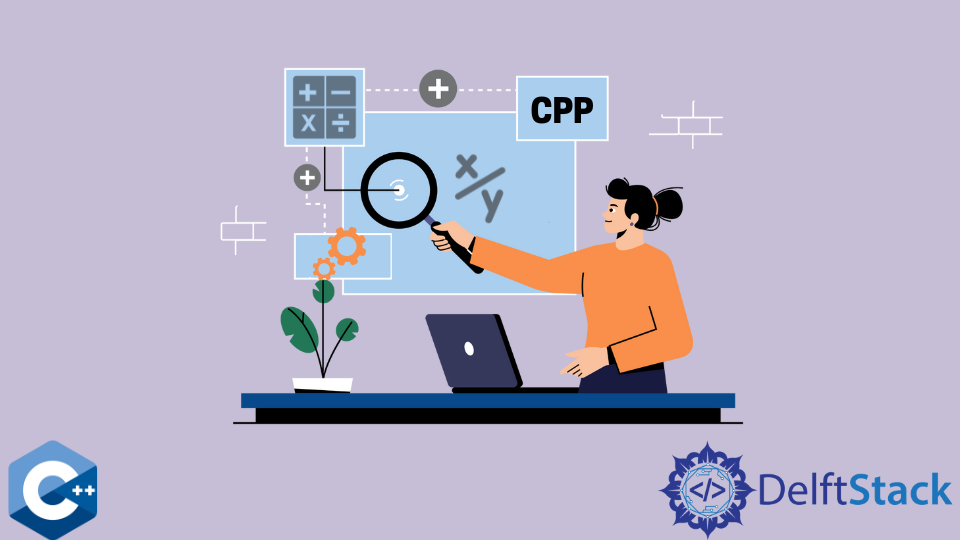
本文将讨论如何使用构造函数创建分数,找到 GCD 以减少分数倍数,并对它们执行计算。
在 C++ 中使用构造函数对分数进行算术运算
读者必须了解程序的先决条件。要使用构造函数创建分数,
- 分母不能为零;
- 如果分子和分母都可整除,则必须将其约简到其最小倍数;
- 程序必须设计成通过新的数据成员存储和显示结果,而预先存在的变量不得改变。
这些条件将使程序可行并能够正确计算分数。
在 C++ 中声明构造函数并初始化其数据成员
构造函数与不同的成员方法交互并相互传递值。任何构造函数程序的第一步是加载导入包并声明默认构造函数和参数化构造函数。
- 导入包。
#include <iostream>
- 成员方法
reduce()
将用于将分数减少到其最低倍数。
int 减少(int m,int n);
命名空间标准
用于iostream
包。
使用命名空间标准;
- 类名必须与成员方法同名。
类计算分数
- 默认构造函数
private
:具有分数的分子和分母的两个数据成员。
{
私人的:
int 背线;
int 底线;
- 参数化构造函数
public:
之后声明。默认构造函数的数据成员在此处使用两个具有相同数据类型(int tl = 0, int bl = 1)
的新变量进行初始化。
构造函数类有五个成员方法。第一个。computefraction()
,与类名共享相同的名称,并存储减少前后的分数值。
在将值传递给其他成员方法之前,需要减少分数。为 4 种运算符计算声明了四个成员方法 - 加法、减法、乘法和除法。
声明的另外两个成员方法读取分数并打印它们。
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
下面是程序第一部分的表示。
#include <iostream>
int reduce(int m, int n);
using namespace std;
class computefraction
{
private:
int topline;
int bottomline;
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
实现成员方法以在 C++ 中执行操作
本文的这一部分实现了在 C++ 中对分数执行算术运算的成员方法。
C++ 中的分数类运算符重载
在这个程序中,运算符在每个成员方法中都被重载,以将两个分数的值放入一个对象中。当使用用户定义的数据类型而不是内置的数据类型时会进行重载,并且需要更改其返回类型。
这是通过::
范围解析运算符实现的。它从特定类访问构造函数的局部变量。
分数的值存储在构造函数 computefraction
中。程序会将这些值传递给其他成员方法以对它们执行算术运算,但首先,需要减少分数才能将其提供给其他成员方法。
语法 computefraction::computefraction(int tl, int bl)
用于访问构造函数 computefraction
的局部变量。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl)
类似地,在添加两个分数的成员方法中,运算符 sum
被重载以适合两个分数的值。
语法:
return_method class_name::operator(argument)
该语法从类 computefraction
访问运算符 sum
,用复制对象 b2
重载它,并将最终结果返回到构造函数 computefraction
。
computefraction computefraction::sum(computefraction b2) const
C++ 中查找分数的 GCD 的成员方法
函数 gcd
找到 GCD 以减少分数倍数。分子和分母是两个数据成员 int m
和 int n
。
该函数首先检查该值是负数还是正数。如果分子或分母中的任何一个为负值,则使用 m = (m < 0) ? -m : m;
来变成正值。
该函数比较变量 m
和 n
之间的较高值以找到用于减少分数倍数的 GCD。如果较大的值存储在变量 n
中,它与 m
交换。
该函数的设计使得较高的值将始终存储在变量 m
中。该函数从较高的值中减去较低的值,直到 m
减少到零。
最后,变量 n
中留下的值是 GCD,它被返回。
int gcd(int m, int n)
{
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0)
{
if (m < n)
{
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
C++ 中一个分数的分子和分母减少的成员方法
通过将分子和分母除以其 GCD 来减少分数。
这里初始化了两个变量,分子的 topline
和分母的 bottomline
。
if-else
条件检查变量 bottomline
值。如果值为零,则程序退出并显示错误消息。
一个整数变量 reduce
被初始化以存储从方法 gcd
返回的两个数字的 GCD。
然后,存储在 reduce
中的值从 topline
和 bottomline
中分离出来,并将结果存储回相同的变量。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl==0){
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
}
else
bottomline = bl;
int reduce = gcd(topline, bottomline);
topline /= reduce;
bottomline /= reduce;
}
创建成员方法以在 C++ 中添加两个分数
用于计算操作的所有方法都创建了一个复制对象,如复制构造函数。这是通过语法 computefraction computefraction::sum(bounty b2) const
完成的。
关键字 bounty b2
创建了一个复制对象 b2
。制作复制对象是在不初始化额外变量的情况下重用第二部分的对象。
添加两个分数 a/b 和 c/d 的方程式是,
$$
e = \frac {a \cdot d + c \cdot b } {b \cdot d}
$$
变量 int tl
存储分子的结果,而 int bl
存储分母的结果。最后,通过了 tl
和 bl
。
computefraction computefraction::sum(bounty b2) const
{
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
其他成员方法以相同的方式创建。要检查,读者可以到本文的最终程序。
如何在 C++ 中使用构造函数实现主函数
一旦创建了所有成员方法,就需要编写 main()
函数,以便程序可以返回传递的值并显示它们。
该程序的编写方式必须使最终用户按以下顺序获得选择和响应:
- 选择运算符的选项,即加法、减法等。
- 如果选择无序并且程序重新加载,则抛出异常。
- 当给出正确的选择时,要求用户输入第一部分的分子和分母,然后是第二部分。
- 如果在分母中插入零并退出,则抛出错误异常。
- 如果输入正确,程序将结果作为最终响应。
- 程序重新加载到步骤 1。
Main 函数的局部变量和构造函数对象的初始化
作为一个菜单驱动程序,声明了一个字符变量 ch
。声明了三个对象:up
传递和存储第一个分数,down
保存第二个分数,final
显示两个分数的结果。
int main()
{
char ch;
computefraction up;
computefraction down;
computefraction final;
}
使用 Do While
循环实现菜单驱动条件
该程序使用 5 个菜单驱动案例和一个 do-while
循环下的默认案例。前四种情况执行计算操作,而第五种情况保留用于退出选项。
default
将被定义为超出范围的选择。程序循环运行,直到用户选择第 5 种情况跳出并结束程序。
do
{
switch (ch)
{
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
.
.
.
case '5':
break;
default:
cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5');
使用 C++ 中的构造函数计算分数的完整代码
//import package
#include <iostream>
//member method to find gcd, with two data members
int gcd(int m, int n);
using namespace std;
class computefraction
{
// Default constructor
private:
int topline;
int bottomline;
// Parameterized Constructor
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
// Member methods of class type bounty.
// In constructors, the class and constructor names must be the same.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl)
{
if (bl==0)
{
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
}
else
bottomline = bl;
//Below codes reduce the fractions using gcd
int reduce = gcd(topline, bottomline);
topline /= multiple;
bottomline /= multiple;
}
// Constructor to add fractions
computefraction computefraction::sum(computefraction b2) const
{
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to subtract fractions
computefraction computefraction::minus(computefraction b2) const
{
int tl = topline * b2.bottomline - b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to multiply fractions
computefraction computefraction::mult(computefraction b2) const
{
int tl = topline * b2.topline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to divide fractions
computefraction computefraction::rem(computefraction b2) const
{
int tl = topline * b2.bottomline;
int bl = bottomline * b2.topline;
return computefraction(tl, bl);
}
// Method to print output
void computefraction::show() const
{
cout << endl << topline << "/" << bottomline << endl;
}
//Method to read input
void computefraction::see()
{
cout << "Type the Numerator ";
cin >> topline;
cout << "Type the denominator ";
cin >> bottomline;
}
//GCD is calculated in this method
int gcd(int m, int n)
{
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0)
{
if (m < n)
{
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
//Main Function
int main()
{
char ch;
computefraction up;
computefraction down;
computefraction final;
do
{
cout << " Choice 1\t Sum\n";
cout << " Choice 2\t Minus\n";
cout << " Choice 3\t multiply\n";
cout << " Choice 4\t Divide\n";
cout << " Choice 5\t Close\n";
cout << " \nEnter your choice: ";
cin >> ch;
cin.ignore();
switch (ch)
{
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '2':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.minus(down);
final.show();
break;
case '3':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '4':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.mult(down);
final.show();
break;
case '5':
break; //exits program.
default:
cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5'); //program stops reloading when choice 5 is selected
return 0;
}
输出:
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 2
first fraction: Type the Numerator 15
Type the denominator 3
Second fraction: Type the Numerator 14
Type the denominator 7
3/1
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 3
first fraction: Type the Numerator 5
Type the denominator 0
Second fraction: Type the Numerator 6
Type the denominator 8
You cannot put 0 as a denominator
--------------------------------
Process exited after 47.24 seconds with return value 0
Press any key to continue . . .