C 语言中的排序函数
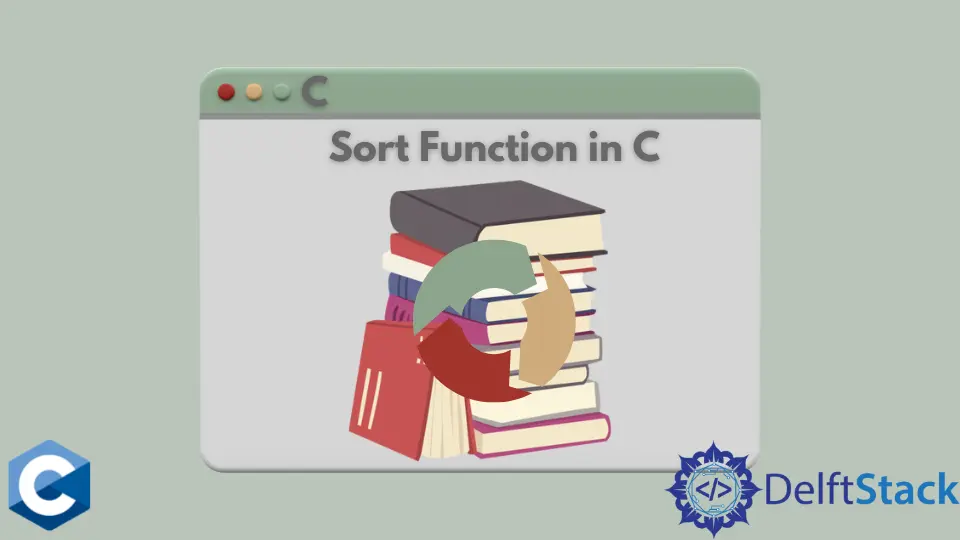
本文将介绍几种在 C 语言中使用标准库排序函数的方法。
使用 qsort
函数对 C 语言中的整数数组进行排序
qsort
函数为不同的数据元素数组实现了某种通用的排序操作。也就是说,qsort
将函数指针作为第四个参数,传递给定元素数组的比较函数。在这种情况下,我们实现了 intCompare
函数来使用 qsort
比较整数组。注意,intCompare
应该具有 qsort
原型指定的类型 - int (*compar)(const void *, const void *)
。因此,我们首先将 p1
/p2
参数转换为 int
指针,然后再去引用它们来访问值本身。如果第一个参数小于另一个参数,比较函数的返回值必须是小于 0 的整数,如果前者大于后者,则返回大于 0 的整数,而相等则返回 0。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static int intCompare(const void *p1, const void *p2) {
int int_a = *((int *)p1);
int int_b = *((int *)p2);
if (int_a == int_b)
return 0;
else if (int_a < int_b)
return -1;
else
return 1;
}
void printIntegers(int arr[], size_t size) {
for (size_t i = 0; i < size; i++) printf("%4d | ", arr[i]);
printf("\n");
}
int main(int argc, char *argv[]) {
int arr2[] = {53, 32, 12, 52, 87, 43, 93, 23};
printIntegers(arr2, 8);
qsort(arr2, 8, sizeof(int), intCompare);
printIntegers(arr2, 8);
exit(EXIT_SUCCESS);
}
输出:
53 | 32 | 12 | 52 | 87 | 43 | 93 | 23 |
12 | 23 | 32 | 43 | 52 | 53 | 87 | 93 |
使用 qsort
函数对 C 语言中的字符串数组进行排序
qsort
可以按升序对字符串数组进行排序,strcmp
作为比较函数。在这种情况下,我们声明并初始化了 char
指针数组,只要调用 qsort
函数,就可以对其元素进行排序。请注意,铸造和去引用是比较函数的必要部分,因为它将两个参数都作为 void
指针类型。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static int stringCompare(const void *p1, const void *p2) {
return strcmp(*(char *const *)p1, *(char *const *)p2);
}
void printStrings(char *arr[], size_t size) {
for (size_t i = 0; i < size; i++) printf("%10s | ", arr[i]);
printf("\n");
}
int main(int argc, char *argv[]) {
char *arr[] = {"jello", "hello", "mello", "zello", "aello"};
printStrings(arr, 5);
qsort(arr, 5, sizeof(char *), stringCompare);
printStrings(arr, 5);
exit(EXIT_SUCCESS);
}
输出:
jello | hello | mello | zello | aello |
aello | hello | jello | mello | zello |
或者,我们可以重新实现前面的示例代码,让用户提供带有程序参数的字符串数组,然后将排序后的数组打印出来作为输出。这一次,在继续调用 qsort
之前,必须检查是否有足够的参数传递给排序。注意,stringCompare
函数直接返回 strcmp
调用的值,因为后者与 qsort
的比较函数的返回值规格相同。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static int stringCompare(const void *p1, const void *p2) {
return strcmp(*(char *const *)p1, *(char *const *)p2);
}
void printStrings(char *arr[], size_t size) {
for (size_t i = 0; i < size; i++) printf("%10s | ", arr[i]);
printf("\n");
}
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: ./program string_0 string_1 string_2...\n");
exit(EXIT_FAILURE);
}
printStrings(argv + 1, argc - 1);
qsort(argv + 1, argc - 1, sizeof(char *), stringCompare);
printStrings(argv + 1, argc - 1);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn