在 Angular 中使用 TypeScript 的 getElementById 替换
-
TypeScript 中的
document.getElementById()
方法 -
在 Angular 中使用 TypeScript 使用
ElementRef
作为getElementById
替换
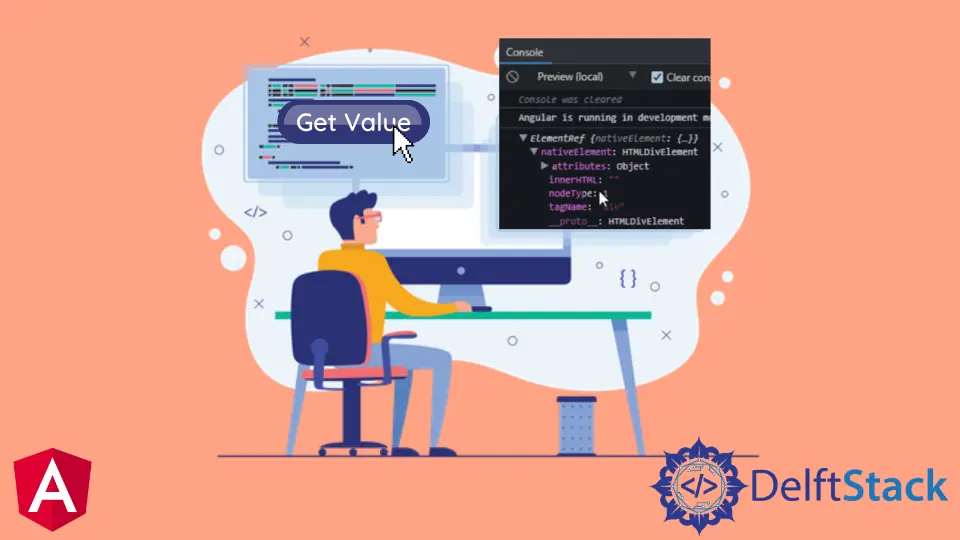
本教程指南提供了使用 TypeScript 在 Angular 中替换 document.getElementById
的简要说明。
这还通过代码示例提供了用于在 Angular 中 getElementById
的最佳方法。我们还将了解 TypeScript 中 DOM querySelector 的用途。
首先,让我们了解一下 TypeScript 中的 document.getElementById
方法以及它在开发人员社区中流行的原因。
TypeScript 中的 document.getElementById()
方法
document.getElementById()
是一个预定义的 JavaScript(以及 TypeScript)方法,可用于操作 document
对象。它返回一个具有指定值的元素,如果该元素不存在,则返回 null
。
document.getElementById()
是开发人员社区中用于 HTML DOM 的最常见和流行的方法之一。
要记住的一个重要点是,如果两个或多个元素具有相同的 id,document.getElementById()
将返回第一个元素。
现在,让我们看一些编码示例,以更好地了解它们的目的和用法。
考虑带有一些具有唯一 demo
id 的文本的 h1
标签。现在,在 scripts
部分中,如果我们想要针对特定标签,我们使用 document
对象中可用的 getElementById()
方法。
<!DOCTYPE html>
<html>
<body>
<h1 id="demo">The Document Object</h1>
<h2>The getElementById() Method</h2>
<script>
document.getElementById("demo").style.color = "red";
</script>
</body>
</html>
输出:
现在让我们考虑一个更动态的例子:一个输入字段接受一个数字并返回它的立方值。
<!DOCTYPE html>
<html>
<body>
<form>
Enter No:<input type="text" id="number" name="number"/><br/>
<input type="button" value="cube" onclick="getcube()"/>
</form>
<script>
function getcube(){
var number=document.getElementById("number").value;
alert(number*number*number);
}
</script>
</body>
</html>
输出:
现在,让我们看看使用 TypeScript 在 Angular 中的 getElementById
替换。
在 Angular 中使用 TypeScript 使用 ElementRef
作为 getElementById
替换
AngularJs 的 ElementRef
是 HTML 元素的包装器,包含属性 nativeElement
并保存对底层 DOM 对象的引用。使用 ElementRef
,我们可以使用 TypeScript 在 AngularJs 中操作 DOM。
通过使用 ViewChild
,我们可以在组件类中获取 HTML 元素的 ElementRef
。Angular 允许在构造函数中请求组件的指令元素时注入 ElementRef
。
使用 @ViewChild
装饰器,我们使用类中的 ElementRef
接口来获取元素引用。
考虑以下 main.component.html
文件中 AngularJs
中的代码示例。我们有一个带有事件的按钮和一个具有唯一 ID myName
的 div:
# angular
<div #myName></div>
<button (click)="getData()">Get Value</button>
输出:
示例 1(app.component.ts
):
#angular
import { Component, VERSION, ViewChild, ElementRef } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./main.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
name = "Angular " + VERSION.major;
@ViewChild("myName") myName: ElementRef;
getData() {
console.log(this.myName);
this.myName.nativeElement.innerHTML = "I am changed by ElementRef & ViewChild";
}
}
输出:
使用 @ViewChild
装饰器,我们在类中的 ElementRef
接口的帮助下获取输入的引用。然后,我们使用带有此引用的 getData()
函数更改其值。
示例 2(app.component.ts
):
import { Component, ViewChild, ElementRef, AfterViewInit } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent implements AfterViewInit {
name = "Angular";
@ViewChild("ipt", { static: true }) input: ElementRef;
ngAfterViewInit() {
console.log(this.input.nativeElement.value);
}
onClick() {
this.input.nativeElement.value = "test!";
}
}
输出:
这将获取输入字段的引用并在单击按钮时更改其内部文本。
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn