Pandas DataFrame DataFrame.set_index() 函数
Suraj Joshi
2023年1月30日
2020年6月17日
-
pandas.DataFrame.set_index()
方法的语法 -
示例代码:用 Pandas
DataFrame.set_index()
方法设置 Pandas DataFrame 索引 -
示例代码:在 Pandas
DataFrame.set_index()
方法中设置drop=False
-
示例代码在 Pandas
DataFrame.set_index
方法中设置inplace=True
-
示例代码:使用 Pandas
DataFrame.set_index()
方法设置多索引列 -
示例代码:Pandas
Dataframe.set_index()
在verify_integrity
为True
时的行为
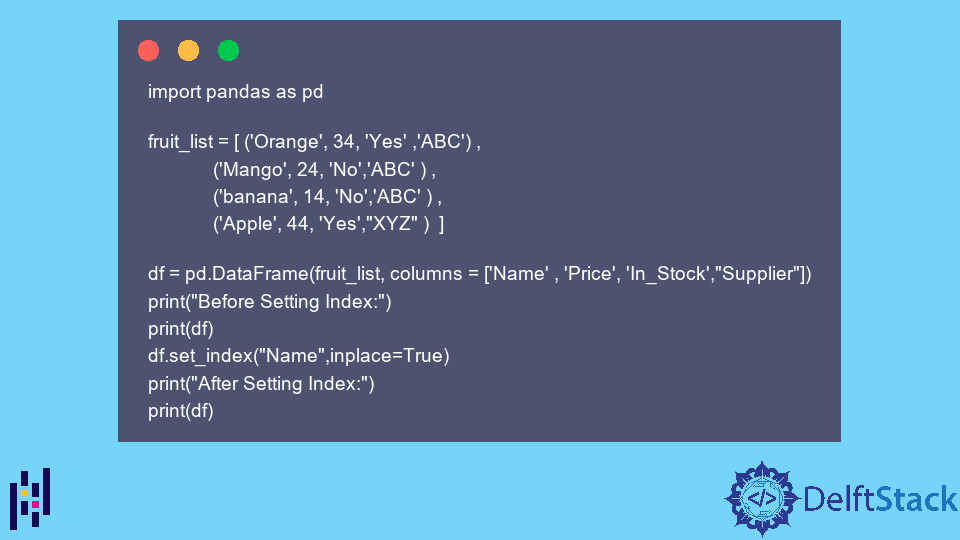
pandas.DataFrame.set_index()
方法可以用来设置适当长度的数组或列作为 DataFrame 的索引,即使在 DataFrame 创建后也可以使用。新设置的索引可以替代现有的索引,也可以在现有索引的基础上进行扩展。
pandas.DataFrame.set_index()
方法的语法
DataFrame.set_index(keys,
drop=True,
append=False,
inplace=False,
verify_integrity=False)
参数
keys |
列或列列表作为索引设置 |
drop |
布尔型。默认值为 True ,删除要设置为索引的列 |
append |
布尔型。默认值是 False ,它指定是否将列追加到现有的索引中 |
inplace |
布尔型。如果 True ,则在原地修改调用者的 DataFrame |
verify_integrity |
布尔型。如果是 True ,在创建有重复的索引时引发 ValueError 。默认值是 False |
返回值
如果 inplace
为 True
,则返回一个带有修改过的索引列的 DataFrame
对象;否则为 None
。
示例代码:用 Pandas DataFrame.set_index()
方法设置 Pandas DataFrame 索引
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name")
print(df_modified)
输出:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
4 Pineapple 64 No XYZ
5 Kiwi 84 Yes XYZ
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
Pineapple 64 No XYZ
Kiwi 84 Yes XYZ
原始的 Dataframe
将数字范围作为默认的索引列,而在 modified_df
中,我们使用 set_index()
方法设置 Name
列作为索引。
示例代码:在 Pandas DataFrame.set_index()
方法中设置 drop=False
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name",drop=False)
print(df_modified)
输出:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
Name Price In_Stock Supplier
Name
Orange Orange 34 Yes ABC
Mango Mango 24 No ABC
banana banana 14 No ABC
Apple Apple 44 Yes XYZ
如果我们在 DataFrame 的 set_index
方法中设置 drop=False
,即使 Name
列被设置为 index
列,它仍然是 Dataframe
中的一列。
示例代码在 Pandas DataFrame.set_index
方法中设置 inplace=True
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",inplace=True)
print("After Setting Index:")
print(df)
输出:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
如果我们在 set_index()
方法中设置 inplace=True
,调用者 DataFrame
将被原地修改。
示例代码:使用 Pandas DataFrame.set_index()
方法设置多索引列
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",append=True,inplace=True,drop=False)
print("After Setting Index:")
print(df)
输出:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Name Price In_Stock Supplier
Name
0 Orange Orange 34 Yes ABC
1 Mango Mango 24 No ABC
2 banana banana 14 No ABC
3 Apple Apple 44 Yes XYZ
如果我们在 set_index()
方法中设置 append=True
,则会将新设置的索引列追加到现有的索引中,并且单个 DataFrame
有多个索引列。
示例代码:Pandas Dataframe.set_index()
在 verify_integrity
为 True
时的行为
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('Apple', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
df_modified=df.set_index("Name", verify_integrity=True)
print(df_modified)
输出:
Traceback (most recent call last):
.....line 3920, in set_index
dup=duplicates))
ValueError: Index has duplicate keys: Index(['Apple'], dtype='object', name='Name')
因为索引有重复的索引- Apple
,所以会引发 ValueError
。它有两个 Apple
在列中被设置为索引;因此,如果 set_index()
方法中的 verify_integrity
被设置为 True
,它就会引发一个错误。
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn