Pandas DataFrame DataFrame.replace()函数
Suraj Joshi
2023年1月30日
-
pandas.DataFrame.replace()
语法 -
示例代码:使用
pandas.DataFrame.replace()
替换 DataFrame 中的值 -
示例代码:在 DataFrame 中使用
pandas.DataFrame.replace()
替换多个值
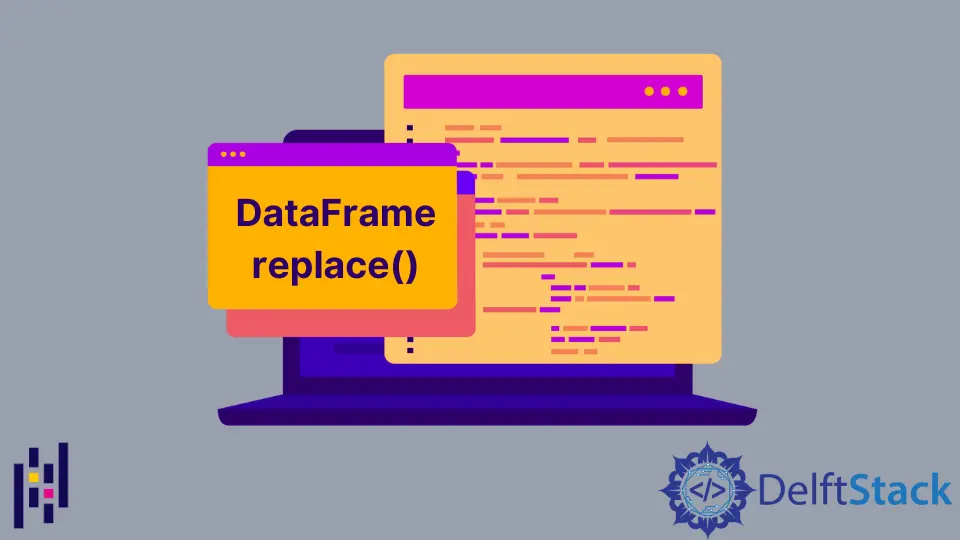
pandas.DataFrame.replace()
用其他值替换 DataFrame 中的值,这些值可以是字符串、正则表达式、列表、字典、Series
或数字。
pandas.DataFrame.replace()
语法
DataFrame.replace(,
to_replace=None,
value=None,
inplace=False,
limit=None,
regex=False,
method='pad')
参数
to_replace |
字符串,正则表达式,列表,字典,系列,数字或 None . 需要替换的 DataFrame 中的值。 |
value |
标量,字典,列表,字符串,正则表达式或 None . 替换任何与 to_replace 匹配的值。 |
inplace |
布尔值。如果为 True 修改调用者的 DataFrame |
limit |
整数。向前或向后填充的最大间隙大小 |
regex |
布尔型。如果 to_replace 和/或 value 是一个正则表达式,则将 regex 设为 True 。 |
method |
用于替换的方法 |
返回值
它返回一个 DataFrame
,用给定的 value
替换所有指定的字段。
示例代码:使用 pandas.DataFrame.replace()
替换 DataFrame 中的值
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace(1, 5)
print("After Replacement")
print(replaced_df)
输出:
Before Replacement
X Y
0 1 4
1 2 1
2 3 8
After Replacement
X Y
0 5 4
1 2 5
2 3 8
这里,1
代表 to_replace
参数,5
代表 replace()
方法中的 value
参数。因此,在 df
中,所有值为 1
的元素都被 5
取代。
示例代码:在 DataFrame 中使用 pandas.DataFrame.replace()
替换多个值
使用列表替换
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace([1,2,3],[1,4,9])
print("After Replacement")
print(replaced_df)
输出:
Before Replacement
X Y
0 1 4
1 2 1
2 3 8
After Replacement
X Y
0 1 4
1 4 1
2 9 8
这里,[1,2,3]
代表 to_replace
参数,[1,4,9]
代表 replace()
方法中的 value
参数。因此,在 df
中,列 [1,2,3]
被 [1,4,9]
替换。
使用字典替换
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [3, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace({1:10,3:30})
print("After Replacement")
print(replaced_df)
输出:
Before Replacement
X Y
0 1 3
1 2 1
2 3 8
After Replacement
X Y
0 10 30
1 2 10
2 30 8
它将所有值为 1
的元素替换为 10
,将所有值为 3
的元素替换为 30
。
使用 Regex
替换
import pandas as pd
df = pd.DataFrame({'X': ["zeppy", "amid", "amily"],
'Y': ["xar", "abc", "among"]})
print("Before Replacement")
print(df)
df.replace(to_replace=r'^ami.$', value='song', regex=True,inplace=True)
print("After Replacement")
print(df)
输出:
Before Replacement
X Y
0 zeppy xar
1 amid abc
2 amily among
After Replacement
X Y
0 zeppy xar
1 song abc
2 amily among
它将所有前三个字符为 ami
然后后面跟任何一个字符的元素替换为 song
。这里只有 amid
满足给定的正则表达式,因此只有 amid
被 song
替换。虽然 amily
的前三个字符是 ami
,但 ami
后面还有两个字符。所以,amily
不满足给定的 regex,因此它保持一样,没被替换。如果你使用的是正则表达式,请确保 regex
设置为 True
,并且 inplace=True
在调用 replace()
方法后修改原来的 DataFrame
。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn