二叉搜尋樹中序後代
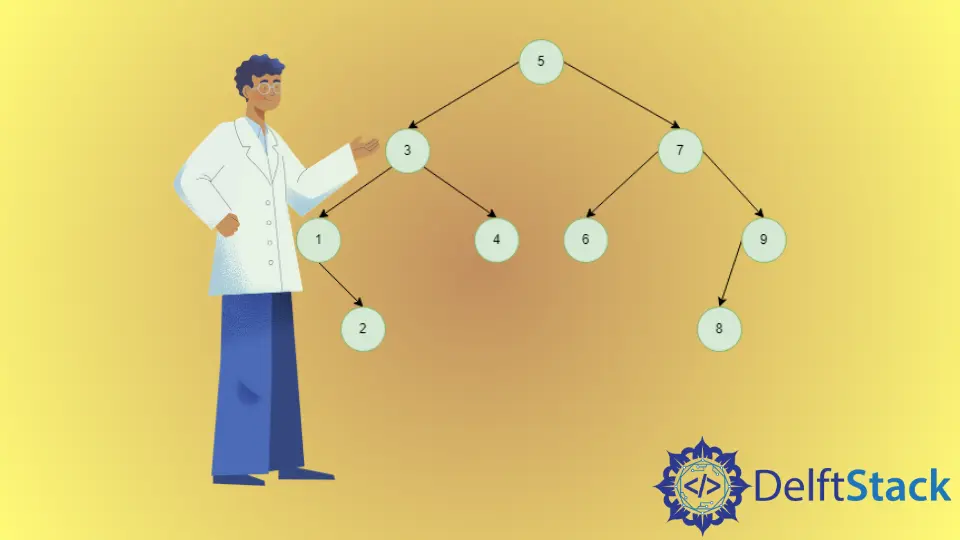
二叉樹的中序後代是二叉樹中序遍歷中下一個節點。所以,對於樹內的最後一個節點來說,它是 NULL
。由於二叉搜尋樹的中序遍歷是一個排序陣列。比給定節點大鍵最小的節點被定義為它的中序後代。在 BST 中,中序後代有兩種可能,即在節點的右側子樹或祖先中,值最小的節點。否則,該節點的中序後代不存在。
BST 演算法中的中序後代演算法
- 如果
root
=NULL
,則將succ
設為NULL
並返回。 - 如果
root->data
<current->data
,則succ
為current
,current
為current->left
。 - 如果
root->data
>current->data
,則current
為current->right
。 - 如果
root->data
==current->data
和root->right
!=NULL
,succ
=minimum(current->right)
。 - 返回
succ
。
BST 中的中序後代圖示
3
的中序後代是 4
,因為 3
有一個右結點,4
是右子樹中比 3
大的最小的結點。
4
的順序後代是 5
,因為 4
沒有右結點,我們要看它的祖先,其中 5
是比 4
大的最小的結點。
BST 中序後代的實現
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node *left, *right;
Node(int x) {
this->data = x;
this->left = this->right = NULL;
}
};
Node* insert(Node* root, int key) {
if (root == NULL) {
return new Node(key);
}
if (key < root->data) {
root->left = insert(root->left, key);
} else {
root->right = insert(root->right, key);
}
return root;
}
Node* getNextleft(Node* root) {
while (root->left) {
root = root->left;
}
return root;
}
void inorderSuccessor(Node* root, Node*& succ, int key) {
if (root == NULL) {
succ = NULL;
return;
}
if (root->data == key) {
if (root->right) {
succ = getNextleft(root->right);
}
}
else if (key < root->data) {
succ = root;
inorderSuccessor(root->left, succ, key);
} else {
inorderSuccessor(root->right, succ, key);
}
}
int main() {
int keys[] = {1, 5, 8, 2, 6, 3, 7, 4};
Node* root = NULL;
for (int key : keys) {
root = insert(root, key);
}
for (int key : keys) {
Node* prec = NULL;
inorderSuccessor(root, prec, key);
if (prec) {
cout << "Inorder successor of node " << key << " is " << prec->data;
} else {
cout << "No inorder Successor of node " << key;
}
cout << '\n';
}
return 0;
}
BST 查詢中序後代的演算法複雜度
時間複雜度
- 平均情況
在平均情況下,在 BST 中尋找中序後代的時間複雜度與二叉搜尋樹的高度相當。平均來說,一個 BST 的高度是 O(logn)
。當形成的 BST 是一個平衡的 BST 時,就會出現這種情況。因此,時間複雜度是 [Big Theta]:O(logn)
。
- 最佳情況
最好的情況是當樹是一個平衡的 BST 時。最佳情況下,刪除的時間複雜度為 O(logn)
。它與平均情況下的時間複雜度相同。
- 最壞情況
在最壞的情況下,我們可能需要從根節點到最深的葉子節點,即樹的整個高度 h
。如果樹是不平衡的,即它是傾斜的,樹的高度可能會變成 n
,因此插入和搜尋操作的最壞情況下的時間複雜性是 O(n)
。
空間複雜度
由於遞迴呼叫所需的額外空間,該演算法的空間複雜度為 O(h)
。
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn