在 TypeScript 中獲取類名
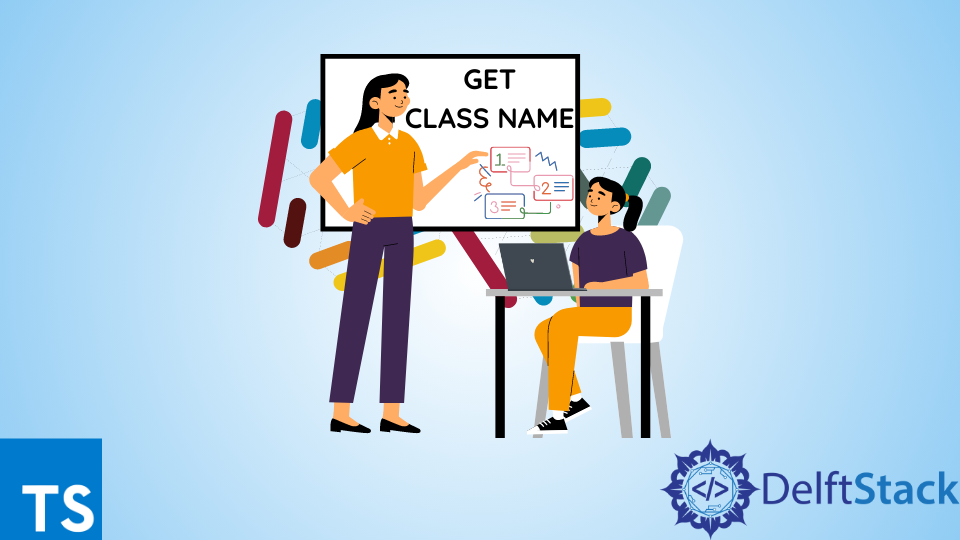
舊 JavaScript 更傾向於函數語言程式設計。隨著作為 JavaScript 超集 TypeScript 的發展,它開始支援物件導向的程式設計功能。
物件導向程式設計背後的基本術語是程式由互動的現實世界實體組成。
TypeScript 類和物件
TypeScript 支援帶有 class
概念的物件導向程式設計。類是用於建立類例項的真實世界實體的藍圖。
TypeScript 類是從 ES6 引入的。一個類由欄位、方法和建構函式組成。
class Animal {
// properties/fields
name: string;
color: string;
// constructor
constructor(name: string) {
this.name = name;
}
// methods/functions
eat(): void {
console.log("I am eating");
}
}
這些欄位被定義為儲存 Animal
物件的名稱和顏色。TypeScript 建構函式用於初始化物件的屬性。
它是一個可以引數化的特殊函式,如上例所示。
有了 Animal
類,就可以從中建立物件。TypeScript 使用 new
關鍵字從給定的類建立新物件。
由於 TypeScript 是一種強型別語言,新的 Animal
例項的型別將是 Animal
型別。
let dog: Animal = new Animal('rocky');
如你所見,建立 Animal
例項時已呼叫 Animal
類的建構函式。Animal
類建構函式接受一個字串引數,我們必須在建立物件時傳遞它。
在執行時獲取 TypeScript 物件的型別
在某些情況下,你需要在執行時知道 TypeScript 物件的型別。假設我們有一個名為 Vehicle
的類。
class Vehicle {
vehicleNo: string;
vehicleBrand: string
constructor(vehicleNo: string, vehicleBrand: string) {
this.vehicleNo = vehicleNo;
this.vehicleBrand = vehicleBrand;
}
}
讓我們建立一個新的 Vehicle
物件,miniCar
。
let miniCar: Vehicle = new Vehicle('GH-123', 'AUDI');
miniCar
是 vehicle
物件,其型別是 Vehicle
。我們可以使用該類的 name
屬性檢查特定類的名稱。
語法:
<class_name>.name
讓我們檢查類 Vehicle
的名稱。
console.log(Vehicle.name);
輸出:
Vehicle
TypeScript 物件的建構函式
如前所述,每個 TypeScript 類都包含一個建構函式,該建構函式可能是程式設計師編寫的自定義建構函式或預設建構函式。它是一種特殊型別的 TypeScript 函式。
因此,我們可以獲得對物件的 constructor
函式的引用。
語法:
Object.constructor
讓我們獲取對 miniCar
物件的建構函式的引用,如下所示。
let miniCarConstructorRef: any = miniCar.constructor;
接下來,我們將把 miniCarConstructorRef
變數值記錄到控制檯。
[Function: Vehicle]
正如預期的那樣,它引用了 miniCar
物件的建構函式。
TypeScript 允許你使用 name
屬性獲取物件的建構函式名稱。
語法:
Object.constructor.name
讓我們使用上面的語法來獲取 miniCar
物件的類名。
let classNameOfTheObject = miniCarConstructorRef.name;
最後,我們將把 classNameOfTheObject
變數值記錄到控制檯。
console.log(classNameOfTheObject);
輸出:
Vehicle
這是檢查物件所屬類的推薦方法。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.