TypeScript 中的 React 事件型別
- 在 TypeScript 中為 React 事件新增型別
-
在 TypeScript 中對事件使用 React
SyntheticEvent
型別 - 在 TypeScript 中處理鍵盤事件
- 在 TypeScript 中處理滑鼠事件
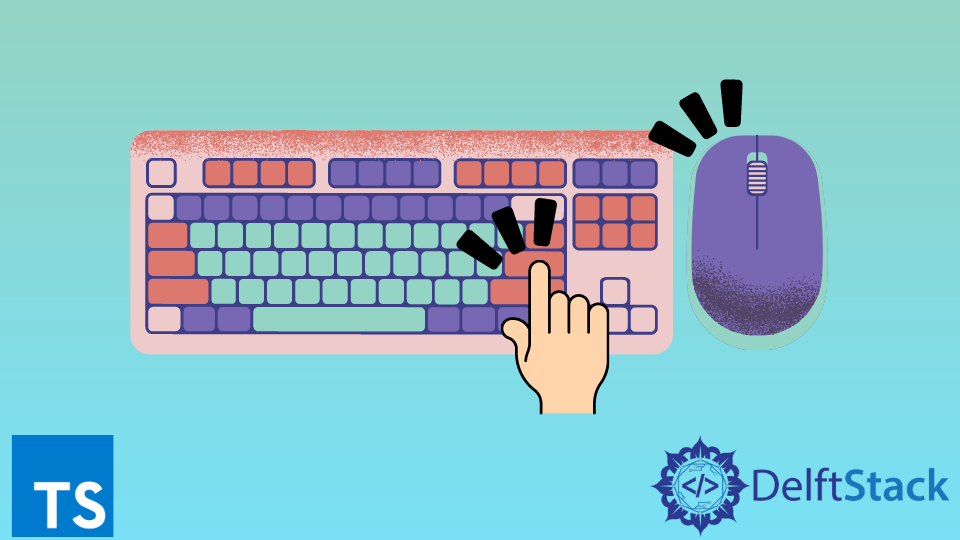
在 React 中,經常需要監聽由於對某些 HTML 元素的某些操作而觸發的事件監聽器。TypeScript 對由於 HTML 元素上的某些操作(例如觸控、單擊、聚焦和其他操作)而觸發的所有事件具有強大的鍵入支援。
本文將演示如何在 React 中為不同的事件新增型別。
在 TypeScript 中為 React 事件新增型別
React 對由 DOM 上的操作觸發的各種 HTML 事件有其型別定義。事件是由於某些操作而觸發的,例如單擊或更改某些輸入元素。
事件觸發器的一個示例是標準 HTML 文字框。
<input value={value} onChange={handleValueChange} />
上面顯示了 jsx
中的一個示例,其中 handleValueChange
接收一個 event
物件,該物件引用由於文字輸入框中輸入的更改而觸發的事件。
考慮 React 程式碼段的示例,必須為傳遞給 handleValueChange
函式的事件新增適當的型別。
const InputComponent = () => {
const [ value, setValue ] = React.useState<string>("");
const handleValueChange : React.ChangeEventHandler<HTMLInputElement> = (event) => {
setValue(event.target.value);
}
return (
<input value={value} onChange={handleValueChange}/>
)
}
因此型別 ChangeEventHandler<HTMLInputElement>
是輸入文字框中文字更改事件的型別。它可以從 React 中匯入,例如 import {ChangeEventHandler} from 'react'
。
這種型別也可以用 React.FormEvent<HTMLInputElement>
型別表示。React 事件的一些有用的型別別名可以是:
type InputChangeEventHandler = React.ChangeEventHandler<HTMLInputElement>
type TextAreaChangeEventHandler = React.ChangeEventHandler<HTMLTextAreaElement>
type SelectChangeEventHandler = React.ChangeEventHandler<HTMLSelectElement>
// can be used as
const handleOptions : SelectChangeEventHandler = (event) => {
}
在 TypeScript 中對事件使用 React SyntheticEvent
型別
React SyntheticEvent
型別充當所有事件型別的包裝器,如果不需要強型別安全性,可以使用它。可以根據需要對某些輸入使用型別斷言。
const FormElement = () => {
return (
<form
onSubmit={(e: React.SyntheticEvent) => {
e.preventDefault();
// type assertions done on the target
const target = e.target as typeof e.target & {
email: { value: string };
password: { value: string };
};
const email = target.email.value;
const password = target.password.value;
}}
>
<div>
<label>
Email:
<input type="email" name="email" />
</label>
</div>
<div>
<label>
Password:
<input type="password" name="password" />
</label>
</div>
<div>
<input type="submit" value="Sign in" />
</div>
</form>
)
}
在 TypeScript 中處理鍵盤事件
當在鍵盤上按下一個鍵時觸發鍵盤事件。React 對有關鍵盤事件的型別有很好的支援。
const handleKeyBoardPress = (event : React.KeyboardEvent<Element>) => {
if (event.key === 'Enter'){
// do something on press of enter key
}
}
它也可以表示為事件處理程式。
const handleKeyBoardPress : KeyboardEventHandler<Element> = (event) => {
if (event.key === 'Enter'){
// do something on press of enter key
}
}
Element
是封裝了以下函式 handleKeyBoardPress
的元件。
在 TypeScript 中處理滑鼠事件
滑鼠事件也可以通過在 TypeScript 中新增型別來支援。需要型別斷言來訪問與觸發滑鼠事件的 HTML 元素關聯的方法。
const handleOnClick : React.MouseEventHandler<HTMLInputElement> = (event) => {
const HTMLButton = e.target as HTMLElement;
}