用 Python 向檔案寫入位元組
Manav Narula
2023年1月30日
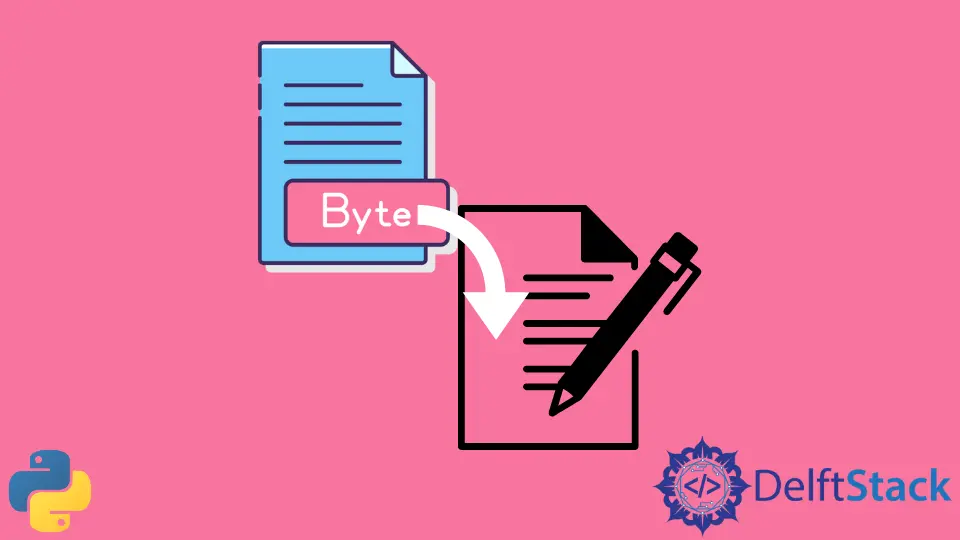
在本教程中,我們將介紹在 Python 中如何向二進位制檔案寫入位元組。
二進位制檔案包含型別為位元組的字串。當我們讀取二進位制檔案時,會返回一個位元組型別的物件。在 Python 中,位元組用十六進位制數字表示。它們的字首是 b
字元,表示它們是位元組。
在 Python 中向檔案寫入位元組
為了向檔案寫入位元組,我們將首先使用 open()
函式建立一個檔案物件,並提供檔案的路徑。檔案應該以 wb
模式開啟,它指定了二進位制檔案的寫入模式。下面的程式碼顯示了我們如何向檔案寫入位元組。
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.write(data)
當我們需要在現有檔案的末尾新增更多的資料時,我們也可以使用追加模式 - a
。比如說
data = b"\xC3\xA9"
with open("test.bin", "ab") as f:
f.write(data)
要在特定位置寫入位元組,我們可以使用 seek()
函式,指定檔案指標的位置來讀寫資料。例如:
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.seek(2)
f.write(data)
在 Python 中向檔案寫入位元組陣列
我們可以使用 bytearray()
函式建立一個位元組陣列。它返回一個可變的 bytearray
物件。我們也可以將其轉換為位元組來使其不可變。在下面的例子中,我們將一個位元組陣列寫入一個檔案。
arr = bytearray([1, 2, 5])
b_arr = bytes(arr)
with open("test.bin", "wb") as f:
f.write(b_arr)
將 BytesIO
物件寫入二進位制檔案中
io
模組允許我們擴充套件與檔案處理有關的輸入輸出函式和類。它用於在記憶體緩衝區中分塊儲存位元組和資料,並允許我們處理 Unicode 資料。這裡使用 BytesIO
類的 getbuffer()
方法來獲取物件的讀寫檢視。請看下面的程式碼。
from io import BytesIO
bytesio_o = BytesIO(b"\xC3\xA9")
with open("test.bin", "wb") as f:
f.write(bytesio_o.getbuffer())
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn