在 Python 中檢查字串是否為數字
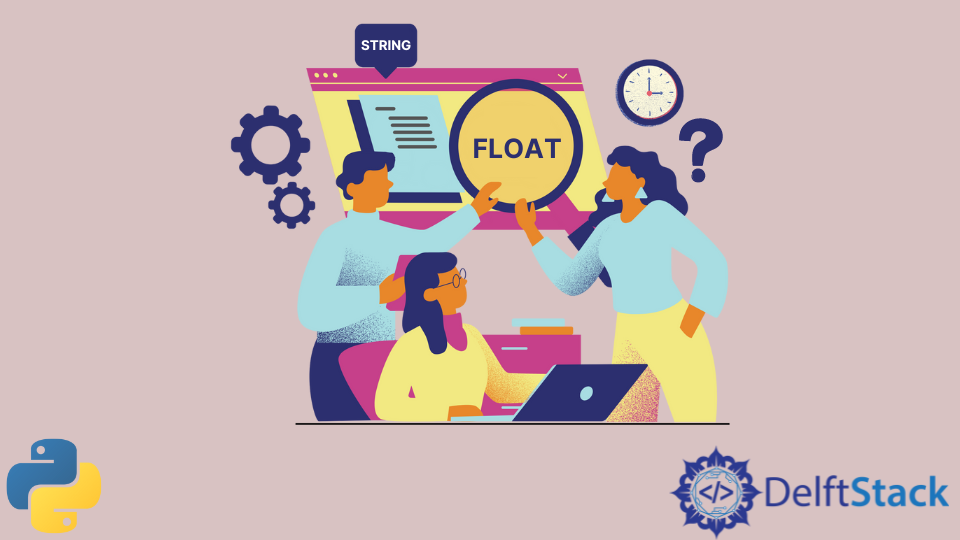
字串是由雙引號或單引號括起來的字元序列。跨越多行的字串,也稱為多行字串,用三引號括起來。
另一方面,浮點數是 Python 最常見的內建數字資料型別之一。float
或浮點數可以描述為帶小數點的數字。
print(type(3.445))
輸出:
<class 'float'>
在 Python 中使用 float()
函式檢查字串是否為數字
Python 提供了預定義的型別轉換函式,可以將一種資料型別轉換為另一種資料型別。這些函式包括分別用於將資料型別轉換為整數、字串和浮點資料型別的 int()
、str()
和 float()
函式。
除了通過插入小數點建立浮點數外,還可以通過將字串隱式或顯式轉換為浮點數來建立浮點數。
僅當值相容時,隱式轉換由 Python 自動完成,無需使用者參與。使用 float()
函式可以將字串資料型別顯式轉換為浮點數,如下面的程式碼所示。
count = "22.23"
print(type(count))
r = float(count)
print(type(r))
輸出:
<class 'str'>
<class 'float'>
型別轉換是 Python 和一般程式設計中的一個重要概念。當需要在程式中使用特定資料型別時,這一點尤為明顯;在這種情況下,型別轉換可以將任何資料轉換為所需的資料型別。
在執行檔案操作(例如將檔案讀取和寫入各種程式)時,經常會遇到此類情況。
雖然在上面的程式碼片段中,我們毫不費力地使用了單引數函式 float()
將字串資料型別轉換為浮點數字資料型別,但這種方法並非沒有限制。這些限制包括 ValueError
,它通常用於將具有字元序列的字串轉換為浮動資料型別。
如果傳遞了超出函式範圍的數字,則使用 float 函式可能會導致 OverFlow
錯誤。下面的程式碼片段說明了當 float 函式轉換無效字串時如何產生 ValueError
。
name = "developer"
rslt = float(name)
print(rslt)
輸出:
Traceback (most recent call last):
File "<string>", line 2, in <module>
ValueError: could not convert string to float: 'developer'
ValueError
可以通過確保考慮進行轉換的字串看起來像一個浮點數來避免。
其他例項也可能導致 ValueError
,其中包括空格、逗號或任何其他非特殊字元。因此,無論何時處理檔案,都需要事先檢查字串值是否有效以及是否可以轉換為浮點數。
但是,如果值太多,嘗試檢查每個值是低效的。或者,我們可以建立一個函式來檢查字串是否是可以轉換為浮點數的有效浮點數。
如果字串無效,程式應該引發異常而不是丟擲錯誤。
def is_valid_float(element: str) -> bool:
try:
float(element)
return True
except ValueError:
return False
上面的函式接受一個字串作為引數,並檢查它是否是可以轉換為浮點數的有效字串。如果字串不是可以轉換為浮點數的有效字串,則該函式返回 false,否則返回 true。
在 Python 中使用 isdigit()
和 partition()
函式檢查字串是否為數字
或者,我們可以使用 isdigit()
函式而不是 float()
函式。如果字串僅包含數字,則 isdigit()
函式返回 true,如果至少有一個字元不是數字,則返回 false。
但是,如果字串包含浮點數,即使浮點數是有效字串,此函式也會返回 false。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546.4646")
輸出:
String is not valid
我們將使用 partition
函式來確保 isdigit()
函式不會返回 false 語句,即使字串包含浮點數。
這個函式允許我們將包含浮點數的字串分成幾部分來檢查它們是否是數字。如果兩個部分都是數字,該函式會讓我們知道這是一個有效的字串。
def check_float(element):
partition = element.partition('.')
if element.isdigit():
newelement = float(element)
print('string is valid')
elif (partition[0].isdigit() and partition[1] == '.' and partition[2].isdigit()) or (partition[0] == '' and partition[1] == '.' and partition[2].isdigit()) or (partition[0].isdigit() and partition[1] == '.' and partition[2] == ''):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float('234.34')
輸出:
234.34
String is also valid !
請注意,如果字串包含不是浮點數的數字,則該函式不需要對字串進行分割槽。
def check_float(element):
partition = element.partition('.')
if element.isdigit():
newelement = float(element)
print('string is valid')
elif (partition[0].isdigit() and partition[1] == '.' and partition[2].isdigit()) or (partition[0] == '' and partition[1] == '.' and partition[2].isdigit()) or (partition[0].isdigit() and partition[1] == '.' and partition[2] == ''):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float('234')
輸出:
string is valid
另一方面,如果字串包含一個浮點數,其中包含一個不能被視為數字的字元,那麼該浮點數將不是一個有效的字串。在這種情況下,有效字串是指可以使用 float()
函式轉換為浮點數的字串。
def check_float(element):
partition = element.partition('.')
if element.isdigit():
newelement = float(element)
print('string is valid')
elif (partition[0].isdigit() and partition[1] == '.' and partition[2].isdigit()) or (partition[0] == '' and partition[1] == '.' and partition[2].isdigit()) or (partition[0].isdigit() and partition[1] == '.' and partition[2] == ''):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float('234.rt9')
輸出:
string is not valid !
對於以指數形式表示的整數和數字以及任何數字的 Unicode 字元,isdigit()
函式也返回 true。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546")
輸出:
String is valid
如果字串只包含上面示例程式碼塊中的數字,我們將 isdigit()
方法與一個函式一起使用,該函式返回一個肯定的訊息。但是,當使用不包含數字的引數呼叫該函式時,該函式會列印一條否定訊息,如下面的程式碼所示。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("Pal Alto")
輸出:
String is not valid
因此,使用 isdigit()
函式,我們可以輕鬆確定字串是否有效以及是否可以轉換為浮點數。包含空格和符號的字串不能轉換為浮點數。
使用 isdigit()
方法,我們還可以確定包含空格的字串是否有效,如下面的程式碼所示。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("$4546.343")
輸出:
String is not valid
包含任何空格的字串同樣不是有效字串,不能轉換為浮點數。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("45 8")
輸出:
String is not valid
複數在 Python 中的三種內建數字資料型別中同等重要。我們還可以在 complex()
函式旁邊使用 float()
函式來確定包含複數的字串是否也可以轉換為浮點資料型別。
complex()
函式將整數和字串轉換為複數。因此使用這兩個函式,我們可以確認包含字元序列的字串不能轉換為浮點數,也不能轉換為複數。
這些函式還可以檢查包含複數的字串是否可以轉換為浮點數,如下面的程式碼所示。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string('hello'))
輸出:
False
相反,由整數或浮點陣列成的字串可以轉換為浮點數和複數。因此,如果我們傳遞具有上述描述的引數,該函式可能會返回 True
。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string('456.45'))
輸出:
True
由整陣列成的字串同樣有效,可以轉換為浮點數和複數。因此,我們也會得到一個 True 語句。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string('456'))
輸出:
True
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn相關文章 - Python String
- 在 Python 中從字串中刪除逗號
- 如何用 Pythonic 的方式來檢查字串是否為空
- 在 Python 中將字串轉換為變數名
- Python 如何去掉字串中的空格/空白符
- 如何在 Python 中從字串中提取數字
- Python 如何將字串轉換為時間日期 datetime 格式