如何在 Python 中替換字串中的多個字元
-
使用
str.replace()
替換 Python 中的多個字元 -
使用
re.sub()
或re.subn()
來替換 Python 中的多個字元 -
在 Python 中使用
translate()
和maketrans()
替換多個字元
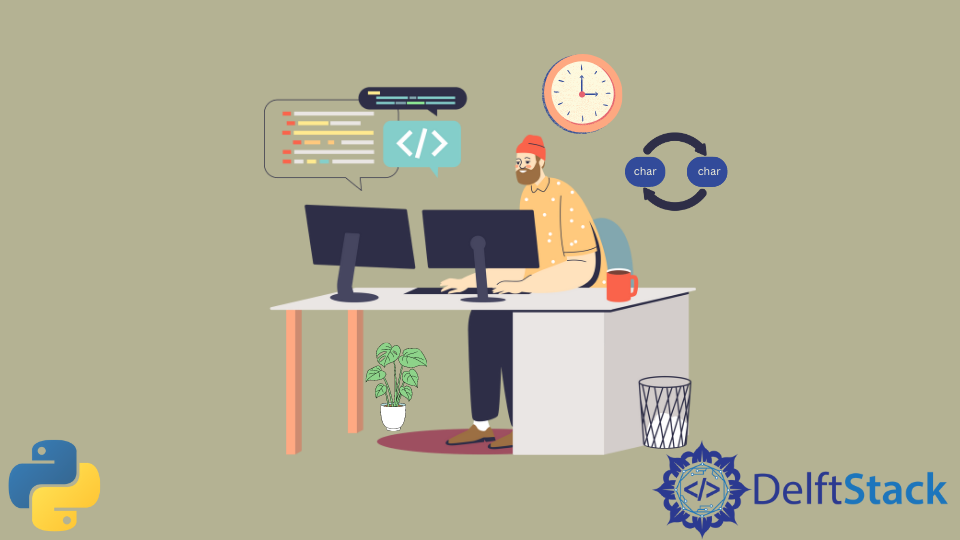
本教程向你展示瞭如何在 Python 中替換一個字串中的多個字元。
假設我們想刪除字串中的特殊字元,並用空格代替。
- 要刪除的特殊字元列表是
!#$%^&*()
。 - 另外,我們還想用 whitespace 替換逗號
,
。 - 我們將操作的示例文字。
A!!!,Quick,brown#$,fox,ju%m%^ped,ov&er&),th(e*,lazy,d#!og$$$
使用 str.replace()
替換 Python 中的多個字元
我們可以使用 str
的 replace()
方法將子字串替換成不同的輸出。
replace()
接受兩個引數,第一個引數是你要匹配字串的 regex 模式,第二個引數是匹配字串的替換字串。
它在 replace()
中還有第三個可選引數,它接受一個整數,用來設定要執行的最大 count
替換次數。如果你把 2
作為 count
引數,replace()
函式將只匹配和替換字串中的 2 個例項。
str.replace('Hello', 'Hi')
將用 Hi
替換字串中所有 Hello
的例項。如果你有一個字串 Hello World
,並對其執行 replace 函式,執行後會變成 Hi World
。
讓我們對上面宣告的示例文字使用 replace
。首先將特殊字元去掉,通過迴圈每個字元並替換成一個空字串,然後將逗號轉換為空格。
txt = "A!!!,Quick,brown#$,fox,ju%m%^ped,ov&er&),th(e*,lazy,d#!og$$$"
def processString(txt):
specialChars = "!#$%^&*()"
for specialChar in specialChars:
txt = txt.replace(specialChar, '')
print(txt) # A,Quick,brown,fox,jumped,over,the,lazy,dog
txt = txt.replace(',', ' ')
print(txt) # A Quick brown fox jumped over the lazy dog
這意味著在 spChars
方括號內的任何字元都將被一個空字串替換,使用 txt.replace(spChars, '')
。
第一個 replace()
函式的字串結果將是:
A,Quick,brown,fox,jumped,over,the,lazy,dog
下一個 replace()
呼叫將把所有逗號 ,
的例項替換成單個空格。
A Quick brown fox jumped over the lazy dog
使用 re.sub()
或 re.subn()
來替換 Python 中的多個字元
在 Python 中,你可以匯入 re
的模組,它有大量的 regex 的表示式匹配操作供你利用。
re
中的兩個這樣的函式是 sub()
和 subn()
。
我們再為這些方法宣告一個字串的例子。假設我們想把一個字串內的所有數字替換成 X。
txt = "Hi, my phone number is 089992654231. I am 34 years old. I live in 221B Baker Street. I have 1,000,000 in my bank account."
在 Python 中使用 re.sub()
替換多個字元
該函式有 3 個主要引數。第一個引數接受一個 regex 模式,第二個引數是一個字串,用來替換匹配的模式,第三個引數是要操作的字串。
建立一個函式,將一個字串中的所有數字轉換為 X。
import re
txt = "Hi, my phone number is 089992654231. I am 34 years old. I live in 221B Baker Street. I have 1,000,000 in my bank account."
def processString3(txt):
txt = re.sub('[0-9]', 'X', txt)
print(txt)
processString3(txt)
輸出:
Hi, my phone number is XXXXXXXXXXXX. I am XX years old. I live in XXXB Baker Street. I have X,XXX,XXX in my bank account.
re.subn()
替換 Python 中的多個字元
這個函式本質上與 re.sub()
相同,但是返回一個轉換後的字串的元組和替換的數量。
import re
txt = "Hi, my phone number is 089992654231. I am 34 years old. I live in 221B Baker Street. I have 1,000,000 in my bank account."
def processString4(txt):
txt, n = re.subn('[0-9]', 'X', txt)
print(txt)
processString4(txt)
輸出:
Hi, my phone number is XXXXXXXXXXXX. I am XX years old. I live in XXXB Baker Street. I have X,XXX,XXX in my bank account.'
txt, n = re.subn('[0-9]', 'X', txt)
在上面的程式碼片段中,處理後的字串被分配給 txt
,替換計數器被分配給 n
。
如果你想記下有多少個模式組作為指標或用於進一步處理,則 re.subn()
很有用。
在 Python 中使用 translate()
和 maketrans()
替換多個字元
translate()
和 maketrans()
使用一種不同於 regex 的方法,它利用字典將舊值對映到新值。
maketrans()
接受 3 個引數或一個字典的對映。
str1
- 要替換的字串。str2
- 以上字元的替換字串。str3
- 要刪除的字串。
maketrans()
一個原始字串和它的替換之間的對映表。
translate()
接受任何 maketrans()
返回,然後生成翻譯後的字串。
比方說,我們想把一個字串中的所有小寫母音轉換成大寫和刪除字串中的所有 x、y 和 z。
txt = "Hi, my name is Mary. I like zebras and xylophones."
def processString5(txt):
transTable = txt.maketrans("aeiou", "AEIOU", "xyz")
txt = txt.translate(transTable)
print(txt)
processString5(txt)
輸出:
HI, m nAmE Is MAr. I lIkE EbrAs And lOphOnEs.
translate()
將所有小寫母音轉換為大寫,並刪除所有 x, y, 和 z 的例項。
使用這些方法的另一種方法是使用一個單一的對映字典而不是三個引數。
def processString6(txt):
dictionary = {'a': 'A', 'e':'E', 'i': 'I', 'o': 'O', 'u': 'U', 'x': None, 'y': None, 'z': None}
transTable = txt.maketrans(dictionary)
txt = txt.translate(transTable)
print(txt)
這仍然會產生與 processString5
相同的輸出,但是是用字典實現的。你可以使用任何對你更方便的方法。
總之,有多種方法可以通過使用 Python 中的內建函式或從匯入庫中的函式來替換字串中的多個字元。
最常用的方法是使用 replace()
。re.sub()
和 subn()
也相當容易使用和學習。translate()
使用不同的方法,因為它不依靠正規表示式來執行字串操作,而是依靠字典和 Map。
如果你願意,你甚至可以使用 for 迴圈在字串上手動迴圈,並新增你自己的條件來替換,只需使用 substring()
或 split()
,但這將是非常低效和多餘的。Python 提供了現有的函式來為你完成這項工作,這比你自己完成繁瑣的工作要容易得多。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn