Python 中的私有方法
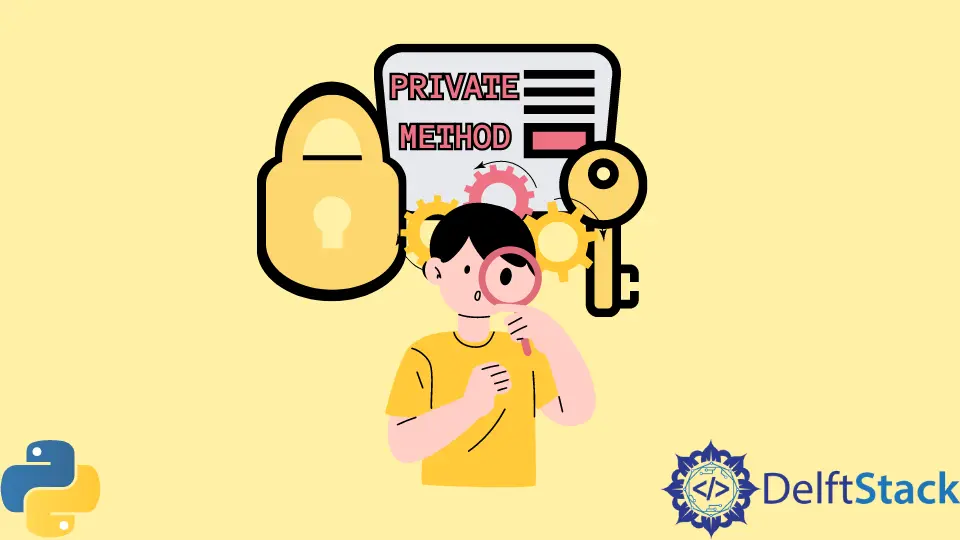
本教程演示瞭如何在 Python 中宣告、操作和使用私有方法。
private
是物件導向程式語言中使用的一種訪問修飾符的關鍵字。訪問修飾符在一定程度上限制了函式或變數的可見性。將你的函式/變數宣告為私有將訪問許可權僅限於封裝它的類。
與私人方法的現實比較將是家庭照明系統。電燈開關和燈泡就像公共方法,因為人們可以直接訪問和檢視它們。同時,保護橡膠內的電線是私人方法,因為它們通常不可見,除非被篡改,但它們仍然在大多數情況下沒有人注意的情況下工作。
Python 中的私有訪問修飾符
在 Python 中,私有方法是不能在宣告它的類之外訪問的方法,也不能被任何其他基類訪問。
要在 Python 中宣告私有方法,請在方法名稱的開頭插入雙下劃線。
__init__()
方法
Python 中一個值得注意的私有方法是 __init__()
方法,它用作類物件的類建構函式。當類的物件被例項化時,根據方法的引數呼叫此方法。
例如,宣告一個具有兩個欄位和一個 __init__()
方法的 Person
類:
class Person:
name = ""
age = 0
def __init__(self, n, a):
self.name = n
self.age = a
現在,要訪問類外的私有 __init__()
方法,我們需要它在例項化後從類本身的物件中訪問它。
例如,在同一目錄中的另一個檔案中,建立 Person
類的例項並使用類名呼叫建構函式。
from personClass import Person
sys.path.append(".")
person = Person("John Doe", 25)
print(person.name, person.age)
輸出:
John Doe 25
sys.path.append()
和要匯入的類的字串路徑目錄作為引數。在這種情況下,兩個檔案都駐留在同一個資料夾中,因此句點 .
足夠了。然後,從 .py
檔案 (personClass.py
) 匯入類 (Person
)。在將 Person
類例項化為變數以重新例項化物件後,可以顯式呼叫 __init__()
方法。
例如:
person = Person("John Doe", 25)
person.__init__("Jane Doe", 29)
print(person.name, person.age)
輸出:
Jane Doe 29
此外,__init()__
方法可以通過從類本身呼叫它來顯式呼叫。儘管對於這種方法,你需要明確地將第一個引數 self
放入引數中。
person = Person("John Doe", 25)
Person.__init__(person, "Jack Sparrow", 46) # person is the 'self' argument
print(person.name, person.age)
輸出:
Jack Sparrow 46
所有這些方法都保留了 __init__()
方法的私有屬性。
現在已經剖析了這個內建方法。讓我們繼續將我們自己的私有方法實際實現到一個類中,並將其訪問與公共方法區分開來。
在 Python 中宣告一個私有方法
要宣告私有方法,請在相關方法前加上雙下劃線 __
。否則,它將被視為預設公共方法。
讓我們從前面的例子中擴充套件 Person
類,並建立一個子類 Employee
,它的建構函式基於 Person
類。
此外,在 person 類中建立兩個新方法,一個公共方法和一個私有方法。
class Person:
name = ""
age = 0
def __init__(self, name, age):
self.name = name
self.age = age
def walk(self):
print("Walking")
def __call(self):
print("Taking a call")
現在,建立擴充套件 Person
的派生類或子類 Employee
:
class Employee(Person):
occupation = "Unemployed"
salary = 0
def __init__(self, name, age, occupation, salary):
Person.__init__(self, name, age)
self.occupation = occupation
self.salary = salary
def work(self):
print("Employee is working")
self.walk()
def takeCall(self):
self.__call()
print("Employee is taking a call")
Person
類。在這個類中,work()
和 takeCall()
方法分別從父類 Person
外部呼叫 walk()
和 __call()
類。
另一個方法從外部呼叫公共方法,另一個方法從其父類呼叫私有方法。讓我們看看當我們執行它時這個行為是如何工作的。
例如,給定上面的類宣告:
employee_1 = Employee("John Doe", 25, "Software Engineer", 40000)
employee_1.work()
employee_1.takeCall()
輸出:
Employee is working
Walking
Traceback (most recent call last):
File "python/demo.py", line 35, in <module>
employee_1.takeCall()
File "python/demo.py", line 29, in takeCall
self.__call()
AttributeError: 'Employee' object has no attribute '_Employee__call'
對 work()
方法的呼叫成功執行,列印出來自 work()
和 walk()
方法的語句。但是,對 takeCall()
的呼叫會觸發 AttributeError
,因為它無法將 Person
類的 __call()
方法識別為 Employee
類的方法。將一個類擴充套件到另一個類不會在擴充套件中包含它自己的私有方法。
總之,Python 中的私有方法是通過在方法前加上兩個下劃線 __
來宣告的。宣告私有方法允許為封裝類專門保留一個方法。使用私有方法擴充套件另一個類的類不會繼承這些方法,並在嘗試訪問它時觸發錯誤。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn