在 Python 中解析 YAML 檔案
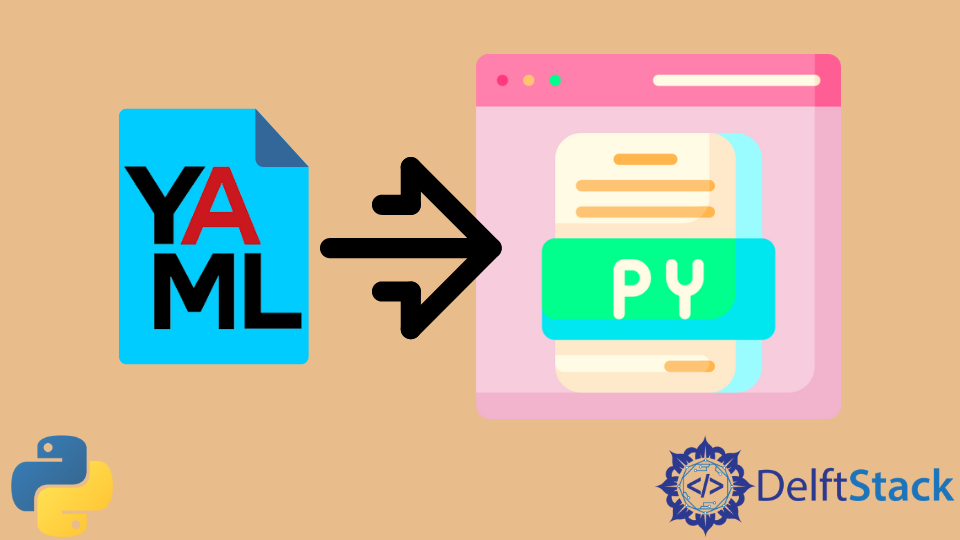
本教程將演示在 Python 中解析 YAML 檔案的不同方法。
YAML 是一種資料序列化標準,用作檔案型別以在專案中儲存配置檔案或屬性檔案。YAML 通常替換典型的 XML 或 JSON 配置檔案,因為與這兩種檔案型別相比,檔案更易於序列化。
在 Python 中使用 PyYAML
包解析 YAML
pyyaml
是一個 Python 軟體包,提供了實用程式來解析和處理 Python 中的 YAML 檔案。
第一步是使用 pip
安裝 pyyaml
,因為它不是現成的 Python 包。
pip install pyyaml
對於 Python 3.x 使用者,請使用 pip3
而不是 pip
。
pip3 install pyyaml
現在安裝完成,繼續解析示例 YAML 檔案。YAML 檔案的開頭始終始終帶有三個破折號 ---
,以表示新的 YAML 文件的開頭。
請注意,縮排 YAML 檔案時應僅使用空格,因為這些檔案不會將製表符空格識別為縮排。
YAML 檔案的副檔名可以是 .yaml
或 .yml
。
sample.yaml
---
name: "John"
age: 23
isMale: true
hobbies:
- swimming
- fishing
- diving
要在 Python 中讀取給定的 YAML 檔案,請首先匯入 yaml
模組。然後,使用 open()
函式開啟 yaml 檔案。yaml
模組具有預定義的函式 load()
,該函式接受檔案作為引數,在 Python 中載入 YAML 檔案,並將其轉換為 Python 物件資料型別。
import yaml
with open('sample.yaml', 'r') as f:
print(yaml.load(f))
如果你列印 yaml.load()
的輸出,它將以 Python 物件格式顯示 YAML 檔案的內容。
輸出:
{'name': 'John', 'age': 23, 'isMale': True, 'hobbies': ['swimming', 'fishing', 'diving']}
為了確保 YAML 檔案存在並且沒有損壞,可以安全使用,我們可以使用 yaml
模組 YAMLError
中的內建錯誤物件新增 try
塊並捕獲異常。使程式碼更安全使用的另一層是用 safe_load()
替換 load()
函式,該函式在載入之前先驗證 YAML 檔案。
import yaml
with open("sample.yaml", 'r') as stream:
try:
print(yaml.safe_load(stream))
except yaml.YAMLError as exc:
print(exc)
此解決方案與以前的解決方案具有相同的輸出,儘管這是在 Python 中解析 YAML 檔案的更安全的方法。
總而言之,使用 yaml
模組可以很容易地在 Python 中解析 YAML 檔案。使用模組之前,請確保先執行 pip install pyyaml
,因為它不是現成的內建模組。
用 try...except
塊封裝檔案操作函式是一個很好的做法,以確保檔案操作的順利進行和安全執行。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn