如何在 Python 中查詢列表中的最大值
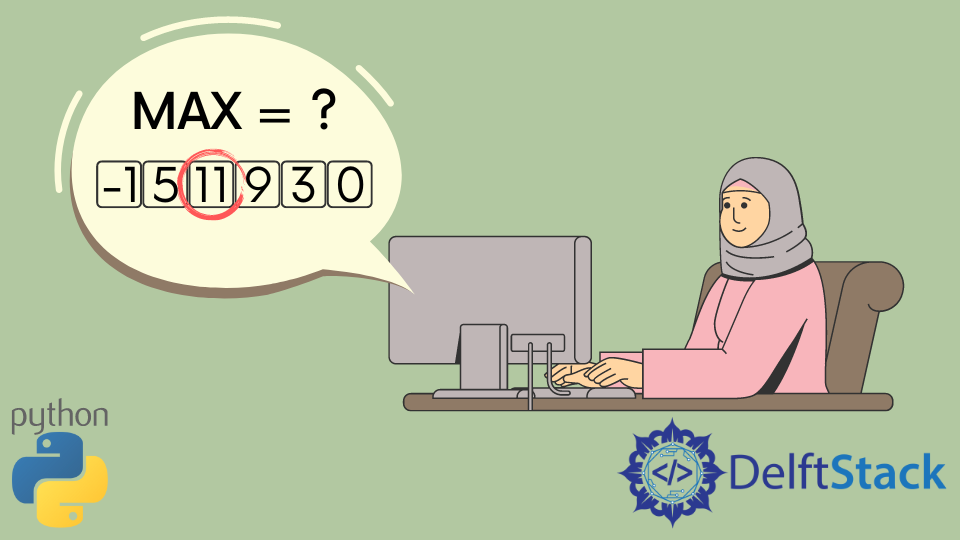
本教程將演示如何在 Python 中查詢列表中的最大值。
本教程將涵蓋一些場景和資料型別,從簡單的整數列表到更復雜的結構,如陣列中的陣列。
在 Python 中使用 for
迴圈查詢列表中的最大值
Python for
迴圈可以通過比較陣列中的每個值並將最大的值儲存在一個變數中,從而找到列表中的最大值。
例如,讓我們宣告一個隨機整數的陣列,並列印出最大值。同時,宣告一個變數 max_value
來儲存最大值,並在迴圈結束後將其列印出來。
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = None
for num in numbers:
if (max_value is None or num > max_value):
max_value = num
print('Maximum value:', max_value)
輸出:
Maximum value: 104
如果需要用最大值的索引來進一步操作輸出,只需使用 Python 函式 enumerate
,它可以使 for
迴圈接受第二個引數,表示當前迴圈迭代的索引。
新增一個新變數 max_idx
來儲存最大值的最大索引。
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = None
max_idx = None
for idx, num in enumerate(numbers):
if (max_value is None or num > max_value):
max_value = num
max_idx = idx
print('Maximum value:', max_value, "At index: ", max_idx)
輸出:
Maximum value: 104 At index: 4
在 Python 中使用 max()
函式查詢列表中的最大值
Python 有一個預先定義的函式,叫做 max()
,它可以返回一個列表中的最大值。
使用 max()
尋找最大值只需要一行程式碼。
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = max(numbers)
print('Maximum value:', max_value)
輸出:
Maximum value: 104
如果你需要最大值的索引,我們可以呼叫 Python 列表中內建的 index()
函式。該函式將返回陣列中指定元素的索引。
numbers = [55, 4, 92, 1, 104, 64, 73, 99, 20]
max_value = max(numbers)
print('Maximum value:', max_value, "At index:", arr.index(max_value))
輸出:
Maximum value: 104 At index: 4
使用 max()
在字串和字典列表中查詢最大值
函式 max()
還提供對字串列表和字典資料型別的支援。
對於一個字串列表,函式 max()
將返回最大的元素,按字母排序。字母 Z 是最大的值,A 是最小的值。
strings = ['Elephant', 'Kiwi', 'Gorilla', 'Jaguar', 'Kangaroo', 'Cat']
max_value = max(strings)
print('Maximum value:', max_value, "At index:", strings.index(max_value))
輸出:
Maximum value: Kiwi At index: 1
在 Python 中查詢巢狀列表中的最大值
一個更復雜的例子是在 Python 中尋找巢狀列表的最大值。
首先,我們在一個巢狀列表中宣告隨機值。
nst = [ [1001, 0.0009], [1005, 0.07682], [1201, 0.57894], [1677, 0.0977] ]
從這個巢狀列表中,我們想得到巢狀列表中第 2 個元素的最大值。我們還想把第 1 個元素列印出來,並把它當作一個索引。巢狀列表的處理方式更像是一個列表中的鍵值對。
為了找到這個複雜結構的最大值,我們可以使用函式 max()
及其可選的引數 key
,它記下了巢狀列表中要比較的元素。
nst = [ [1001, 0.0009], [1005, 0.07682], [1201, 0.57894], [1677, 0.0977] ]
idx, max_value= max(nst, key=lambda item: item[1])
print('Maximum value:', max_value, "At index:",idx)
key
引數接受一個 lambda 函式,將允許函式 max()
返回一個鍵值對。
輸出:
Maximum value: 0.57894 At index: 1201
總而言之,本教程涵蓋了在 Python 中尋找不同型別列表中的最大值的不同方法。你可以在這裡閱讀更多關於函式 max()
及其不同引數的資訊。除了 max()
之外,還有一些內建的函式,如 min()
、all()
和 any()
,可以在 Python 中操作可迭代物件。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn