在 Python 中使用尤拉數
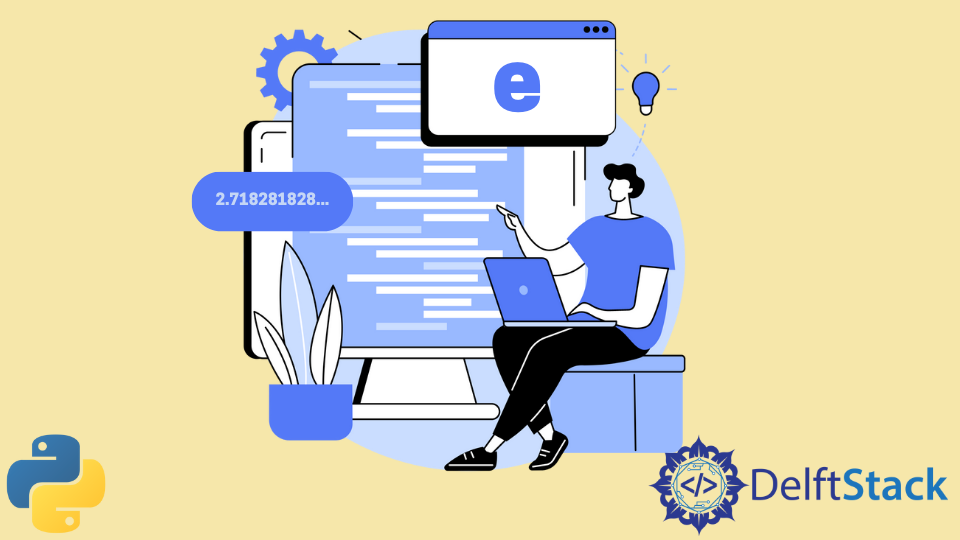
尤拉數或 e
是數學中最基本的常數之一,就像 pi
一樣。e
是自然對數函式的基礎。它是代表指數常數的無理數。
本教程將演示如何在 Python 中複製尤拉數(e
)。
有三種常見的方法來獲取尤拉數並將其用於 Python 中的方程式。
- 使用
math.e
- 使用
math.exp()
- 使用
numpy.exp()
在 Python 中使用 math.e
來獲取尤拉數
Python 模組 math
包含許多可用於方程式的數學常數。尤拉數或 e
是 math
模組具有的常數之一。
from math import e
print(e)
輸出:
2.718281828459045
上面的輸出是 e
常數的基值。
作為一個示例方程式,讓我們建立一個函式,以將 e^n
或 e
的值轉換為數字 n
的冪,其中 n = 3
。
另外,請注意,Python 中冪運算的語法是雙星號**
。
from math import e
def getExp(n):
return e**n
print(getExp(3))
輸出:
20.085536923187664
如果你希望控制結果的小數位數,則可以通過將值格式化為字串並在格式化後將其列印出來來實現。
要將浮點值的格式設定為小數位數 n
,我們可以在字串上使用 format()
函式,語法為 {:.nf}
,其中 n
是要顯示的小數位數。
例如,使用上面的相同示例,將輸出格式設定為 5 個小數位。
def getExp(n):
return "{:.5f}".format(e**n);
print(getExp(3))
輸出:
20.08554
在 Python 中使用 math.exp()
來獲取尤拉數
math
模組還具有一個稱為 exp()
的函式,該函式將 e
的值返回為數字的冪。與 math.e
相比,exp()
函式的執行速度大大提高,並且包含可驗證給定 number 引數的程式碼。
對於此示例,請嘗試使用十進位制數字作為引數。
import math
print(math.exp(7.13))
輸出:
1248.8769669132553
另一個示例是通過將引數設定為 1
來確定該值,從而獲得 e
的實際基準值。
import math
print(math.exp(1))
輸出:
2.718281828459045
輸出是 e
的實際值,設定為小數點後 15 位。
在 Python 中使用 numpy.exp()
來獲取尤拉數
NumPy
模組中的 exp()
函式也執行與 math.exp()
相同的操作並接受相同的引數。
不同之處在於,它的執行速度比 math.e
和 math.exp()
都快,而 math.exp()
僅接受標量數,而 numpy.exp()
接受標量數和向量例如陣列和集合。
例如,使用 numpy.exp()
函式來接受浮點數陣列和單個整數值。
import numpy as np
int arr = [3., 5.9, 6.52, 7.13]
int singleVal = 2
print(np.exp(arr))
print(np.exp(singleVal))
輸出:
[20.08553692 365.03746787 678.57838534 1248.87696691]
7.38905609893065
如果將數字陣列用作引數,則它將返回 e
常量結果陣列,該陣列的值提高到給定陣列中所有值的冪。如果給定單個數字作為引數,則其行為將與 math.exp()
完全相同。
總之,要獲取 Python 中的尤拉數或 e
,請使用 math.e
。使用 math.exp()
將需要一個數字作為引數以用作指數值,而將 e
作為其基值。
使用 exp()
來計算雙星號上的 e
的指數時,**
的效果要優於後者,因此,如果要處理大量數字,則最好使用 math.exp()
。
另一種選擇是使用 numpy.exp()
,它支援數字陣列作為引數,並且比 math
模組中的兩種解決方案都執行得更快。因此,如果方程式中包含向量,請改用 numpy.exp()
。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn