在 Python 中從檔案中刪除特定行
- 在 Python 中使用行號從檔案中刪除特定行
- 在 Python 中從檔案中刪除第一行或最後一行
- 刪除與給定特定文字匹配的行
- 刪除與給定特定單詞匹配的行
- 在 Python 中刪除給定檔案中的最短行
- 從 Python 中的給定特定檔案中刪除所有行
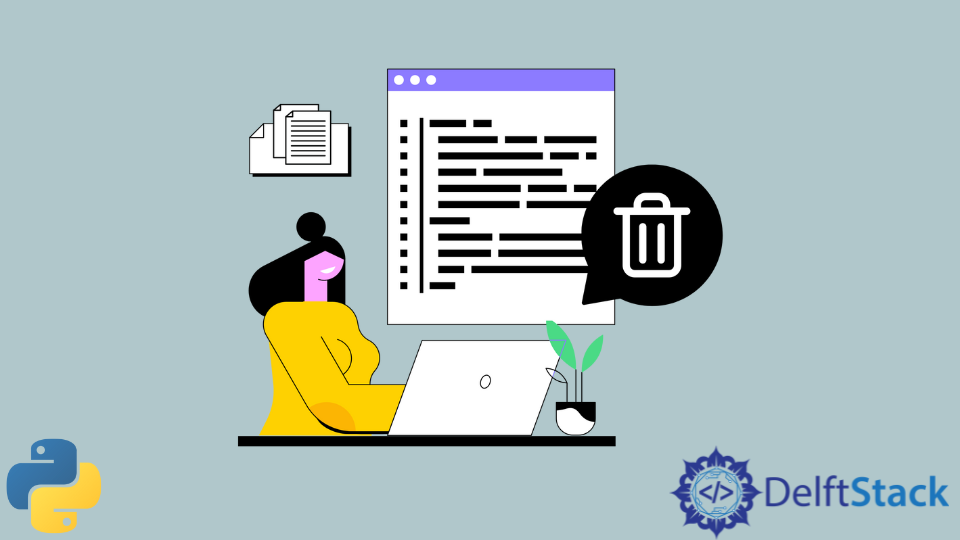
Python 支援和允許資料檔案處理,它構成了 Python 程式語言的重要組成部分。但是,Python 中沒有任何直接函式可以刪除給定檔案中的特定行。
本教程演示了在 Python 中從檔案中刪除特定行的不同方法。
可以藉助幾種不同的方法來實現刪除特定行的任務。
在 Python 中使用行號從檔案中刪除特定行
方法一
如上所述,此方法利用使用者指定的行號從 Python 中的特定檔案中刪除一行。
它使用了 for
迴圈、readlines()
方法和 enumerate()
方法。
讓我們以一個名為 test.txt
的示例檔案為例,其內容如下所述。
Hello
My name is
Adam
I am
a
good
singer
cricketer dancer
以下程式碼使用 enumerate()
和 readlines()
方法從 Python 中的特定檔案中刪除一行。
l1 = []
with open(r"C:\test\test.txt", 'r') as fp:
l1 = fp.readlines()
with open(r"C:\test\test.txt", 'w') as fp:
for number, line in enumerate(l1):
if number not in [4, 6]:
fp.write(line)
上面的程式碼進行了以下更改:
Hello
My name is
Adam
I am
good
cricketer dancer
程式碼說明:
- 首先,檔案以
read
模式開啟。 - 然後在
readlines()
函式的幫助下,將給定檔案中的內容讀入一個列表。 - 然後關閉檔案。
- 然後再次開啟檔案,但這次是在
write
模式下。 - 然後,對建立的列表執行
for
迴圈和enumerate()
函式。 if
條件用於檢查和選擇行號。提到的行號被刪除。- 然後關閉檔案。
方法二
seek()
方法也可用於執行使用行號從檔案中刪除行的相同任務。我們將使用上述方法中提到的同一個檔案 test.txt
。
通過使用 seek()
方法,我們無需兩次開啟同一個檔案,從而更輕鬆、更快捷。
以下程式碼使用 seek()
方法從 Python 中的特定檔案中刪除一行。
with open(r"C:\test\test.txt", 'r+') as fp:
lines = fp.readlines()
fp.seek(0)
fp.truncate()
for number, line in enumerate(lines):
if number not in [4, 6]:
fp.write(line)
上面的程式碼進行了以下更改:
Hello
My name is
Adam
I am
good
cricketer dancer
程式碼說明:
- 檔案以
r+
模式開啟,允許讀取和寫入功能。 - 然後在
readlines()
函式的幫助下將檔案內容讀入列表。 - 然後使用
seek()
方法將指標移回列表的起點。 - 然後在
truncate()
方法的幫助下截斷檔案。 - 然後,對建立的列表執行
for
迴圈和enumerate()
函式。 if
條件用於檢查和選擇行號。提到的行號被刪除。- 檔案關閉。
在 Python 中從檔案中刪除第一行或最後一行
為了實現此方法,我們在將檔案內容寫入列表時利用列表切片。
例如,我們將從上面提到的同一檔案(test.txt
)中刪除第一行。
以下程式碼從 Python 中的給定檔案中刪除第一行。
with open(r"C:\test\test.txt", 'r+') as fp:
lines = fp.readlines()
fp.seek(0)
fp.truncate()
fp.writelines(lines[1:])
上面的程式碼進行了以下更改並提供了以下輸出:
My name is
Adam
I am
a
good
singer
cricketer dancer
程式碼說明:
- 檔案以
r+
模式開啟,允許讀取和寫入功能。 - 藉助
readlines()
函式將檔案內容讀入列表。 - 然後使用
seek()
方法將指標移回列表的起點。 - 然後在
truncate()
方法的幫助下截斷檔案。 - 然後將檔案中的所有行寫入一個列表,但第一行除外。這在列表切片的幫助下成為可能。
刪除與給定特定文字匹配的行
當有多行包含特定文字時,可以使用此方法。然後可以刪除與給定文字匹配的行。
例如,我們將從上述方法中使用的同一檔案(test.txt
)中刪除與給定特定文字匹配的行。
該方法利用 strip()
函式和 write()
函式來實現刪除包含某些給定文字的行的任務。
以下程式碼刪除與 Python 中給定特定字串匹配的行。
with open(r"C:\test\test.txt", 'r') as file:
content = "cricketer dancer"
with open(r"C:\test\test.txt", 'w') as file:
for line in lines:
if line.strip("\n") != content:
file.write(line)
上面的程式碼進行了以下更改並提供了以下輸出:
Hello
My name is
Adam
I am
a
good
singer
刪除與給定特定單詞匹配的行
類似於通過匹配整個字串來刪除一行,我們也可以嘗試找到可能包含在一行中的單詞,然後刪除該特定行。
例如,我們將從上述方法中使用的同一檔案(test.txt
)中刪除與給定特定單詞匹配的行。
對於這種方法,我們使用 Python 提供的 os
模組。我們還建立另一個新檔案並將資料寫入其中以實現此任務。
以下程式碼刪除與 Python 中給定特定單詞匹配的行。
import os
with open(r"C:\test\test.txt", "r") as input:
with open("bb.txt", "w") as output:
for line in input:
if "cricketer" not in line.strip("\n"):
output.write(line)
os.replace('bb.txt', 'aa.txt')
上面的程式碼進行了以下更改並提供了以下輸出:
Hello
My name is
Adam
I am
a
good
singer
在 Python 中刪除給定檔案中的最短行
顧名思義,此方法查詢並刪除給定檔案的最短行。在這裡,我們將使用 len()
方法來實現此任務。
例如,我們將從上述方法中使用的同一檔案(test.txt
)中刪除最短的行。
以下程式碼在 Python 中刪除給定檔案中的最短行。
with open(r"C:\test\test.txt",'r') as rf:
lines = rf.readlines()
shortest = 1000
lineToDelete = ""
for line in lines:
if len(line) < shortest:
shortest = len(line)
lineToDelete = line
with open(r"C:\test\test.txt",'w') as write_file:
for line in lines:
if line == lineToDelete:
pass
else:
write_file.write(line)
從 Python 中的給定特定檔案中刪除所有行
要在 Python 中刪除特定檔案的所有行,我們可以使用 truncate()
函式。此外,檔案指標然後被設定迴檔案的開頭。
以下程式碼從 Python 中的給定特定檔案中刪除所有行。
with open(r"C:\test\test.txt", "r") as fp:
fp.truncate()
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn