在 Python 中轉換 Epoch 到 Datetime
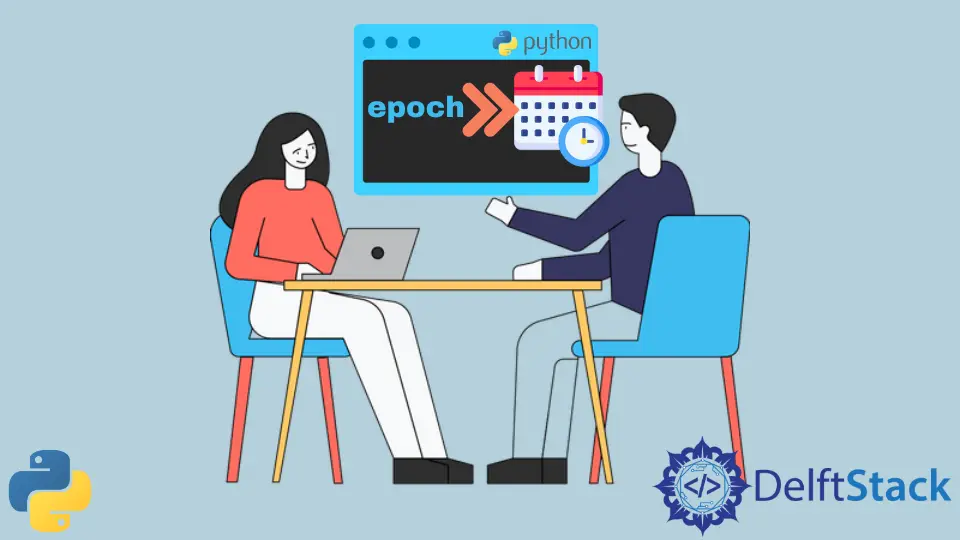
本教程將介紹如何在 Python 中把 Unix epoch 秒數轉換為 datetime 格式。
Epoch/紀元是一個術語,指的是自紀元日期–1970 年 1 月 1 日以來所經過的秒數。1970 年是 UNIX 作業系統的釋出日期。Epoch 時間也被稱為 UNIX 時間戳。
例如,2020 年 11 月 25 日(00:00:00)的紀元是 1606262400
,這是自 1970 年 1 月 1 日到該日的秒數。
在 Python 中使用 time
模組將紀元轉換為日期時間
Python time
模組提供了方便的時間相關函式。該函式為 UNIX 時間戳相關的計算提供了很多支援。
例如,函式 time.localtime()
接受 epoch 秒作為引數,並生成一個由 9 個時間序列組成的時間值序列。
讓我們在這個例子中使用一個隨機的紀元時間。
import time
epoch_time = 1554723620
time_val = time.localtime(epoch_time)
print("Date in time_struct:", time_val)
輸出:
Date in time_struct: time.struct_time(tm_year=2019, tm_mon=4, tm_mday=8, tm_hour=19, tm_min=40, tm_sec=20, tm_wday=0, tm_yday=98, tm_isdst=0)
localtime()
輸出一個元組 struct_time()
,其中包含了紀元時間的詳細資訊,包括確切的年、月、日和其他紀元時間的詳細資訊。
time
模組有另一個函式 time.strftime()
來將元組轉換為日期時間格式。
該函式接受 2 個引數,第一個引數是包含指定轉換格式的字串,第二個引數是 struct_time()
元組。
現在,將上面相同的紀元時間例子轉換為可讀的日期時間格式。
import time
epoch_time = 1554723620
time_formatted = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
輸出:
Formatted Date: 2019-04-08 19:40:20
除了這種格式,另一種通用的日期格式是月份的全稱,包括星期幾。讓我們試一試。
import time
epoch_time = 1554723620
time_formatted = time.strftime("%A, %B %-d, %Y %H:%M:%S", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
Formatted Date: Monday, April 8, 2019 19:40:20
strftime()
中另一種只需要一個字元的有用格式是%c
,它是 Locale 的通用日期和時間表示法。
time_formatted = time.strftime("%c", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
輸出:
Formatted Date: Mon Apr 8 19:40:20 2019
在 Python 中使用 datetime
模組把 Epoch 轉換為 Datetime
datetime
是 Python 中另一個支援時間相關函式的模組。不同的是,time
模組專注於 Unix 時間戳,而 datetime
支援廣泛的日期和時間函式,同時仍然支援 time
模組的許多操作。
datetime
有一個函式 fromtimestamp()
,它接受 epoch 秒和 datetime
物件。
讓我們嘗試一下這個模組的不同的紀元秒的例子。
datetime
是有意重複兩次的。在 datetime
模組中,有一個 datetime
物件,其函式為 fromtimestamp()
。date
和 time
也是 datetime
模組中的物件。import datetime
epoch_time = 561219765
time_val = datetime.datetime.fromtimestamp(epoch_time)
print("Date:", time_val)
如果 datetime.datetime
是專門在 datetime
模組中使用的物件,那麼就從模組中匯入它,以避免程式碼顯得多餘。
from datetime import datetime
輸出:
Date: 1987-10-14 22:22:45
為了將 datetime 轉換為另一種格式,datetime()
還有一個實用函式 strftime()
,它提供了與 time.strftime()
相同的功能。
import datetime
epoch_time = 561219765
time_formatted = datetime.datetime.fromtimestamp(epoch_time).strftime(
"%A, %B %-d, %Y %H:%M:%S"
)
print("Formatted Date:", time_formatted)
輸出:
Formatted Date: Wednesday, October 14, 1987 22:22:45
綜上所述,這就是 Python 中把 epoch 轉換為 datetime 格式的兩種主要方法。time
和 datetime
模組都提供了可以方便地用於日期時間轉換的函式。
這兩個模組都有函式 strftime()
,這是一種方便的格式化日期的方法。
這裡有一個參考文獻,可以熟悉 Python 中的日期時間格式,以便能夠使用 strftime()
自定義日期格式。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn