在 Python 中清除列表
-
使用
clear()
清除 Python 中的列表 - 在 Python 中設定一個空的列表值來清除一個列表
-
在 Python 中使用
del
和切片運算子:
清除列表 -
將整個列表與
0
相乘以清除 Python 中的列表
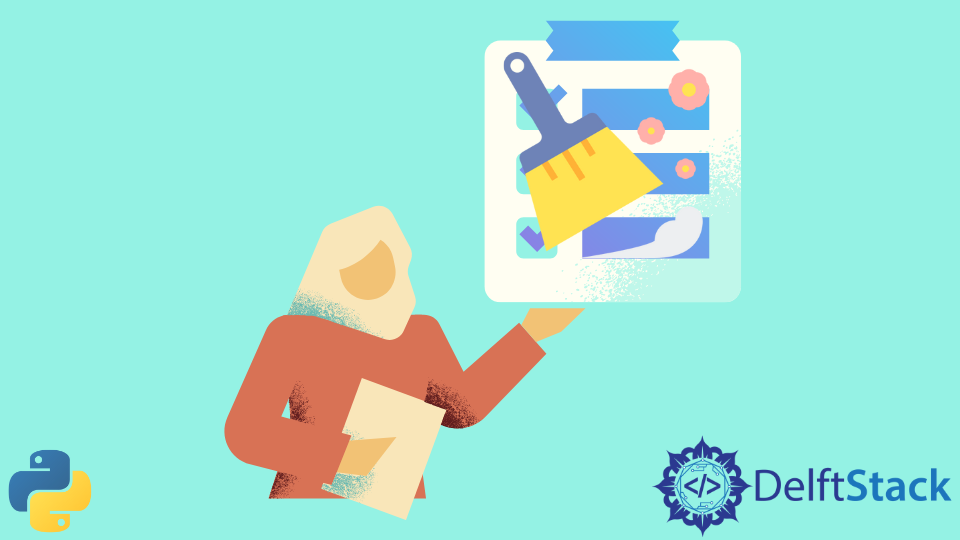
本教程將演示清除 Python 列表的不同方法。
使用 clear()
清除 Python 中的列表
在 Python 中清除列表的最簡單,最明顯的方法是使用 clear()
方法,這是 Python 列表中的內建方法。
例如,使用整數值初始化列表,然後使用 clear()
函式刪除所有值。
lst = [1, 6, 11, 16]
print(lst)
lst.clear()
print(lst)
輸出:
[1, 6, 11, 16]
[]
在 Python 中設定一個空的列表值來清除一個列表
清除現有列表變數的另一種方法是使用一對空的方括號 []
為該變數設定一個空列表。
lst = [1, 6, 11, 16]
print(lst)
lst = []
print(lst)
輸出:
[1, 6, 11, 16]
[]
儘管這樣做也可以快速清除列表,但這不會從記憶體中刪除列表的值。而是建立一個列表的新例項,並指向新建立的例項。
如果沒有其他變數指向列表變數的先前值,則這些變數將不可訪問並且將用於垃圾回收。
如果我們使兩個變數指向同一列表,並在之後將其中一個變數設定為空列表,則可以清楚地看出這種情況。
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst = []
print(lst)
print(lst2)
輸出:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[1, 6, 11, 16]
第二個列表 lst2
被設定為 lst
的值,但是即使在將新的 lst
值設定為空列表之後,列表 lst2
仍然保留以前的舊值。在這種情況下,先前的值不適合進行垃圾回收,因為仍然有指向它的變數。
在 Python 中使用 del
和切片運算子:
清除列表
Python 關鍵字 del
用於完全刪除記憶體中的物件及其引用。del
關鍵字用於刪除 Python 物件,可迭代物件,列表的一部分等。
Python 中的冒號運算子:
是用於將陣列切成可接受兩個整數引數的部分的運算子。
而:
操作符則是用來獲取列表的實際引用進行刪除。
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
del lst[:]
print(lst)
print(lst2)
另一個不使用 del
關鍵字即可完成此操作的解決方案是將列表設定為空列表,但使用:
運算子將以前的引用替換為空列表。
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst[:] = []
print(lst)
print(lst2)
兩種解決方案的輸出如下:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[]
從前面的示例中可以注意到,將現有列表變數設定為空列表實際上並不會刪除先前的值。這個解決方案將完全刪除之前的值,即使其他變數指向主列表中相同的值引用。
將整個列表與 0
相乘以清除 Python 中的列表
清除列表的最快方法是將整個列表乘以 0
。將列表變數本身與 0
相乘將清除列表並清除其先前在記憶體中的引用。
lst = [1, 6, 11, 16]
lst2 = lst
print(lst)
print(lst2)
lst *= 0
print(lst)
print(lst2)
輸出:
[1, 6, 11, 16]
[1, 6, 11, 16]
[]
[]
在所有提到的解決方案中,此方法是執行最快的解決方案。
總之,清除列表的最明顯方法是在列表中使用內建的 clear()
函式,儘管執行最快的解決方案是將列表變數與值 0
相乘。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn