在 Python 中將列表的列表連線為列表
- 為什麼我們需要將列表的列表連線為列表
-
使用
for
迴圈將列表加入列表 - 使用列表推導將列表加入列表
- Python 中使用遞迴將列表的列表連線為列表
- 使用內建 Python 函式將列表的列表連線為列表
- 使用 Functools 將列表的列表連線為列表
-
使用
Chain()
方法將列表的列表連線為列表 - 使用 Numpy 模組將列表的列表連線為列表
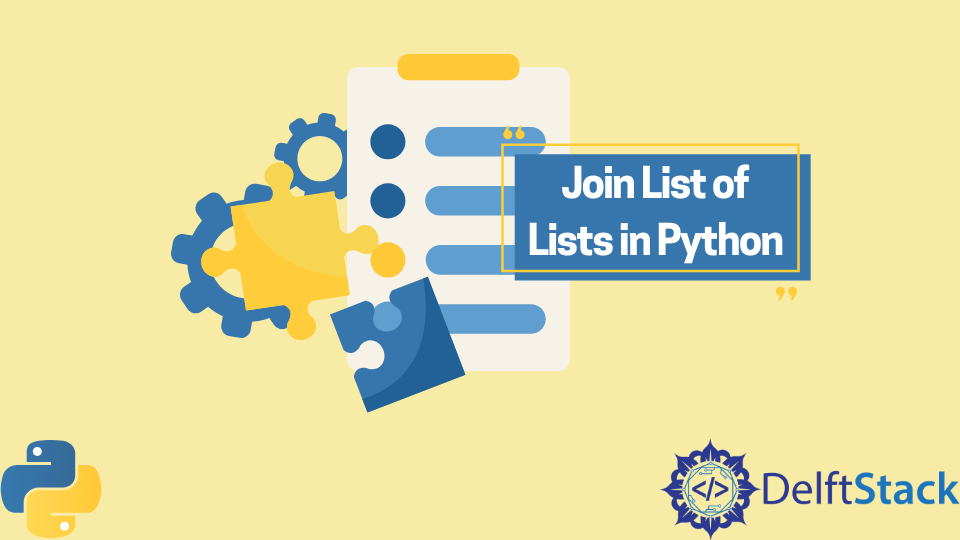
本文提供了在 Python 中將列表加入列表的各種方法。在我們瞭解如何將列表列表加入 Python 中的列表之前,讓我們瞭解要求以及此加入過程稱為什麼。
為什麼我們需要將列表的列表連線為列表
列表列表是一種二維格式,也稱為巢狀列表,包含多個子列表。列表是 Python 中最靈活的資料結構之一,因此將列表列表加入單個一維列表可能是有益的。
在這裡,我們將這個列表列表轉換為一個單一結構,其中新列表將包含列表列表中每個子列表中包含的元素。在 Python 語言中,這個過程也稱為展平。
包含所有元素的一維列表是一種更好的方法,因為:
- 它使程式碼清晰易讀,以獲得更好的文件,因為從單一結構比二維結構更容易閱讀。
- 一維列表在資料科學應用程式中很有幫助,因為你可以快速讀取、過濾或刪除列表中的專案。
- 當你希望基於集合論過濾資料時,列表也是首選。
使用 for
迴圈將列表加入列表
將列表列表轉換為一維列表的最簡單方法是實現巢狀 for 迴圈,其中包含不同元素的 if 條件。你可以通過以下方式做到這一點:
Listoflists = [[100,90,80],[70,60],[50,40,30,20,10]] #List of Lists
mylist = [] #new list
for a in range(len(Listoflists)): #List of lists level
for b in range (len(Listoflists[a])): #Sublist Level
mylist.append(Listoflists[a][b]) #Add element to mylist
print("List of Lists:",Listoflists)
print("1-D List:",mylist)
輸出:
List of Lists: [[100, 90, 80], [70, 60], [50, 40, 30, 20, 10]]
1-D List: [100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
在上面的程式碼片段中,我們使用了巢狀的 for 迴圈。第一個迴圈選擇主列表中的每個元素並檢查它是否是列表型別。
如果子元素是一個列表,它會啟動另一個 for
迴圈來迭代這個子列表並將其值新增到新列表中。否則,它會附加在該位置找到的單個元素。
使用巢狀的 for
迴圈適用於規則和不規則的列表列表,但這是一種蠻力方法。
使用列表推導將列表加入列表
將巢狀列表轉換為單個列表的另一種方法是使用列表推導。列表推導式提供了易於理解的單行乾淨程式碼,可以使用其他結構(如字串、陣列、巢狀列表等)建立全新的列表。
讓我們看看如何使用列表推導來解決我們的問題:
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]]
#mylist defined using list comprehension
mylist = [item for sublist in listoflists for item in sublist]
print('List of Lists', listoflists)
print('1-D list', mylist)
輸出:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的程式碼片段中,我們指定建立一個新列表,其中包含原始列表列表中每個子列表的每個專案提供的元素。你還可以將包含各種資料型別元素的子列表組合到一維列表中。
Python 中使用遞迴將列表的列表連線為列表
遞迴提供了另一種在 python 中展平列表列表的方法。讓我們看看如何實現遞迴以從列表列表建立單個列表:
def to1list(listoflists):
if len(listoflists) == 0:
return listoflists
if isinstance(listoflists[0], list):
return to1list(listoflists[0]) + to1list(listoflists[1:])
return listoflists[:1] + to1list(listoflists[1:])
print("MyList:",to1list([["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]]))
輸出:
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的程式碼片段中,我們定義了一個名為 to1list
的遞迴函式,它接受一個列表列表,listoflists
是它的引數。如果列表列表包含單個元素,則按原樣返回。
否則,使用帶有 isinstance()
的 if 語句來檢查列表列表中第 0 個位置的列表是否為列表。
如果為真,那麼我們返回 [0]
處子列表的值並再次呼叫我們的函式 to1list
,但這一次,跳過第 0 個位置並通過指定切片並傳遞子列表從第一個位置開始-list 作為引數。
現在讓我們看看如何使用內建函式將巢狀列表連線到列表中。
使用內建 Python 函式將列表的列表連線為列表
我們可以使用 sum() 函式和 lambda 函式來連線 Python 中的列表列表。
使用 sum()
函式將列表的列表連線為列表
你可以使用內建的 sum 函式從列表列表中建立一維列表。
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]] #List to be flattened
mylist = sum(listoflists, [])
print("List of Lists:",listoflists)
print("My List:",mylist)
輸出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
在上面的程式中,sum 函式傳遞了兩個引數;第一個是它將迭代的列表列表,第二個是我們最初為空的一維列表的開始。
使用 Lambda 函式加入列表列表
你還可以使用 lambda(即匿名函式)將列表列表轉換為列表。讓我們看一個程式碼示例:
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]] #List to be flattened
to1list = lambda listoflists:[element for item in listoflists for element in to1list(item)] if type(listoflists) is list else [listoflists]
print("List of lists:", listoflists)
print("MyList:", to1list(listoflists))
輸出:
List of lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的程式中,我們定義了一個 lambda 函式,它迭代專案的每個元素,即列表列表中的子列表,並使用 to1list(item)
將該元素新增到單個列表中。
讓我們看看如何使用 Python 庫將列表列表轉換為一維列表。
使用 Functools 將列表的列表連線為列表
在 Python 中將二維列表轉換為一維列表的最快方法之一是使用其 Functools 模組,該模組提供的函式可以有效地處理更高或複雜的函式。
我們可以使用 Functools 模組中提供的 reduce()
函式將一個列表加入到一個列表中,如下程式碼所示:
import operator
import functools
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]]
#mylist using functools
mylist = functools.reduce(operator.iconcat, listoflists, [])
print('List of Lists', listoflists)
print('1-D list', mylist)
輸出:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的程式碼片段中,新的一維列表是用 reduce()
函式定義的,該函式使用 operator.iconcat
函式連線我們從左到右移動的列表列表的子列表中的所有元素.
最好記住為 functools
和 operator
新增匯入,因為這種方法需要兩者。
使用 Chain()
方法將列表的列表連線為列表
另一個 Python 庫,稱為 Itertools
,包含一個 chain()
方法,你可以使用該方法將列表列表轉換為一維列表。讓我們看看如何:
import itertools
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]] #List to be flattened
mylist = list(itertools.chain(*listoflists))
print("List of Lists:",listoflists)
print("My List:",mylist)
輸出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上述程式中,chain()
方法用於迭代列表列表中的所有子列表,並返回包含所有子列表的所有元素的單個可迭代列表。
使用 Numpy 模組將列表的列表連線為列表
Numpy 庫提供了連線子字串並將它們展平為單個一維列表的函式。讓我們看看如何使用 numpy.concatenate()
將巢狀列表轉換為列表:
import numpy
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12,8.6,14.01], [100,100]] #List to be flattened
mylist = list(numpy.concatenate(listoflists).flat)
print("List of Lists:",listoflists)
print("My List:",mylist)
輸出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
在上面的程式碼片段中,我們使用了列表列表,與上面的程式相同,並使用 NumPy
的 concatenate()
和 flat()
函式建立了一個名為 mylist
的新列表。