如何在 Python 中基於多個定界符分割字串
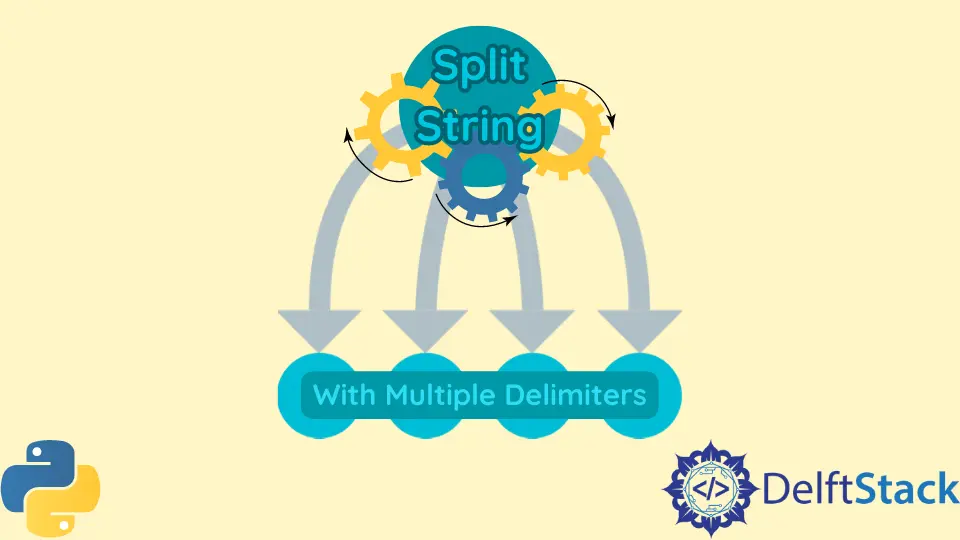
Python 字串 split()
方法允許根據分隔符將字串輕鬆拆分為列表。儘管在某些情況下,你可能需要不僅基於一個分隔符值,而且還要基於多個定界符值進行分隔。這篇文章介紹了可以在 Python 中實現的兩種便捷方法。
具有兩個定界符的 Python 分割字串
假設以下字串。
text = "python is, an easy;language; to, learn."
對於我們的示例,我們需要用分號,空格 (; )
或逗號和空格 (, )
進行拆分。在這種情況下,不應該考慮出現任何奇數的分號或逗號,即沒有尾部空格的 ,
或 ;
。
正規表示式
儘管正規表示式的使用由於字串解析的昂貴特性而不被推薦使用,但是在這種情況下可以放心使用正規表示式。
使用正規表示式
Python 的內建模組 re
具有一種可用於這種情況的 split()
方法。
讓我們使用基本的 a 或 b 正規表示式(a|b
)來分隔多個定界符。
import re
text = "python is, an easy;language; to, learn."
print(re.split("; |, ", text))
輸出:
['python is', 'an easy;language', 'to', 'learn.']
如 Wikipedia 頁面所述,正規表示式使用 IEEE POSIX 作為其語法的標準。通過引用此標準,我們可以管理幾種其他方式來編寫符合用例的正規表示式。
無需使用豎線分隔符(|
)來定義分隔符,我們可以使用正規表示式中提供的 Range([]
)語法實現相同的結果。你可以通過在方括號內提供正規表示式可以匹配的字元範圍。
因此,當指定正規表示式的模式時,我們可以簡單地在方括號內提供分號和逗號,以及一個額外的空格 [[;,])
,這將導致正規表示式與具有[分號或逗號]和尾隨空格。
import re
text = "python is, an easy;language; to, learn."
print(re.split("[;,] ", text))
建立一個函式
前面提到的正規表示式僅限於硬編碼的分隔符集。稍後在進行定界符修改時,這可能會帶來麻煩,並且還會限制其在程式碼其他部分的可重用性。因此,最好使用最佳實踐來考慮使程式碼更加通用和可重用。因此,為了安全起見,讓我們將該邏輯編碼到 Python 函式中。
import re
text = "python is, an easy;language; to, learn."
separators = "; ", ", "
def custom_split(sepr_list, str_to_split):
# create regular expression dynamically
regular_exp = "|".join(map(re.escape, sepr_list))
return re.split(regular_exp, str_to_split)
print(custom_split(separators, text))
使用字串函式
如果你想避免使用正規表示式,或者不必為了拆分字串而在專案中引入新模組,則可以使用 replace()
和 split()
方法出現在字串模組中本身達到相同的結果。
text = "python is, an easy;language; to, learn."
# transform [semicolon-space] parts of the string into [comma-space]
text_one_delimiter = text.replace("; ", ", ")
print(text_one_delimiter.split(", "))
首先,我們用其他定界符替換逗號中所有出現的分號,後跟空格 (; )
,該分隔符是逗號,後跟空格 (, )
。這樣,我們可以將字串拆分限制為一個定界符,在這種情況下,它是一個逗號,後跟一個空格 (, )
。
現在,我們可以使用 Python 字串模組內建的簡單 split()
函式安全地拆分修改後的字串,以得到相同的結果。
請注意,這次我們尚未將任何新模組匯入程式碼中以實現結果。
具有多個定界符的 Python 拆分字串
參考下面提到的文字。
text = "python is# an% easy;language- to, learn."
對於此示例,我們需要在所有例項上將其分割為文字具有任何字元 # % ; - ,
,後跟一個空格。
正規表示式
在這種情況下,我們可以在定義正規表示式時輕鬆新增其他分隔符。
import re
text = "python is# an% easy;language- to, learn."
print(re.split("; |, |# |% |- ", text))
輸出:
['python is', 'an', 'easy;language', 'to', 'learn.']
作為一個函式
同樣在這種情況下,我們可以簡單地使用我們之前使用的帶有兩個定界符的程式碼,只需將所有其他分隔符新增到 separators
變數中即可。
import re
text = "python is# an% easy;language- to, learn."
separators = "; ", ", ", "# ", "% ", "- "
def custom_split(sepr_list, str_to_split):
# create regular expression dynamically
regular_exp = "|".join(map(re.escape, sepr_list))
return re.split(regular_exp, str_to_split)
print(custom_split(separators, text))
使用字串函式
與我們之前使用兩個定界符處理它的方式類似,我們可以使用 replace()
和 split()
函式也可以解決此問題。
text = "python is, an easy;language; to, learn."
# transform [semicolon-space] parts of the string into [comma-space]
text_one_delimiter = (
text.replace("# ", ", ").replace("% ", ", ").replace("; ", ", ").replace("- ", ", ")
)
print(text_one_delimiter.split(", "))
輸出:
['python is', 'an easy;language', 'to', 'learn.']
應該注意的是,在這種情況下,當用於更高的定界符計數時,不建議使用此方法。由於在這種情況下,必須多次使用字串 replace()
方法才能對字串進行多次遍歷,因此最終將導致不希望的效能峰值,使用正規表示式可以輕鬆避免。