如何在 Python 中檢查字串是否包含數字
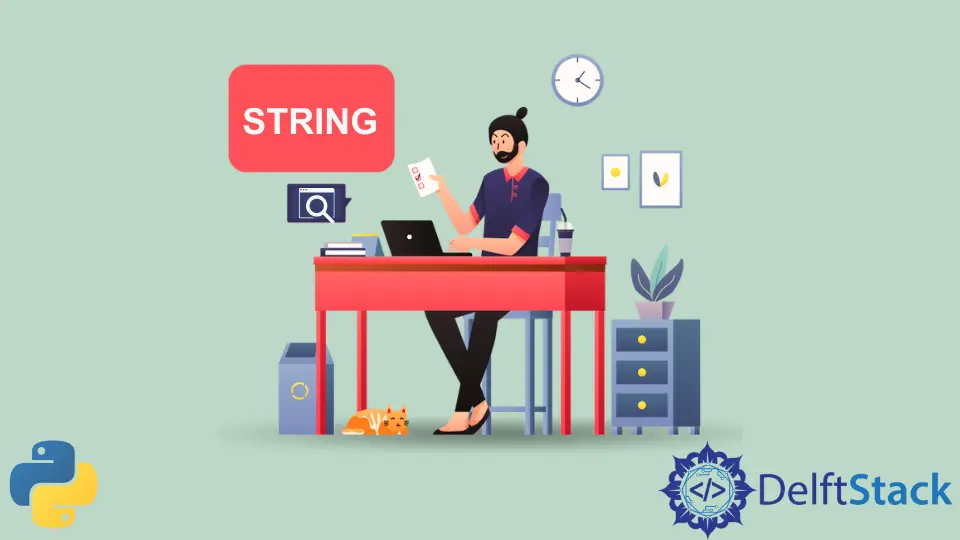
本文介紹如何檢查 Python 字串是否包含數字。
如果給定的字串包含數字,則 Python 內建的 any
函式與 str.isdigit
一起將返回 True
。否則返回 False
。
如果給定的字串包含數字,則模式為 r'\d'
的 Python 正規表示式搜尋方法也可以返回 True
。
帶有 str.isdigit
的 Python any
函式來檢查字串是否包含數字
如果給定的 Python 迭代物件的任何元素為 True
,則 any
函式返回 True
,否則,返回 False
。
如果給定字串中的所有字元均為數字,則 str.isdigit()
返回 True
,否則返回 False
。
any(chr.isdigit() for chr in stringExample)
如果 stringExample
至少包含一個數字,那麼上面的程式碼將返回 True
,因為 chr.isdigit() for chr in stringExample
在可迭代物件中至少有一個 True
。
工作示例
str1 = "python1"
str2 = "nonumber"
str3 = "12345"
print(any(chr.isdigit() for chr in str1))
print(any(chr.isdigit() for chr in str2))
print(any(chr.isdigit() for chr in str3))
輸出:
True
False
True
map()
函式
Python map(function, iterable)
函式將函式 function
應用於給定可迭代物件的每個元素,並返回一個迭代器產生以上結果。
因此,我們可以將上述解決方案重寫為
any(map(str.isdigit, stringExample))
工作示例
str1 = "python1"
str2 = "nonumber"
str3 = "12345"
print(any(map(str.isdigit, str1)))
print(any(map(str.isdigit, str2)))
print(any(map(str.isdigit, str3)))
輸出:
True
False
True
re.search(r'\d')
檢查字串是否包含數字
re.search(r'\d', string)
函式掃描 string
並返回匹配物件對於與給定模式匹配的第一個位置- \d
,表示在 0 到 9 之間的任何數字,如果找不到匹配項,則返回 None
。
import re
print(re.search(r"\d", "python3.8"))
# output: <re.Match object; span=(6, 7), match='3'>
match
物件包含 2 個元組的 span
,它們是 match
的開始和結束位置,以及匹配的內容,例如 match = '3'
。
bool()
函式可以將 match
物件或 None
的 re.search
結果轉換為 True
或 False
。
工作示例
import re
str1 = "python12"
str2 = "nonumber"
str3 = "12345"
print(bool(re.search(r"\d", str1)))
print(bool(re.search(r"\d", str2)))
print(bool(re.search(r"\d", str3)))
輸出:
True
False
True
就執行時間而言,正規表示式評估比使用內建的字串函式要快得多。如果字串的值相當大,那麼 re.search()
比使用字串函式更理想。
在給定字串上執行 search()
函式之前,使用 re.compile()
編譯表示式,也會使執行時間更快。
將 compile()
的返回值捕獲到一個變數中,然後在這個變數中呼叫 search()
函式。在這種情況下,search()
只需要一個引數,即對照編譯後的表示式搜尋的字串。
def hasNumber(stringVal):
re_numbers = re.compile("\d")
return False if (re_numbers.search(stringVal) == None) else True
綜上所述,內建函式 any()
和 isdigit()
可以很容易地串聯使用,檢查一個字串是否包含一個數字。
然而,在正規表示式模組 re
中使用實用函式 search()
和 compile()
會比內建的字串函式更快地生成結果。所以,如果你要處理大的數值或字串,那麼正規表示式的解決方案要比字串函式更理想。
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn