處理 Python 斷言錯誤並查詢錯誤源
- 在 Python 中處理斷言錯誤並查詢錯誤源
-
使用
Try-Except
塊處理 Python 中的斷言錯誤 -
使用帶有
Try-Except
塊的日誌記錄模組來處理 Python 中的斷言錯誤 - 使用 Traceback 模組處理 Python 中的斷言錯誤
-
在 Python 中使用
print
語句手動處理AssertionError
異常 - まとめ
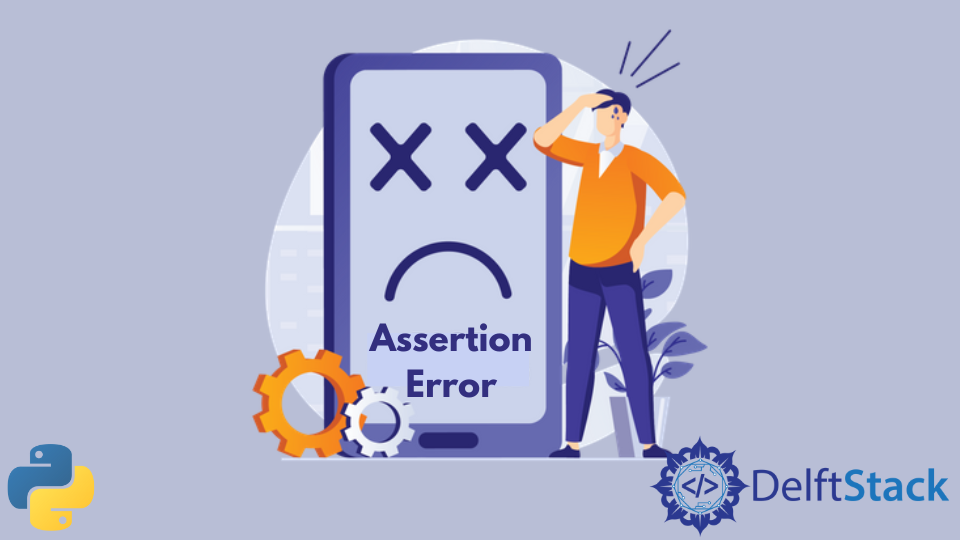
在本文中,我們將學習如何以不同的方式處理 Python 的斷言錯誤。我們還看到了識別引發此錯誤的語句的方法。
在 Python 中處理斷言錯誤並查詢錯誤源
在 Python 中,我們可以使用 assert
語句來檢查程式碼中的任何條件。如果條件為 True
,則控制更進一步。
但是如果條件結果為 False
,我們就會得到 AssertionError
,程式的流程就會被打亂。
assert 語句的語法如下。
assert statement,message
這裡,statement
是一個布林語句。如果它評估為 False
,程式將引發 AssertionError
。
message
是可選的,並在 AssertionError
發生時列印。如果 statement
的計算結果為 True
,則什麼也不會發生。
這就是 Python 引發 AssertionError
異常的方式。
assert True == False, "Whoops, something went wrong!"
print(True)
輸出:
Traceback (most recent call last):
File "Desktop/Tut.py", line 2, in <module>
assert True == False, "Whoops, something went wrong!"
AssertionError: Whoops, something went wrong!
你可以觀察到我們使用了語句 True==False
,其計算結果為 False
。因此,程式會引發 AssertionError
異常。
我們可以通過多種方式處理此異常。讓我們一個接一個地瀏覽它們。
使用 Try-Except
塊處理 Python 中的斷言錯誤
嘗試執行以下程式碼。
try:
assert 123 == 256432
except AssertionError:
print ("There is some problem!")
輸出:
There is some problem!
在這裡,assert
語句檢查兩個數字是否相等。由於這些數字不相等,AssertionError
異常從 try
塊引發。
except
塊捕獲異常並執行列印語句。在這裡,我們在異常塊的 print 語句中獲得了輸出。
要知道異常的來源在哪裡,我們可以使用 raise
關鍵字在 except
塊中重新引發異常。
raise
關鍵字將在出現異常時引發錯誤並停止程式。它有助於跟蹤當前的異常。
raise
語句的語法如下。
raise {exception class}
異常可以是內建異常,我們也可以建立自定義異常。我們還可以使用 raise
關鍵字列印一些訊息並建立自定義異常。
raise Exception("print some string")
這個例子展示了 raise
關鍵字的工作原理。
try:
assert 1 == 2
except AssertionError:
print ("There is some problem!")
raise
輸出:
There is some problem!
Traceback (most recent call last):
File "Desktop/Tut.py", line 2, in <module>
assert 1 == 2
AssertionError
我們在上面程式碼的 except
塊中重新引發了異常。你可以觀察使用 raise
關鍵字如何在 line 2
中給出異常源。
通過這種方式,我們可以獲得異常的行號以及引發程式碼的確切錯誤部分。
使用帶有 Try-Except
塊的日誌記錄模組來處理 Python 中的斷言錯誤
Python 中的 logging
模組可幫助你跟蹤應用程式的執行和錯誤。該模組跟蹤任何操作期間發生的事件。
這在發生崩潰時很有幫助,因為我們可以從日誌中找到以前的資料。因此,如果出現任何問題,我們可以回顧並找出導致錯誤的原因。
我們可以匯入 logging
模組並在 except
塊中使用 logging.error
方法。
import logging
try:
assert True == False
except AssertionError:
logging.error("Something is quite not right!", exc_info=True)
輸出:
ERROR:root:Something is quite not right!
Traceback (most recent call last):
File "Desktop/Tut.py", line 4, in <module>
assert True == False
AssertionError
此方法還返回異常的行號和確切來源。
該模組有許多物件用於不同型別的錯誤訊息。這些物件在記錄器上記錄具有級別的訊息。
例如,Logger.critical(message)
記錄具有 critical
級別的訊息。Logger.error(message)
在上面的程式碼中記錄級別 error
的訊息。
使用 Traceback 模組處理 Python 中的斷言錯誤
當程式碼有多個斷言語句時,traceback
模組有助於捕捉錯誤的確切來源。
import sys
import traceback
try:
assert 88 == 88
assert 1 == 101
assert True
except AssertionError:
_, _, var = sys.exc_info()
traceback.print_tb(var)
tb_info = traceback.extract_tb(var)
filename, line_number, function_name, text = tb_info[-1]
print("There is some error in line {} in this statement: {}".format(line_number, text))
exit(1)
輸出:
File "Desktop/Tut.py", line 6, in <module>
assert 1 == 101
There is some error in line 6 in this statement: assert 1 == 101
使用 traceback
模組,我們可以編寫帶有佔位符 {}
的 print
語句。
此外,我們可以指定不同的變數來儲存檔名、行號、函式名和發生異常的文字。
這裡,tb
指的是回溯物件。我們在 print 語句中只使用了兩個佔位符,一個用於行號,另一個用於文字。
sys.exc_info()
函式返回 raise 語句的三個部分 - exc_type
、exc_traceback
和 exc_value
。讓我們在檔名的 print 語句中放置另一個佔位符。
import sys
import traceback
try:
assert 88 == 88
assert 1 == 101
assert True
except AssertionError:
_, _, var = sys.exc_info()
traceback.print_tb(var)
tb_info = traceback.extract_tb(var)
filename, line_number, function_name, text = tb_info[-1]
print("There is some error in the file {} on line {} in this statement: {}".format(filename, line_number, text))
exit(1)
輸出:
File "Desktop/Tut.py", line 6, in <module>
assert 1 == 101
There is some error in the file Desktop/Tut.py on line 6 in this statement: assert 1 == 101
這次我們還得到了檔案的完整 URL 作為檔名。
有關回溯模組的更多詳細資訊,請參閱此文件。
在 Python 中使用 print
語句手動處理 AssertionError
異常
我們可以在 except
塊中使用 print
語句來手動處理異常。
try:
assert True == False, "Operation is invalid"
print(True)
except AssertionError as warn:
print(warn)
輸出:
Operation is invalid
使用者提供的任何錯誤訊息都會進入 print
語句並被列印出來。這樣,使用者就不必擔心技術錯誤。
顯示一個簡單的訊息而不是錯誤。
まとめ
本文展示了我們如何在 Python 中處理 AssertionError
。我們討論了使用 raise 關鍵字、日誌記錄模組和回溯模組來處理斷言錯誤。
我們還看到了如何使用簡單的列印語句手動處理 AssertionError
異常。在實際應用中,不使用 AssertionError
。
如果你僅在開發和測試程式時使用它會有所幫助。