查詢 Python 物件的方法
-
使用
dir
方法查詢 Python 物件的方法 -
使用
type
函式查詢 Python 物件型別 -
使用
id
函式查詢 Python 物件 ID -
使用
inspect
模組查詢 Python 物件的方法 -
使用
hasattr()
方法查詢 Python 物件 -
使用
getattr()
方法查詢物件
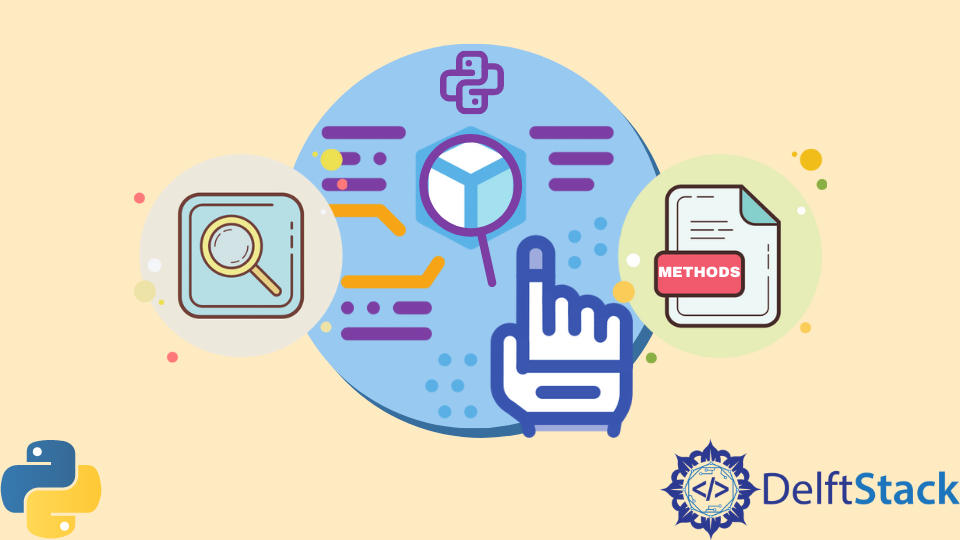
在 Python 程式設計中,動態查詢 Python 物件的方法的能力稱為自省。由於 Python 中的一切都是物件,因此我們可以在執行時輕鬆找到它的物件。
我們可以使用內建函式和模組檢查那些。當我們想在不閱讀原始碼的情況下了解資訊時,它特別有用。
本文介紹了我們可以用來查詢 Python 物件的方法的六種簡單方法。讓我們深入瞭解一下。
使用 dir
方法查詢 Python 物件的方法
查詢方法的第一種方法是使用 dir()
函式。該函式將一個物件作為引數並返回該物件的屬性和方法列表。
這個函式的語法是:
# python 3.x
dir(object)
例如:
# python 3.x
my_object = ['a', 'b', 'c']
dir(my_object)
輸出:
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
從輸出中,我們可以觀察到它已經返回了物件的所有方法。
以雙下劃線開頭的函式稱為 dunder 方法。這些方法稱為包裝物件。例如,dict()
函式將呼叫 __dict__()
方法。
我們已經建立了這個基本的 Vehicle Python 類:
# python 3.x
class Vehicle():
def __init__(self, wheels=4, colour='red'):
self.wheels = wheels
self.colour = colour
def repaint(self, colour=None):
self.colour = colour
如果我們建立這個類的物件並執行 dir()
函式,我們可以看到以下輸出:
# python 3.x
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'colour', 'repaint', 'wheels']
我們可以看到它列出了所有方法及其屬性。它顯示了我們建立的方法,但也列出了該類的所有內建方法。
此外,我們還可以通過使用 callable()
函式並將物件作為引數傳遞來檢查該方法是否可呼叫。
使用 type
函式查詢 Python 物件型別
第二種方法是使用 type()
函式。type()
函式用於返回物件的型別。
我們可以在 type()
函式的引數中傳遞任何物件或值。例如:
# python 3.x
print(type(my_object))
print(type(1))
print(type("hello"))
這將顯示以下輸出:
<class 'list'>
<class 'int'>
<class 'str'>
type()
函式返回了一個物件的型別。
使用 id
函式查詢 Python 物件 ID
要在 Python 中找出物件的 id,我們將使用 id()
函式。
此函式返回作為引數傳遞的任何物件的特殊 id。id 類似於該特定物件在記憶體中的特殊位置。
例如:
# python 3.x
print(id(my_object))
print(id(1))
print(id("Hello"))
執行這些命令後,我們將得到類似的輸出:
140234778692576
94174513879552
140234742627312
使用 inspect
模組查詢 Python 物件的方法
inspect
模組是我們可以用來檢視有關實時 Python 物件的資訊的另一種方法。該模組的語法是:
# python 3.x
import inspect
print(inspect.getmembers(object))
第一步是匯入 inspect
模組。之後,我們將從 inspect
模組呼叫 getmembers()
函式並將物件作為引數傳遞。
例如:
# python 3.x
print(inspect.getmembers(my_object))
print(inspect.getmembers(Vehicle))
在上面的例子中,我們檢查了兩個物件:一個列表和 Vehicle 類的物件。執行程式碼後,我們得到以下輸出:
# python 3.x
[('__add__', <method-wrapper '__add__' of list object at 0x7f8af42b4be0>), ('__class__', <class 'list'>), ('__contains__', <method-wrapper '__contains__' of list object at 0x7f8af42b4be0>), ('__delattr__', <method-wrapper '__delattr__' of list object at 0x7f8af42b4be0>), ('__delitem__', <method-wrapper '__delitem__' of list object at 0x7f8af42b4be0>), ('__dir__', <built-in method __dir__ of list object at 0x7f8af42b4be0>), ('__doc__', 'Built-in mutable sequence.\n\nIf no argument is given, the constructor creates a new empty list.\nThe argument must be an iterable if specified.'), ('__eq__', <method-wrapper '__eq__' of list object at 0x7f8af42b4be0>), ('__format__', <built-in method __format__ of list object at 0x7f8af42b4be0>), ('__ge__', <method-wrapper '__ge__' of list object at 0x7f8af42b4be0>), ('__getattribute__', <method-wrapper '__getattribute__' of list object at 0x7f8af42b4be0>), ('__getitem__', <built-in method __getitem__ of list object at 0x7f8af42b4be0>), ('__gt__', <method-wrapper '__gt__' of list object at 0x7f8af42b4be0>), ('__hash__', None), ('__iadd__', <method-wrapper '__iadd__' of list object at 0x7f8af42b4be0>), ('__imul__', <method-wrapper '__imul__' of list object at 0x7f8af42b4be0>), ('__init__', <method-wrapper '__init__' of list object at 0x7f8af42b4be0>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b668d5a0>), ('__iter__', <method-wrapper '__iter__' of list object at 0x7f8af42b4be0>), ('__le__', <method-wrapper '__le__' of list object at 0x7f8af42b4be0>), ('__len__', <method-wrapper '__len__' of list object at 0x7f8af42b4be0>), ('__lt__', <method-wrapper '__lt__' of list object at 0x7f8af42b4be0>), ('__mul__', <method-wrapper '__mul__' of list object at 0x7f8af42b4be0>), ('__ne__', <method-wrapper '__ne__' of list object at 0x7f8af42b4be0>), ('__new__', <built-in method __new__ of type object at 0x55a6b668d5a0>), ('__reduce__', <built-in method __reduce__ of list object at 0x7f8af42b4be0>), ('__reduce_ex__', <built-in method __reduce_ex__ of list object at 0x7f8af42b4be0>), ('__repr__', <method-wrapper '__repr__' of list object at 0x7f8af42b4be0>), ('__reversed__', <built-in method __reversed__ of list object at 0x7f8af42b4be0>), ('__rmul__', <method-wrapper '__rmul__' of list object at 0x7f8af42b4be0>), ('__setattr__', <method-wrapper '__setattr__' of list object at 0x7f8af42b4be0>), ('__setitem__', <method-wrapper '__setitem__' of list object at 0x7f8af42b4be0>), ('__sizeof__', <built-in method __sizeof__ of list object at 0x7f8af42b4be0>), ('__str__', <method-wrapper '__str__' of list object at 0x7f8af42b4be0>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b668d5a0>), ('append', <built-in method append of list object at 0x7f8af42b4be0>), ('clear', <built-in method clear of list object at 0x7f8af42b4be0>), ('copy', <built-in method copy of list object at 0x7f8af42b4be0>), ('count', <built-in method count of list object at 0x7f8af42b4be0>), ('extend', <built-in method extend of list object at 0x7f8af42b4be0>), ('index', <built-in method index of list object at 0x7f8af42b4be0>), ('insert', <built-in method insert of list object at 0x7f8af42b4be0>), ('pop', <built-in method pop of list object at 0x7f8af42b4be0>), ('remove', <built-in method remove of list object at 0x7f8af42b4be0>), ('reverse', <built-in method reverse of list object at 0x7f8af42b4be0>), ('sort', <built-in method sort of list object at 0x7f8af42b4be0>)] [('__class__', <class '__main__.Vehicle'>), ('__delattr__', <method-wrapper '__delattr__' of Vehicle object at 0x7f8af813a350>), ('__dict__', {'wheels': 4, 'colour': 'red'}), ('__dir__', <built-in method __dir__ of Vehicle object at 0x7f8af813a350>), ('__doc__', None), ('__eq__', <method-wrapper '__eq__' of Vehicle object at 0x7f8af813a350>), ('__format__', <built-in method __format__ of Vehicle object at 0x7f8af813a350>), ('__ge__', <method-wrapper '__ge__' of Vehicle object at 0x7f8af813a350>), ('__getattribute__', <method-wrapper '__getattribute__' of Vehicle object at 0x7f8af813a350>), ('__gt__', <method-wrapper '__gt__' of Vehicle object at 0x7f8af813a350>), ('__hash__', <method-wrapper '__hash__' of Vehicle object at 0x7f8af813a350>), ('__init__', <bound method Vehicle.__init__ of <__main__.Vehicle object at 0x7f8af813a350>>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b9617e20>), ('__le__', <method-wrapper '__le__' of Vehicle object at 0x7f8af813a350>), ('__lt__', <method-wrapper '__lt__' of Vehicle object at 0x7f8af813a350>), ('__module__', '__main__'), ('__ne__', <method-wrapper '__ne__' of Vehicle object at 0x7f8af813a350>), ('__new__', <built-in method __new__ of type object at 0x55a6b6698ba0>), ('__reduce__', <built-in method __reduce__ of Vehicle object at 0x7f8af813a350>), ('__reduce_ex__', <built-in method __reduce_ex__ of Vehicle object at 0x7f8af813a350>), ('__repr__', <method-wrapper '__repr__' of Vehicle object at 0x7f8af813a350>), ('__setattr__', <method-wrapper '__setattr__' of Vehicle object at 0x7f8af813a350>), ('__sizeof__', <built-in method __sizeof__ of Vehicle object at 0x7f8af813a350>), ('__str__', <method-wrapper '__str__' of Vehicle object at 0x7f8af813a350>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b9617e20>), ('__weakref__', None), ('colour', 'red'), ('repaint', <bound method Vehicle.repaint of <__main__.Vehicle object at 0x7f8af813a350>>), ('wheels', 4)]
使用 hasattr()
方法查詢 Python 物件
最後,我們還可以使用 hasattr()
方法來找出 Python 物件的方法。此函式檢查物件是否具有屬性。
此方法的語法是:
# python 3.x
hasattr(object, attribute)
該函式有兩個引數:物件和屬性。它檢查該屬性是否存在於該特定物件中。
例如:
# python 3.x
print(hasattr(my_object,'__doc__'))
如果屬性存在,此函式將返回 True
。否則,它將返回 False
。此外,一旦我們找到了該方法,我們就可以使用 help()
函式來檢視其文件。
例如:
# python 3.x
help(object.method)
使用 getattr()
方法查詢物件
與 hasattr()
方法相反,getattr()
方法返回該特定 Python 物件存在的屬性內容。
這個函式的語法是:
# python 3.x
getattr(object,attribute)
例如:
# python 3.x
print(getattr(my_object,'__doc__'))
輸出:
Built-in mutable sequence.
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified.
從輸出中可以清楚地看出該屬性存在。因此,它返回了其內容以及有關此方法如何工作的詳細資訊。
到目前為止,我們已經研究了幾種執行物件內省的方法。換句話說,我們以 5 種不同的方式列出了 Python 物件的方法和屬性。
通過閱讀本文,我們應該能夠評估 Python 物件並進行自省。
如果本指南對你有幫助,請分享。