在 Python 中使用 fetchall() 從資料庫中提取元素
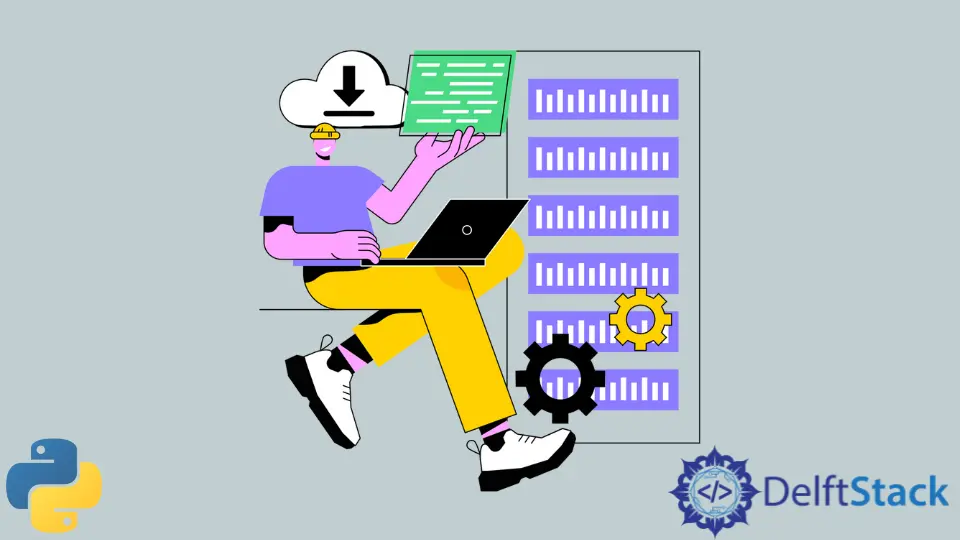
本文旨在介紹使用 fetchall()
從資料庫中提取元素的工作方法以及如何正確顯示它們。本文還將討論 list(cursor)
函式如何在程式中使用。
在 Python 中使用 fetchall()
從資料庫檔案中提取元素
該程式將與副檔名為 .db 的資料庫檔案建立安全 SQL 連線。建立連線後,程式將獲取儲存在該資料庫表中的資料。
由於它是一個使用 fetchall()
提取元素的程式,因此將使用 for
迴圈提取和顯示資料。
匯入 Sqlite3 並與資料庫建立連線
sqlite3 是 Python 中用於訪問資料庫的匯入包。它是一個內建包;它不需要安裝額外的軟體即可使用,可以使用 import sqlite3
直接匯入。
該程式在載入資料庫時使用 try
塊來測試錯誤,並在使用 exception
塊建立連線時丟擲錯誤訊息。最後,程式關閉與 finally
塊的連線。
但是,在瞭解如何使用 fetchall()
檢索專案之前,首先必須瞭解 SQLite 是如何建立連線的。該程式宣告瞭一個方法 allrowsfetched()
,在其中插入了 try
塊並宣告瞭一個變數 database_connecter
。
該變數將與資料庫建立連線並載入其內容,如下面的程式碼片段所示。
import sqlite3
def allrowsfetched():
try:
database = sqlite3.connect("samplefile.db")
cursorfordatabase = database.cursor()
print("Connection is established")
except Exception as e:
print(e)
建立連線後,需要為資料庫建立遊標,這是一種幫助使用 Python 為 SQL 資料庫執行命令的聯結器。
在上面的程式中,遊標是使用語法 database.cursor()
建立並儲存在變數 cursorfordatabase
中的。如果上述所有步驟都正確執行,程式將列印一條成功訊息。
為使用 fetchall()
方法建立遊標物件
要使用 fetchall()
提取元素,我們必須確定資料庫內容。程式中使用的資料庫中儲存了多個表。
該程式需要專門提取一個名為 employees
的表。它必須生成一個查詢:
- 使用語法
SELECT * from table_name
生成查詢。在程式中,查詢是為了從資料庫中找到一個名為employees
的表,它儲存在變數query_for_sqlite
中。 - 生成查詢後,
cursor.execute()
方法對資料庫執行該查詢。 - 最後,
cursor.fetchall()
語法使用fetchall()
提取元素,並將特定表載入到遊標內並將資料儲存在變數required_records
中。 - 變數
required_records
儲存整個表本身,因此返回該變數的長度提供表內的行數。 - 使用
len(required_records)
語法列印行數。
query_for_sqlite = """SELECT * from employees"""
cursorfordatabase.execute(query_for_sqlite)
required_records = cursorfordatabase.fetchall()
print("Rows Present in the database: ", len(required_records))
使用 for
迴圈顯示行元素
在使用 fetchall()
提取元素的步驟啟動後,程式使用 for
迴圈來列印元素。for
迴圈執行的次數是行在變數 required_records
中出現的次數。
在此內部,使用行的索引列印各個元素。在這個資料庫中,有 8 行(索引計數從 0 開始,到 7 結束)。
print("Data in an ordered list")
for row in required_records:
print("Id: ", row[0])
print("Last Name: ", row[1])
print("First Name ", row[2])
print("Title: ", row[3])
print("Reports to: ", row[4])
print("dob: ", row[5])
print("Hire-date: ", row[6])
print("Address: ", row[7])
print("\n")
處理異常
一旦實現了程式的目的,即使用 fetchall()
提取元素,則需要從記憶體中釋放遊標和連線變數中載入的資料。
- 首先,我們使用
cursor.close()
語法來釋放遊標變數cursorfordatabase
中儲存的記憶體。 - 然後程式需要說明異常處理,即程式的
except
和finally
塊,緊跟在try
塊之後。 except
塊用於 sqlite3 錯誤。因此,當未與資料庫建立連線時,程式會顯示錯誤訊息,而不是在執行時崩潰。finally
塊最後執行,在執行兩個塊中的一個後,try
或except
。它關閉 SQLite 連線並列印相關訊息。
finally
塊的執行發生在最後,無論哪個塊在它之前執行,為程式提供了一個關閉的姿態。
cursorfordatabase.close()
except sqlite3.Error as error:
print("Failed to read data from table", error)
finally:
if database:
database.close()
print("Connection closed")
用 Python 從資料庫檔案中提取元素的完整程式碼
下面提供了該程式的工作程式碼,以更好地理解這些概念。
import sqlite3
def allrowsfetched():
try:
database = sqlite3.connect("samplefile.db")
cursorfordatabase = database.cursor()
print("Connection established")
query_for_samplefile = """SELECT * from employees"""
cursorfordatabase.execute(query_for_samplefile)
required_records = cursorfordatabase.fetchall()
print("Rows Present in the database: ", len(required_records))
print("Data in an ordered list")
print(required_records)
for row in required_records:
print("Id: ", row[0])
print("Last Name: ", row[1])
print("First Name ", row[2])
print("Title: ", row[3])
print("Reports to: ", row[4])
print("dob: ", row[5])
print("Hired on: ", row[6])
print("Address: ", row[7])
print("\n")
cursorfordatabase.close()
except sqlite3.Error as error:
print("Failed to read data from table,", error)
finally:
if database:
database.close()
print("The Sqlite connection is closed")
allrowsfetched()
輸出:當成功找到表時,
"C:/Users/Win 10/main.py"
Connection established
Rows Present in the database: 8
Data in an ordered list
Id: 1
Last Name: Adams
First Name Andrew
Title: General Manager
Reports to: None
Birthdate: 1962-02-18 00:00:00
Hire-date: 2002-08-14 00:00:00
Address: 11120 Jasper Ave NW
.
.
.
Connection closed
Process finished with exit code 0
輸出:當所需的表不存在時,
"C:/Users/Win 10/main.py"
Connection established
Failed to read data from table, no such table: salary
Connection closed
Process finished with exit code 0
在這裡,錯誤是通過使用表名 salary
作為查詢建立的,例如 query_for_samplefile = """SELECT * from salary"""
。
在 Python 中使用 list(cursor)
作為從資料庫中提取元素的替代方法
使用 fetchall()
提取元素的方法已經討論到現在,儘管還有其他方法,例如 fetchone()
和 fetchmany()
。
我們也可以在不使用 fetch()
方法的情況下提取元素;相反,我們可以使用 list(cursor)
。這個過程就像 fetchall()
一樣提取所有元素。
該方法節省了記憶體佔用。與載入整個表的 fetchall()
不同,list(cursor)
執行一個迴圈,連續提取元素,然後從資料庫中列印它們而不將它們儲存在任何地方。
下面的程式碼讓我們瞭解如何使用它。
所有步驟都類似於上面的程式,除了沒有使用 fetchall()
初始化新變數來儲存表。遊標 cursorfordatabase
被放入 for
迴圈中,並列印該行。
由於遊標物件僅儲存查詢,因此在記憶體佔用中佔用的空間最少甚至沒有。
query_for_sqlite = """SELECT * from employees"""
cursorfordatabase.execute(query_for_sqlite)
for row in cursorfordatabase:
print("\n", row)
索引還可以獲取有序列表,就像上一個程式一樣。
for row in cursorfordatabase:
print("id:", row[0])
print("l_name:", row[1])
まとめ
本文重點展示如何在 Python 程式中使用 fetchall()
提取元素。你已經學習了諸如 cursor()
之類的概念和諸如 cursor.execute()
、sqlite3.connect
之類的語法函式,以及處理異常塊。
你還了解了 list(cursor)
方法以及它如何成為從資料庫中提取元素的替代方法。