Python 中的餘弦相似度
Shivam Arora
2023年1月30日
-
使用
scipy
模組計算 Python 中兩個列表之間的餘弦相似度 -
使用
NumPy
模組計算 Python 中兩個列表之間的餘弦相似度 -
使用
sklearn
模組計算 Python 中兩個列表之間的餘弦相似度 -
使用
torch
模組計算 Python 中兩個列表之間的餘弦相似度
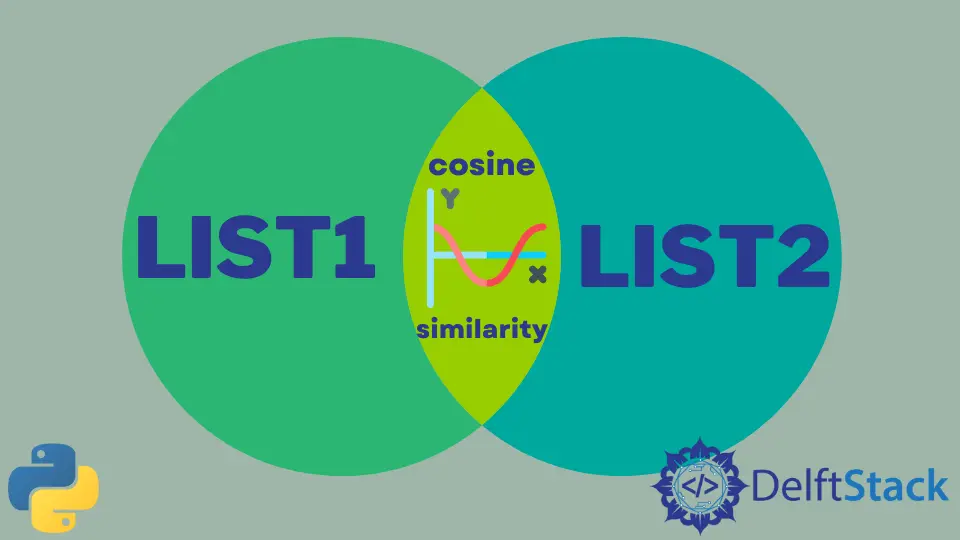
餘弦相似度通過計算兩個向量列表之間的餘弦角來衡量向量列表之間的相似度。如果考慮餘弦函式,它在 0 度時的值為 1,在 180 度時為 -1。這意味著對於兩個重疊的向量,對於兩個完全相反的向量,餘弦值將是最大值和最小值。
在本文中,我們將計算兩個大小相等的列表之間的餘弦相似度。
使用 scipy
模組計算 Python 中兩個列表之間的餘弦相似度
來自 scipy
模組的 spatial.cosine.distance()
函式計算距離而不是餘弦相似度,但為了實現這一點,我們可以從 1 中減去距離的值。
例如,
from scipy import spatial
List1 = [4, 47, 8, 3]
List2 = [3, 52, 12, 16]
result = 1 - spatial.distance.cosine(List1, List2)
print(result)
輸出:
0.9720951480078084
使用 NumPy
模組計算 Python 中兩個列表之間的餘弦相似度
numpy.dot()
函式計算作為引數傳遞的兩個向量的點積。numpy.norm()
函式返回向量範數。
我們可以使用這些函式和正確的公式來計算餘弦相似度。
例如,
from numpy import dot
from numpy.linalg import norm
List1 = [4, 47, 8, 3]
List2 = [3, 52, 12, 16]
result = dot(List1, List2) / (norm(List1) * norm(List2))
print(result)
輸出:
0.9720951480078084
如果有多個或一組向量和一個查詢向量來計算餘弦相似度,我們可以使用以下程式碼。
import numpy as np
List1 = np.array([[4, 45, 8, 4], [2, 23, 6, 4]])
List2 = np.array([2, 54, 13, 15])
similarity_scores = List1.dot(List2) / (
np.linalg.norm(List1, axis=1) * np.linalg.norm(List2)
)
print(similarity_scores)
輸出:
[0.98143311 0.99398975]
使用 sklearn
模組計算 Python 中兩個列表之間的餘弦相似度
在 sklearn
模組中,有一個名為 cosine_similarity()
的內建函式來計算餘弦相似度。
請參考下面的程式碼。
from sklearn.metrics.pairwise import cosine_similarity, cosine_distances
A = np.array([10, 3])
B = np.array([8, 7])
result = cosine_similarity(A.reshape(1, -1), B.reshape(1, -1))
print(result)
輸出:
[[0.91005765]]
使用 torch
模組計算 Python 中兩個列表之間的餘弦相似度
當我們處理具有形狀 (m,n) 的 N 維張量時,我們可以使用 torch
模組中的 consine_similarity()
函式來查詢餘弦相似度。
例如,
import torch
import torch.nn.functional as F
t1 = [3, 45, 6, 8]
a = torch.FloatTensor(t1)
t2 = [4, 54, 3, 7]
b = torch.FloatTensor(t2)
result = F.cosine_similarity(a, b, dim=0)
print(result)
輸出:
tensor(0.9960)
使用 torch.FloatTensor()
模組將列表轉換為張量。