如何在 Tkinter 中建立全屏視窗
-
Windows
root.attributes('-fullscreen', True)
在 Tkinter 中建立全屏模式 -
在 Tkinter 中 Ubuntu
root.attributes('-zoomed', True)
建立全屏模式 - 顯示帶有工具欄的全屏模式
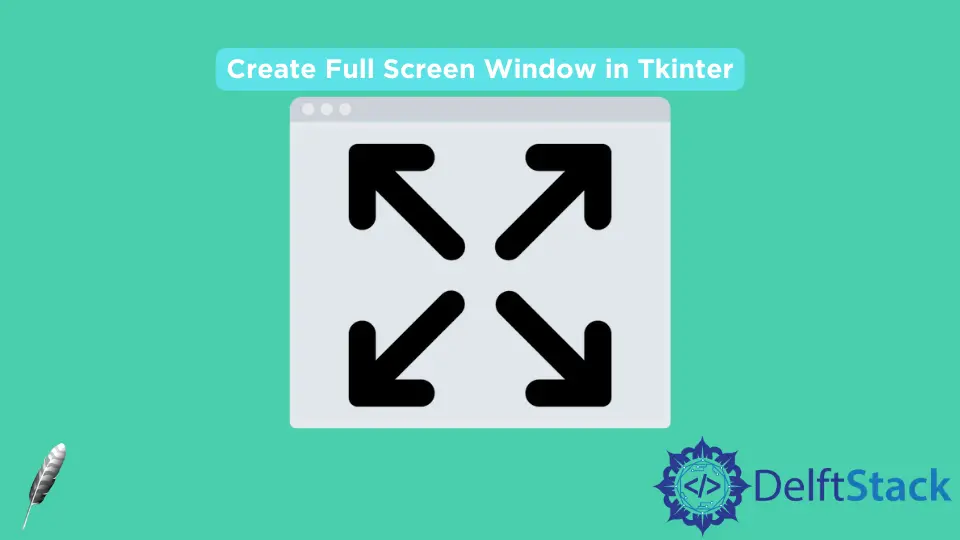
在本教程中,我們將介紹如何在 Tkinter 中建立全屏視窗,以及如何切換或退出全屏模式。
Windows root.attributes('-fullscreen', True)
在 Tkinter 中建立全屏模式
tk.Tk.attributes
設定特定作業系統平臺的屬性。Windows 中的屬性是
-alpha
-transparentcolor
-disabled
-fullscreen
-toolwindow
-topmost
-fullscreen
指定視窗是否為全屏模式。
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-fullscreen", True)
self.fullScreenState = False
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
if __name__ == "__main__":
app = Fullscreen_Example()
self.window.bind("<F11>", self.toggleFullScreen)
繫結 F11 到 toggleFullScreen
函式。
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
在此函式中,fullscreen
模式被更新為跟前一狀態相反的狀態。
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
quitFullScreen
函式通過設定 -fullscreen
為 False
而退出全屏模式。
我們可以使用 lambda
函式來簡化解決方案。
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-fullscreen", True)
self.window.bind(
"<F11>",
lambda event: self.window.attributes(
"-fullscreen", not self.window.attributes("-fullscreen")
),
)
self.window.bind(
"<Escape>", lambda event: self.window.attributes("-fullscreen", False)
)
self.window.mainloop()
if __name__ == "__main__":
app = Fullscreen_Example()
self.window.bind(
"<F11>",
lambda event: self.window.attributes(
"-fullscreen", not self.window.attributes("-fullscreen")
),
)
它將 lambda
函式繫結到 F11,可以在其中讀取當前的全屏狀態 self.window.attributes("-fullscreen")
,如果該方法中未指定任何值,則該方法返回 -fullscreen
的當前狀態。
如果遵循這種方法,我們就不再需要 fullScreenState
狀態變數。
在 Tkinter 中 Ubuntu root.attributes('-zoomed', True)
建立全屏模式
-fullscreen
屬性僅在 Windows 上存在,而在 Linux 或 macOS 上並不存在。Ubuntu 具有類似的屬性 -zoomed
,可將視窗設定為全屏模式。
import tkinter as tk
class Ubuntu_Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-zoomed", True)
self.fullScreenState = False
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-zoomed", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-zoomed", self.fullScreenState)
if __name__ == "__main__":
app = Ubuntu_Fullscreen_Example()
顯示帶有工具欄的全屏模式
上面程式碼中顯示的全屏模式中,工具欄是不可見的。如果需要在視窗中顯示工具欄,則視窗的幾何形狀應與顯示器的尺寸相同。
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
self.w, self.h = (
self.window.winfo_screenwidth(),
self.window.winfo_screenheight(),
)
self.window.geometry("%dx%d" % (self.w, self.h))
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
if __name__ == "__main__":
app = Fullscreen_Example()
self.w, self.h = self.window.winfo_screenwidth(), self.window.winfo_screenheight()
winfo_screenwidth()
和 winfo_screenheight()
獲得顯示器的寬度和高度。
self.window.geometry("%dx%d" % (self.w, self.h))
使用 geometry
方法將 GUI 視窗大小設定為與顯示器的寬度和高度相同。
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn