在 Pandas 中對 DataFrame 進行列切片
Manav Narula
2023年1月30日
-
使用
loc()
對 Pandas DataFrame 中的列切片 -
使用
iloc()
在 Pandas DataFrame 中列切片 -
使用
redindex()
在 Pandas DataFrame 中切列片
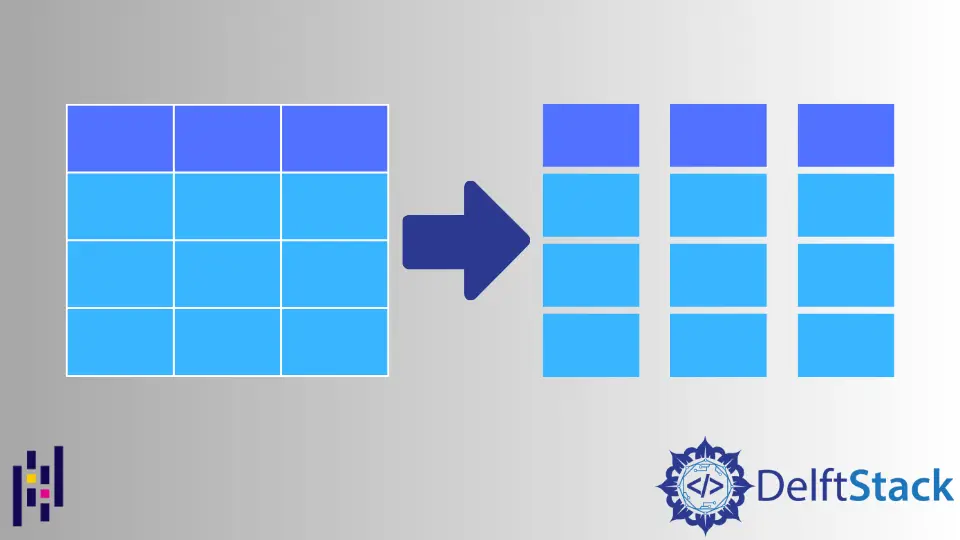
Pandas 中的列式切片允許我們將 DataFrame 切成子集,這意味著它從原來的 DataFrame 中建立一個新的 Pandas DataFrame,其中只包含所需的列。我們將以下面的 DataFrame 為例來進列切片操作。
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
print(df)
輸出:
a b c d
0 0.797321 0.468894 0.335781 0.956516
1 0.546303 0.567301 0.955228 0.557812
2 0.385315 0.706735 0.058784 0.578468
3 0.751037 0.248284 0.172229 0.493763
使用 loc()
對 Pandas DataFrame 中的列切片
Pandas 庫為我們提供了一種以上的方法來進行列式切片。第一種是使用 loc()
函式。
Pandas 的 loc()
函式允許我們使用列名或索引標籤來訪問 DataFrame 的元素。使用 loc()
進行列切片的語法:
dataframe.loc[:, [columns]]
例子:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
df1 = df.loc[:, "a":"c"] # Returns a new dataframe with columns a,b and c
print(df1)
輸出:
a b c
0 0.344952 0.611792 0.213331
1 0.907322 0.992097 0.080447
2 0.471611 0.625846 0.348778
3 0.656921 0.999646 0.976743
使用 iloc()
在 Pandas DataFrame 中列切片
我們也可以使用 iloc()
函式來使用行和列的整數索引來訪問 DataFrame 的元素。使用 iloc()
對列進行切片的語法。
dataframe.iloc[:, [column - index]]
例:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
df1 = df.iloc[:, 0:2] # Returns a new dataframe with first two columns
print(df1)
輸出:
a b
0 0.034587 0.070249
1 0.648231 0.721517
2 0.485168 0.548045
3 0.377612 0.310408
使用 redindex()
在 Pandas DataFrame 中切列片
reindex()
函式也可用於改變 DataFrame 的索引,並可用於列的切片。reindex()
函式可以接受許多引數,但對於列的分割,我們只需要向函式提供列名。
使用 reindex()
進行列切片的語法:
dataframe.reindex(columns=[column_names])
例:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(4, 4), columns=["a", "b", "c", "d"])
# Returns a new dataframe with c and b columns
df1 = df.reindex(columns=["c", "b"])
print(df1)
輸出:
c b
0 0.429790 0.962838
1 0.605381 0.463617
2 0.922489 0.733338
3 0.741352 0.118478
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn