Pandas DataFrame 選擇列
Suraj Joshi
2023年1月30日
2021年1月22日
- 使用索引操作從 Pandas DataFrame 中選擇列
-
使用
DataFrame.drop()
方法從 Pandas DataFrame 中選擇列 -
使用
DataFrame.filter()
方法從 Pandas DataFrame 中選擇列
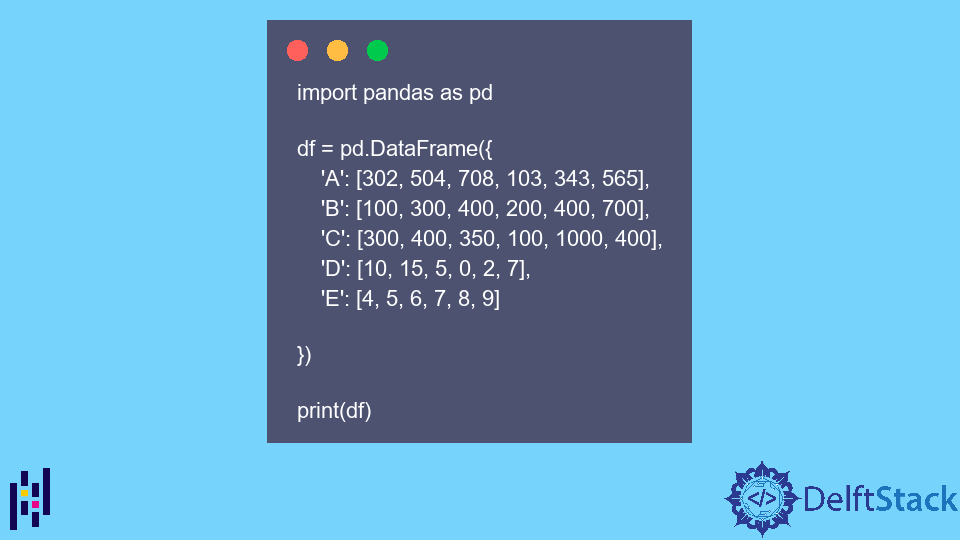
本教程介紹瞭如何通過索引或使用 DataFrame.drop()
和 DataFrame.filter()
方法從 Pandas DataFrame 中選擇列。
我們將使用下面的 DataFrame df
來解釋如何從 Pandas DataFrame 中選擇列。
import pandas as pd
df = pd.DataFrame({
'A': [302, 504, 708, 103, 343, 565],
'B': [100, 300, 400, 200, 400, 700],
'C': [300, 400, 350, 100, 1000, 400],
'D': [10, 15, 5, 0, 2, 7],
'E': [4, 5, 6, 7, 8, 9]
})
print(df)
輸出:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
使用索引操作從 Pandas DataFrame 中選擇列
import pandas as pd
df = pd.DataFrame({
'A': [302, 504, 708, 103, 343, 565],
'B': [100, 300, 400, 200, 400, 700],
'C': [300, 400, 350, 100, 1000, 400],
'D': [10, 15, 5, 0, 2, 7],
'E': [4, 5, 6, 7, 8, 9]
})
derived_df = df[['A', 'C', 'E']]
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
輸出:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
它從 DataFrame df
中選擇列 A
、C
和 E
,並將這些列分配到 derived_df
DataFrame 中。
使用 DataFrame.drop()
方法從 Pandas DataFrame 中選擇列
import pandas as pd
df = pd.DataFrame({
'A': [302, 504, 708, 103, 343, 565],
'B': [100, 300, 400, 200, 400, 700],
'C': [300, 400, 350, 100, 1000, 400],
'D': [10, 15, 5, 0, 2, 7],
'E': [4, 5, 6, 7, 8, 9]
})
derived_df = df.drop(['B', 'D'], axis=1)
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
輸出:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
它從 DataFrame df
中刪除 B
和 D
列,並將其餘列分配給 derived_df
。或者,它選擇除 B
和 D
以外的所有列,並將它們分配到 derived_df
DataFrame 中。
使用 DataFrame.filter()
方法從 Pandas DataFrame 中選擇列
import pandas as pd
df = pd.DataFrame({
'A': [302, 504, 708, 103, 343, 565],
'B': [100, 300, 400, 200, 400, 700],
'C': [300, 400, 350, 100, 1000, 400],
'D': [10, 15, 5, 0, 2, 7],
'E': [4, 5, 6, 7, 8, 9]
})
derived_df = df.filter(["A", "C", "E"])
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
輸出:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
它從 DataFrame df
中提取或過濾 A
、C
和 E
列,並將其分配給 DataFrame derived_df
。
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn