Pandas DataFrame 基於其他列建立新列
Suraj Joshi
2023年1月30日
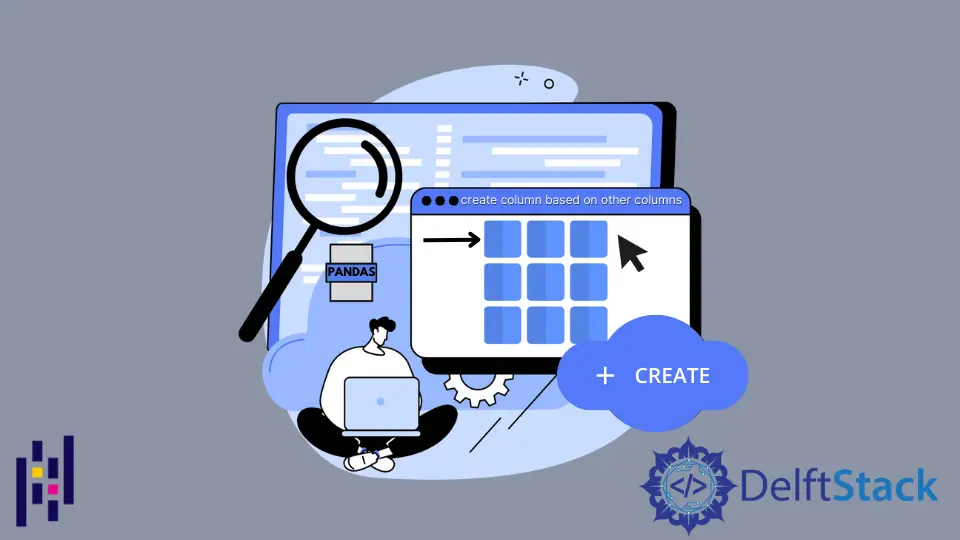
本教程將介紹我們如何在 Pandas DataFrame 中根據 DataFrame 中其他列的值,通過對列的每個元素應用函式或使用 DataFrame.apply()
方法來建立新的列。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print(items_df)
輸出:
Id Name Cost Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
我們將使用上面程式碼片段中顯示的 DataFrame 來演示如何根據 DataFrame 中其他列的值在 Pandas DataFrame 中建立新的列。
Pandas DataFrame 中根據其他列的值按元素操作建立新列
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual Price": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df["Actual Price"] - (
(items_df["Discount(%)"] / 100) * items_df["Actual Price"]
)
print("DataFrame after addition of new column")
print(items_df, "\n")
輸出:
Initial DataFrame:
Id Name Actual Price Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual Price Discount(%) Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
它通過從 DataFrame 的 Actual Price
一欄中減去折扣額的價值來計算每個產品的最終價格。然後將最終價格值的 Series
分配到 DataFrame items_df
的 Final Price
列。
使用 DataFrame.apply()
方法在 Pandas DataFrame 中根據其他列的值建立新列
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual_Price": [300, 400, 350, 100, 1000, 400],
"Discount_Percentage": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df.apply(
lambda row: row.Actual_Price - ((row.Discount_Percentage / 100) * row.Actual_Price),
axis=1,
)
print("DataFrame after addition of new column")
print(items_df, "\n")
輸出:
Initial DataFrame:
Id Name Actual_Price Discount_Percentage
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual_Price Discount_Percentage Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
它將 apply()
方法中定義的 lambda 函式應用於 DataFrame items_df
的每一行,最後將一系列結果分配到 DataFrame items_df
的 Final Price
列。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn