Pandas 將字串轉換為數字型別
Suraj Joshi
2023年1月30日
-
pandas.to_numeric()
方法 -
使用
pandas.to_numeric()
方法將 Pandas DataFrame 的字串值轉換為數字型別 - 將 Pandas DataFrame 中的字串值轉換為含有其他字元的數字型別
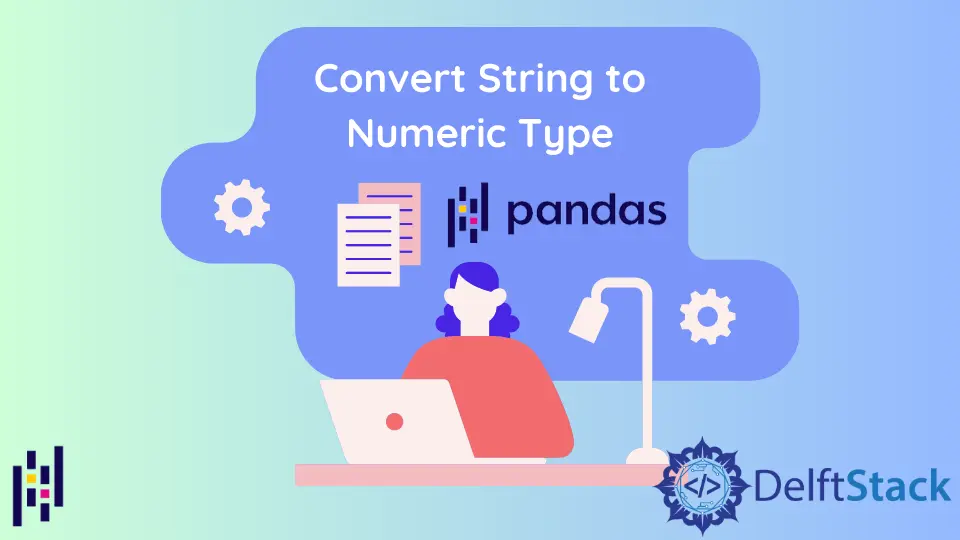
本教程解釋瞭如何使用 pandas.to_numeric()
方法將 Pandas DataFrame 的字串值轉換為數字型別。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print(items_df)
輸出:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
我們將用上面的例子來演示如何將 DataFrame 的值轉換為數字型別。
pandas.to_numeric()
方法
語法
pandas.to_numeric(arg, errors="raise", downcast=None)
它將作為 arg
傳遞的引數轉換為數字型別。預設情況下,arg
將被轉換為 int64
或 float64
。我們可以設定 downcast
引數的值,將 arg
轉換為其他資料型別。
使用 pandas.to_numeric()
方法將 Pandas DataFrame 的字串值轉換為數字型別
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"])
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes)
輸出:
The items DataFrame is:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
它將 items_df
中 Cost
列的資料型別從 object
轉換為 int64
。
將 Pandas DataFrame 中的字串值轉換為含有其他字元的數字型別
如果我們想將一列轉換成數值型別,其中有一些字元的值,我們得到一個錯誤說 ValueError: Unable to parse string
。在這種情況下,我們可以刪除所有非數字字元,然後進行型別轉換。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["$300", "$400", "$350", "$100", "$1000", "$400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"].str.replace("$", ""))
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes, "\n")
print("DataFrame after Type Conversion:")
print(items_df)
輸出:
The items DataFrame is:
Id Name Cost
0 302 Watch $300
1 504 Camera $400
2 708 Phone $350
3 103 Shoes $100
4 343 Laptop $1000
5 565 Bed $400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
DataFrame after Type Conversion:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
它刪除了與 Cost
列的值相連的 $
字元,然後使用 pandas.to_numeric()
方法將這些值轉換為數字型別。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn