在 Pandas 中載入 JSON 檔案
- 將 JSON 檔案載入到 Pandas DataFrame 中
- 將面向索引的 JSON 檔案載入到 Pandas DataFrame 中
- 將面向列的 JSON 檔案載入到 Pandas DataFrame 中
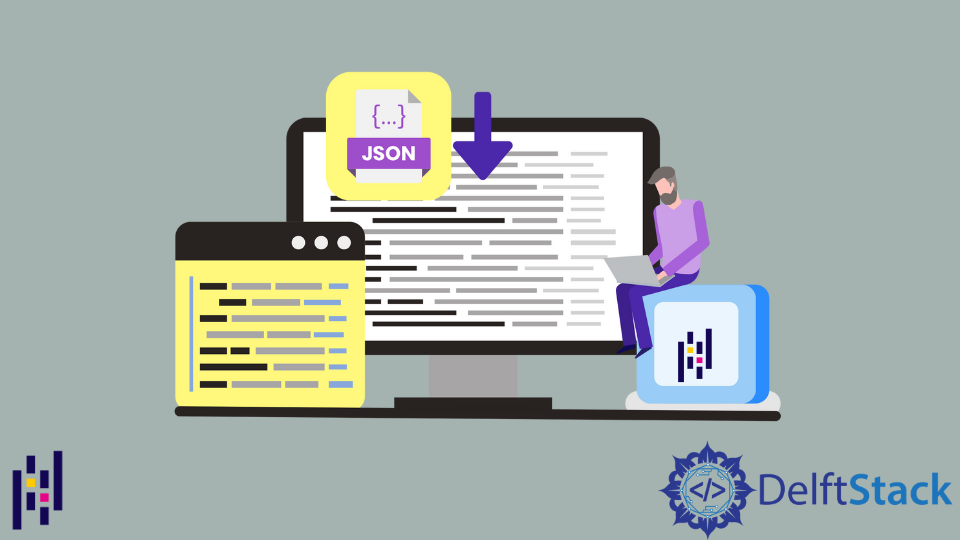
本教程介紹瞭如何使用 pandas.read_json()
方法將一個 JSON 檔案載入到 Pandas DataFrame 中。
將 JSON 檔案載入到 Pandas DataFrame 中
我們可以使用 pandas.read_json()
函式將 JSON 檔案的路徑作為引數傳遞給 pandas.read_json()
函式,將 JSON 檔案載入到 Pandas DataFrame 中。
{
"Name": {
"1": "Anil",
"2": "Biraj",
"3": "Apil",
"4": "Kapil"
},
"Age": {
"1": 23,
"2": 25,
"3": 28,
"4": 30
}
}
示例 data.json
檔案的內容如上所示。我們將從上述 JSON 檔案中建立一個 DataFrame。
import pandas as pd
df=pd.read_json("data.json")
print("DataFrame generated using JSON file:")
print(df)
輸出:
DataFrame generated using JSON file:
Name Age
1 Anil 23
2 Biraj 25
3 Apil 28
4 Kapil 30
它顯示的是由 data.json
檔案中的資料生成的 DataFrame。我們必須確保在當前工作目錄下有 data.json
檔案才能生成 DataFrame,否則我們需要提供 JSON 檔案的完整路徑作為 pandas.read_json()
方法的引數。
由 JSON 檔案形成的 DataFrame 取決於 JSON 檔案的方向。我們一般有三種不同的 JSON 檔案的面向。
- 面向索引
- 面向值
- 面向列
將面向索引的 JSON 檔案載入到 Pandas DataFrame 中
{
"0": {
"Name": "Anil",
"Age": 23
},
"1": {
"Name": "Biraj",
"Age": 25
},
"2": {
"Name": "Apil",
"Age": 26
}
}
這是一個面向索引的 JSON 檔案的例子,其中頂層鍵代表資料的索引。
import pandas as pd
df=pd.read_json("data.json")
print("DataFrame generated from Index Oriented JSON file:")
print(df)
輸出:
DataFrame generated from Index Oriented JSON file:
0 1 2
Name Anil Biraj Apil
Age 23 25 26
它將從 data.json
檔案中建立一個 DataFrame,頂層鍵在 DataFrame 中表示為列。
將面向值的 JSON 檔案載入到 Pandas DataFrame 中
[
["Anil", 23],
["Biraj", 25],
["Apil", 26]
]
這是一個面向值的 JSON 檔案的例子,陣列中的每個元素代表每一行的值。
import pandas as pd
df=pd.read_json("data.json")
print("DataFrame generated from Value Oriented JSON file:")
print(df)
輸出:
DataFrame generated from Value Oriented JSON file:
0 1
0 Anil 23
1 Biraj 25
2 Apil 26
它將從 data.json
檔案中建立一個 DataFrame,JSON 檔案中陣列的每個元素將在 DataFrame 中表示為一行。
將面向列的 JSON 檔案載入到 Pandas DataFrame 中
{
"Name": {
"1": "Anil",
"2": "Biraj",
"3": "Apil"
},
"Age": {
"1": 23,
"2": 25,
"3": 28
}
}
它是一個面向列的 JSON 檔案頂層索引的例子,代表資料的列名。
import pandas as pd
df=pd.read_json("data.json")
print("DataFrame generated from Column Oriented JSON file:")
print(df)
輸出:
DataFrame generated from Column Oriented JSON file:
Name Age
1 Anil 23
2 Biraj 25
3 Apil 28
它將從 data.json
檔案中建立一個 DataFrame,JSON 檔案的頂層索引將作為 DataFrame 中的列名。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn