獲取 Dataframe Pandas 的第一行
Suraj Joshi
2023年1月30日
2021年1月22日
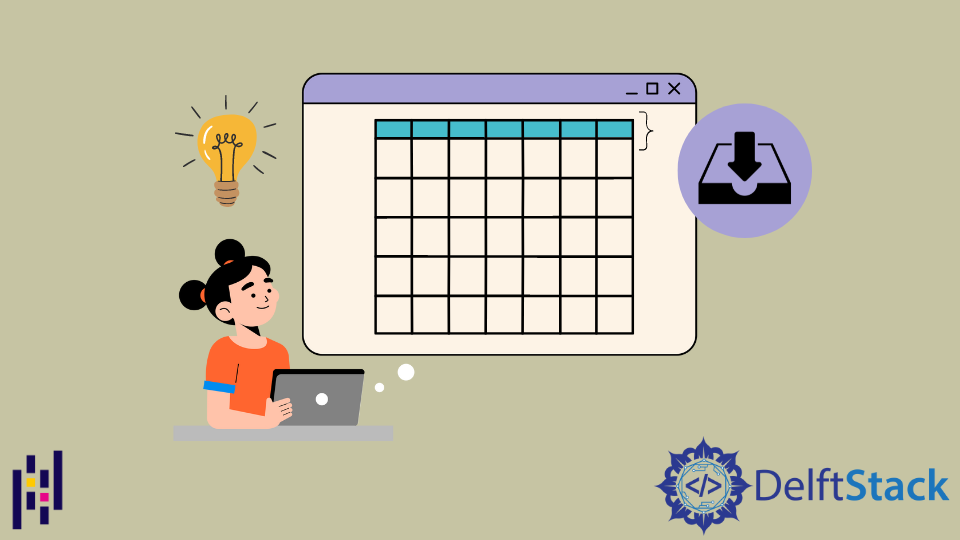
本教程介紹瞭如何使用 pandas.DataFrame.iloc
屬性和 pandas.DataFrame.head()
方法從 Pandas DataFrame 中獲取第一行。
我們將在下面的例子中使用以下 DataFrame 來解釋如何從 Pandas DataFrame 中獲取第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
print(df)
輸出:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
使用 pandas.DataFrame.iloc
屬性獲取 Pandas DataFrame 的第一行
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
row_1=df.iloc[0]
print("The DataFrame is:")
print(df,"\n")
print("The First Row of the DataFrame is:")
print(row_1)
輸出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 A
C_2 40
C_3 430
Name: 0, dtype: object
它顯示 DataFrame df
的第一行。為了選擇第一行,我們使用第一行的預設索引,即 0
和 DataFrame 的 iloc
屬性。
使用 pandas.DataFrame.head()
方法從 Pandas DataFrame 中獲取第一行
pandas.DataFrame.head()
方法返回一個 DataFrame,其中包含 DataFrame 中最上面的 5 行。我們也可以傳遞一個數字作為引數給 pandas.DataFrame.head()
方法,代表要選擇的最上面的行數。我們可以傳遞 1 作為引數到 pandas.DataFrame.head()
方法中,只選擇 DataFrame 的第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
row_1=df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The First Row of the DataFrame is:")
print(row_1)
輸出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 C_2 C_3
0 A 40 430
根據指定的條件從 Pandas DataFrame 中獲取第一行
為了從 DataFrame 中提取滿足指定條件的第一行,我們首先過濾滿足指定條件的行,然後使用上面討論的方法從過濾後的 DataFrame 中選擇第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 500, 350],
})
filtered_df=df[(df.C_2 < 40) & (df.C_3 > 450)]
row_1_filtered=filtered_df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The Filtered DataFrame is:")
print(filtered_df,"\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
輸出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
它將顯示第一條列 C_2
值小於 45 且 C_3
列值大於 450 的行。
我們也可以使用 query()
方法來過濾 DataFrame 中的行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 500, 350],
})
filtered_df=df.query('(C_2 < 40) & (C_3 > 450)')
row_1_filtered=filtered_df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The Filtered DataFrame is:")
print(filtered_df,"\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
輸出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
它將使用 query()
方法過濾所有列 C_2
值小於 45 和列 C_3
值大於 450 的行,然後使用 head()
方法從 filtered_df
中選擇第一行。
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn