將 Pandas DataFrame 匯出到 Excel 檔案
-
使用
to_excel()
函式將 PandasDataFrame
匯出到 Excel 檔案中 -
使用
ExcelWriter()
方法匯出 PandasDataFrame
-
將多個 Pandas
dataframes
匯出到多個 Excel 表格中
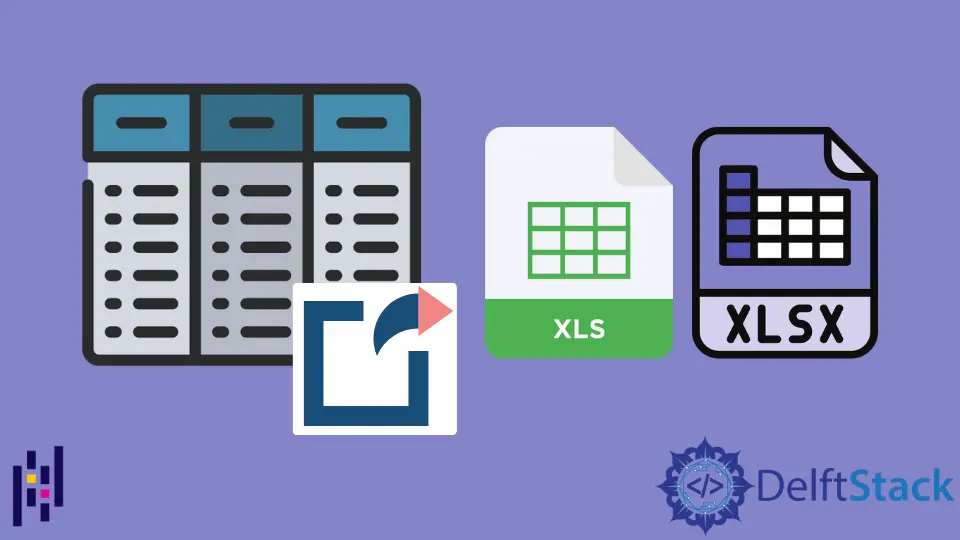
我們將在本教程中演示如何使用兩種不同的方式將 Pandas DataFrame
匯出到 excel 檔案。第一種方法是通過使用檔名呼叫 to_excel()
函式,將 Pandas DataFrame
匯出到 excel 檔案。本文中討論的另一種方法是 ExcelWriter()
方法。此方法將物件寫入 Excel 工作表,然後使用 to_excel
函式將它們匯出到 Excel 檔案中。
在本指南中,我們還將討論如何使用 ExcelWriter()
方法將多個 Pandas dataframes
新增到多個 Excel 工作表中。此外,我們在我們的系統上執行了多個示例來詳細解釋每種方法。
使用 to_excel()
函式將 Pandas DataFrame
匯出到 Excel 檔案中
當我們使用 dataframe.to_excel()
函式將 pandas DataFrame
匯出到 excel 表時,它會直接將一個物件寫入 excel 表中。要實現此方法,請建立一個 DataFrame
,然後指定 excel 檔案的名稱。現在,通過使用 dataframe.to_excel()
函式,將 Pandas DataFrame
匯出到 excel 檔案中。
在以下示例中,我們建立了一個名為 sales_record
的 DataFrame
,其中包含 Products_ID
、Product_Names
、Product_Prices
、Product_Sales
列。之後,我們為 excel 檔案 ProductSales_sheet.xlsx
指定了名稱。我們使用 sales_record.to_excel()
方法將所有資料儲存到 excel 表中。
請參閱下面的示例程式碼:
import pandas as pd
# DataFrame Creation
sales_record = pd.DataFrame(
{
"Products_ID": {
0: 101,
1: 102,
2: 103,
3: 104,
4: 105,
5: 106,
6: 107,
7: 108,
8: 109,
},
"Product_Names": {
0: "Mosuse",
1: "Keyboard",
2: "Headphones",
3: "CPU",
4: "Flash Drives",
5: "Tablets",
6: "Android Box",
7: "LCD",
8: "OTG Cables",
},
"Product_Prices": {
0: 700,
1: 800,
2: 200,
3: 2000,
4: 100,
5: 1500,
6: 1800,
7: 1300,
8: 90,
},
"Product_Sales": {0: 5, 1: 13, 2: 50, 3: 4, 4: 100, 5: 50, 6: 6, 7: 1, 8: 50},
}
)
# Specify the name of the excel file
file_name = "ProductSales_sheet.xlsx"
# saving the excelsheet
sales_record.to_excel(file_name)
print("Sales record successfully exported into Excel File")
輸出:
Sales record successfully exported into Excel File
執行上述原始碼後,excel 檔案 ProductSales_sheet.xlsx
將儲存在當前執行專案的資料夾中。
使用 ExcelWriter()
方法匯出 Pandas DataFrame
Excelwrite()
方法也可用於將 Pandas DataFrame
匯出到 excel 檔案中。首先,我們使用 Excewriter()
方法將物件寫入 excel 表,然後使用 dataframe.to_excel()
函式,我們可以將 DataFrame
匯出到 excel 檔案中。
請參閱下面的示例程式碼。
import pandas as pd
students_data = pd.DataFrame(
{
"Student": ["Samreena", "Ali", "Sara", "Amna", "Eva"],
"marks": [800, 830, 740, 910, 1090],
"Grades": ["B+", "B+", "B", "A", "A+"],
}
)
# writing to Excel
student_result = pd.ExcelWriter("StudentResult.xlsx")
# write students data to excel
students_data.to_excel(student_result)
# save the students result excel
student_result.save()
print("Students data is successfully written into Excel File")
輸出:
Students data is successfully written into Excel File
將多個 Pandas dataframes
匯出到多個 Excel 表格中
在上述方法中,我們將單個 Pandas DataFrame
匯出到 Excel 表格中。但是,使用這種方法,我們可以將多個 Pandas dataframes
匯出到多個 Excel 表格中。
請參閱以下示例,其中我們將多個 DataFrame
分別匯出到多個 Excel 工作表中:
import pandas as pd
import numpy as np
import xlsxwriter
# Creating records or dataset using dictionary
Science_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"science": ["88", "60", "66", "94", "40"],
}
Computer_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"computer_science": ["73", "63", "50", "95", "73"],
}
Art_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"fine_arts": ["95", "63", "50", "60", "93"],
}
# Dictionary to Dataframe conversion
dataframe1 = pd.DataFrame(Science_subject)
dataframe2 = pd.DataFrame(Computer_subject)
dataframe3 = pd.DataFrame(Art_subject)
with pd.ExcelWriter("studentsresult.xlsx", engine="xlsxwriter") as writer:
dataframe1.to_excel(writer, sheet_name="Science")
dataframe2.to_excel(writer, sheet_name="Computer")
dataframe3.to_excel(writer, sheet_name="Arts")
print("Please check out subject-wise studentsresult.xlsx file.")
輸出:
Please check out subject-wise studentsresult.xlsx file.