在 PHP 中建立和使用靜態類
Kevin Amayi
2023年1月30日
2022年5月13日
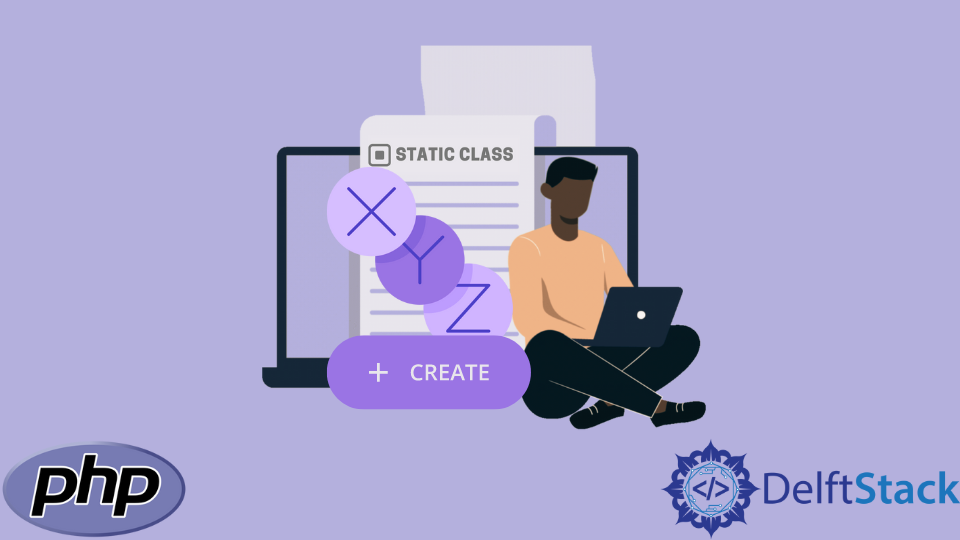
在 PHP 中,靜態類是在程式中只例項化一次的類。它必須有一個靜態成員(variable
)、靜態成員函式(method
),或兩者兼有。
在 PHP 中使用靜態變數建立靜態類
讓我們建立一個類
並初始化四個
變數。然後我們將使用類名訪問它們。
<?php
class Person {
//intialize static variable
public static $name = "Kevin";
public static $age = 23;
public static $hobby = "soccer";
public static $books = "HistoryBooks";
}
// print the static variables
echo Person::$name .'<br>';
echo Person::$age .'<br>';
echo Person::$hobby .'<br>';
echo Person::$books .'<br>';
?>
輸出:
Kevin
23
soccer
HistoryBooks
在 PHP 中使用靜態方法建立靜態類
我們將初始化一個名為 simpleProfile()
的方法。然後使用類名呼叫該方法。
<?php
class Person {
public static function simpleProfile() {
echo "I am a programmer and loves reading historical books.";
}
}
// Call static method
Person::simpleProfile();
?>
輸出:
I am a programmer and loves reading historical books.
在 PHP 中建立靜態類並在另一個類上呼叫靜態方法
我們使用 class name
在另一個類上呼叫 swahiliMan()
方法。
<?php
class greetings {
public static function swahiliGreeting() {
echo "Habari Yako!";
}
}
class swahiliMan {
public function greetings() {
greetings::swahiliGreeting();
}
}
$swahiliman1 = new swahiliMan();
$swahiliman1->greetings();
?>
輸出:
Habari Yako!
使用 parent
關鍵字建立靜態類並在子類上呼叫靜態方法
我們用靜態變數例項化一個方法。之後,使用 parent
關鍵字呼叫該方法並將其分配給擴充套件靜態類的子類上的變數。
class Person {
protected static function getCountry() {
return "Australia";
}
}
// a child class, extends the Person class
class Student extends Person {
public $country;
public function __construct() {
$this->country = parent::getCountry();
}
}
$student = new Student;
echo $student -> country;
?>
輸出:
Australia