在 PHP 中解析 HTML
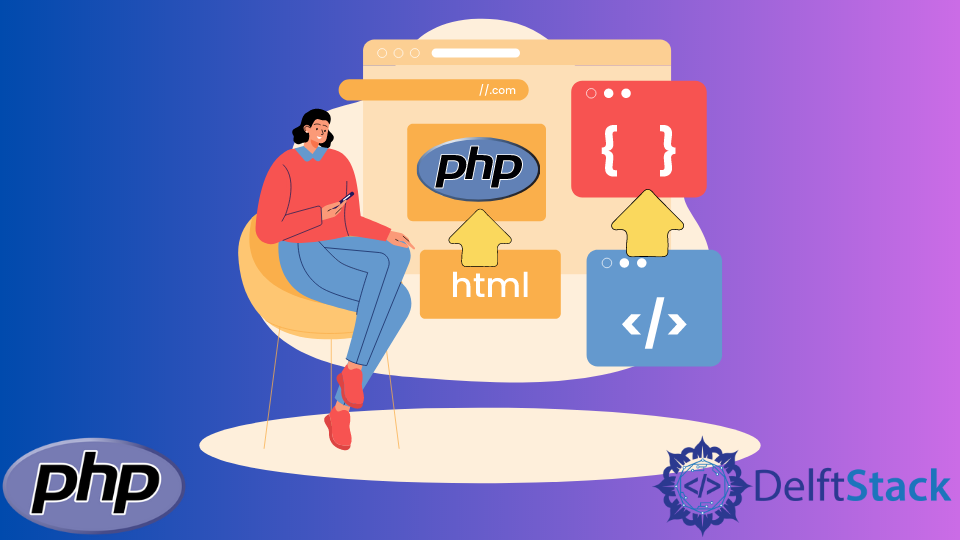
解析 HTML 允許我們將其內容或標記轉換為字串,從而更容易分析或建立動態 HTML 檔案。更詳細地說,它獲取原始 HTML 程式碼,讀取它,生成從段落到標題的 DOM 樹物件結構,並允許我們提取重要或需要的資訊。
我們使用內建庫解析 HTML 檔案,有時使用第三方庫來進行網頁抓取或 PHP 內容分析。根據方法的不同,目標是將 HTML 文件正文轉換為字串以提取每個 HTML 標記。
本文將討論內建類 DomDocument()
和兩個第三方庫 simplehtmldom
和 DiDOM
。
使用 DomDocument()
在 PHP 中解析 HTML
無論是本地 HTML 檔案還是線上網頁,DOMDocument()
和 DOMXpath()
類都有助於解析 HTML 檔案並將其元素儲存為字串,或者在我們的示例中儲存為陣列。
讓我們使用函式解析這個 HTML 檔案並返回標題、子標題和段落。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h2 class="main">Welcome to the Abode of PHP</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Using PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Install PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Configure PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h2 class="main">Welcome to the Abode of JS</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding JS</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
</body>
</html>
PHP 程式碼:
<?php
$html = 'index.html';
function getRootElement($element, $html)
{
$dom = new DomDocument();
$html = file_get_contents($html);
$dom->loadHTML($html);
$dom->preserveWhiteSpace = false;
$content = $dom->getElementsByTagName($element);
foreach ($content as $each) {
echo $each->nodeValue;
echo "\n";
}
}
echo "The H2 contents are:\n";
getRootElement("h2", $html);
echo "\n";
echo "The H3 contents are:\n";
getRootElement("h3", $html);
echo "\n";
echo "The Paragraph contents include\n";
getRootElement("p", $html);
echo "\n";
程式碼片段的輸出是:
The H2 contents are:
Welcome to the Abode of PHP
Welcome to the Abode of JS
The H3 contents are:
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
The Paragraph contents include
PHP has been the saving grace of the internet from its inception, it
runs over 70% of the website on the internet
...
在 PHP 中使用 simplehtmldom
解析 HTML
對於 CSS 樣式選擇器等附加功能,你可以使用名為 Simple HTML DOM Parser 的第三方庫,這是一個簡單快速的 PHP 解析器。你可以下載它幷包含或需要單個 PHP 檔案。
通過此過程,你可以輕鬆解析所需的所有元素。使用與上一節相同的程式碼片段,我們將使用名為 str_get_html()
的函式解析 HTML,該函式處理 HTML 並使用 find()
方法查詢特定的 HTML 元素或標記。
要查詢具有特殊 class
的元素,我們需要將 class
選擇器應用於每個 find
元素。此外,要找到實際文字,我們需要在元素上使用 innertext
選擇器,然後將其儲存在陣列中。
使用與上一節相同的 HTML 檔案,讓我們使用 simplehtmldom
解析它。
<?php
require_once('simple_html_dom.php');
function getByClass($element, $class)
{
$content= [];
$html = 'index.html';
$html_string = file_get_contents($html);
$html = str_get_html($html_string);
foreach ($html->find($element) as $element) {
if ($element->class === $class) {
array_push($heading, $element->innertext);
}
}
print_r($content);
}
getByClass("h2", "main");
getByClass("p", "special");
程式碼片段的輸出是:
Array
(
[0] => Welcome to the Abode of PHP
[1] => Welcome to the Abode of JS
)
Array
(
[0] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
[1] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
)
在 PHP 中使用 DiDOM
解析 HTML
對於這個第三方 PHP 庫,我們必須使用一個名為 Composer 的 PHP 依賴項管理器,它允許我們管理所有 PHP 庫和依賴項。DiDOM
庫可通過 GitHub 獲得,它提供比其他庫更高的速度和記憶體管理。
如果沒有,可以安裝在這裡安裝 Composer。但是,如果你有,以下命令會將 DiDOM
庫新增到你的專案中。
composer require imangazaliev/didom
之後,你可以使用下面的程式碼,它與 simplehtmldom
的結構類似,帶有 find()
方法。有一個 text()
,它將 HTML 元素上下文轉換為我們可以在程式碼中使用的字串。
has()
函式允許你檢查 HTML 字串中是否有元素或類,並返回一個布林值。
<?php
use DiDom\Document;
require_once('vendor/autoload.php');
$html = 'index.html';
$document = new Document('index.html', true);
echo "H3 Element\n";
if ($document->has('h3')) {
$elements = $document->find('h3');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
echo "\nElement with the Class 'main'\n";
if ($document->has('.main')) {
$elements = $document->find('.main');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
程式碼片段的輸出是:
H3 Element
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
Element with the Class 'main'
Welcome to the Abode of PHP
Welcome to the Abode of JS
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn