在 PHP 中調整影象大小
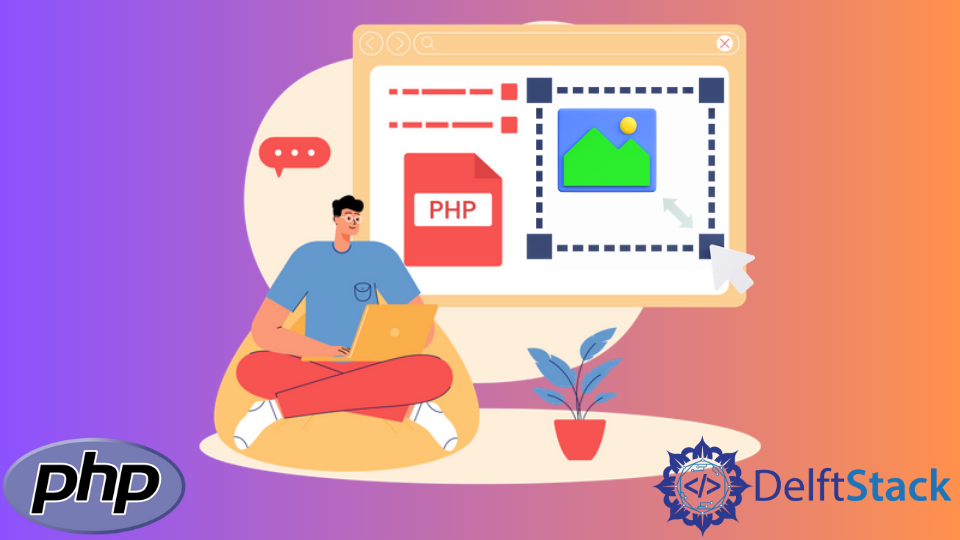
在本教程文章中,我們將討論在 PHP 中調整影象大小。
在調整大小之前載入影象
在調整影象大小之前,我們必須首先將其作為指令碼中的影象資源載入。這與使用 file_get_contents()
之類的函式來獲取影象檔案的內容不同。要載入檔案,我們需要使用 imagecreatefromjpeg()
、imagecreatefrompng()
和 imagecreatefromgif()
等函式。根據我們將調整大小的影象型別,我們將相應地使用不同的函式。
PHP 中的 getimagesize()
載入影象後,我們使用 getimagesize()
來計算輸入影象的寬度、高度和型別。此函式返回一個專案列表,其中影象的寬度和高度分別儲存在索引 0 和 1 處,IMAGETYPE_XXX
常量儲存在索引 2 處。我們將使用返回的這個常量的值來找出什麼要使用的影象型別以及要使用的功能。
<?php
function load_image($filename, $type) {
$new = 'new34.jpeg';
if( $type == IMAGETYPE_JPEG ) {
$image = imagecreatefromjpeg($filename);
echo "here is jpeg output:";
imagejpeg($image, $new);
}
elseif( $type == IMAGETYPE_PNG ) {
$image = imagecreatefrompng($filename);
echo "here is png output:";
imagepng($image,$new);
}
elseif( $type == IMAGETYPE_GIF ) {
$image = imagecreatefromgif($filename);
echo "here is gif output:";
imagejpeg($image, $new);
}
return $new;
}
$filename = "panda.jpeg";
list($width, $height,$type) = getimagesize($filename);
echo "Width:" , $width,"\n";
echo "Height:", $height,"\n";
echo "Type:", $type, "\n";
$old_image = load_image($filename, $type);
?>
輸入影象:
輸出:
Width:225
Height:225
Type:2
here is jpeg output:
輸出影象:
兩者是相同的影象,因為我們在本節中只載入影象並計算原始影象的大小。
PHP 中的 imagecopyresized()
imagecopyresized()
從位置 (src_x,src_y)
處的 src_image
獲取寬度為 src_w
和高度為 src_h
的長方形區域,並將其放置在位置 (dst_x,dst_y) 處的目標影象的矩形區域中
.它是 PHP 中的內建函式。
<?php
// File and new size
$filename = 'panda.jpeg';
$percent = 0.5;
// Content type
header('Content-Type: image/jpeg');
// Get new sizes
list($width, $height, $type) = getimagesize($filename);
$newwidth = $width * $percent;
$newheight = $height * $percent;
$type = $list[2];
echo $type;
// Load
$thumb = imagecreatetruecolor($newwidth, $newheight);
$source = imagecreatefromjpeg($filename);
// Resize
imagecopyresized($thumb, $source, 0, 0, 0, 0, $newwidth, $newheight, $width, $height);
// Output
imagejpeg($thumb,'new.jpeg');
?>
輸入影象:
輸出影象:
如上所述,imagecopyresize()
函式接受十個引數,如下所列。
引數 | 解釋 |
---|---|
$dst_image |
目標影象資源 |
$src_image |
源影象資源 |
$dst_x |
目標點的 x 座標 |
$dst_y |
目標點的 y 座標 |
$src_x |
源點的 x 座標 |
$src_y |
源點的 y 座標 |
$dst_w |
目的地寬度 |
$dst_h |
目的地高度 |
$src_w |
源寬度 |
$src_h |
源高度 |
它在成功時返回布林值 TRUE,在失敗時返回 FALSE。
PHP 中的 imagecopyresampled()
imagecopyresampled()
將一幅影象的長方形部分複製到另一個不同的、無縫插值的畫素值,以減少圖片的尺寸,保持高度的清晰度。
它的功能類似於 imagecopyresized()
功能,除了調整影象大小外,還具有對影象進行取樣的額外好處。
<?php
// The file
$filename = 'panda.jpeg';
$percent = 2;
// Content type
header('Content-Type: image/jpeg');
// Get new dimensions
list($width, $height) = getimagesize($filename);
$new_width = $width * $percent;
$new_height = $height * $percent;
// Resample
$image_p = imagecreatetruecolor($new_width, $new_height);
$image = imagecreatefromjpeg($filename);
imagecopyresampled($image_p, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height);
// Output
imagejpeg($image_p, 'resampled1.jpeg', 100);
?>
輸入影象:
輸出影象:
函式 imagecopyresampled()
接受十個不同的引數。
引數 | 解釋 |
---|---|
$image_p |
它確定目標影象。 |
$image |
它確定源影象。 |
$x_dest |
它確定目標影象的 x 座標。 |
$y_dest |
它確定目標影象的 y 座標。 |
$x_src |
它確定源影象的 x 座標。 |
$y_src |
它確定源影象的 y 座標。 |
$wid_dest |
它確定新影象的寬度。 |
$hei_dest |
它確定新影象的高度。 |
$wid_src |
它確定舊影象的寬度。 |
$wid_src |
它確定舊影象的高度。 |
dest_image
的寬度和高度而不是百分比來實現。PHP 中的 imagescale()
你指定大小而不是定義最新影象的寬度或高度。如果你希望新影象大小是第一張影象的一半,請將大小設定為 0.8。這是在保留比例的同時按給定因子縮放圖片的示例程式碼。
我們將影象的原始寬度和高度與 scale image()
函式中的指定比例相乘。
這是它的例子:
<?php
function load_image($filename, $type) {
if( $type == IMAGETYPE_JPEG ) {
$image = imagecreatefromjpeg($filename);
}
elseif( $type == IMAGETYPE_PNG ) {
$image = imagecreatefrompng($filename);
}
elseif( $type == IMAGETYPE_GIF ) {
$image = imagecreatefromgif($filename);
}
return $image;
}
function scale_image($scale, $image, $width, $height) {
$new_width = $width * $scale;
$new_height = $height * $scale;
return resize_image($new_width, $new_height, $image, $width, $height);
}
function resize_image($new_width, $new_height, $image, $width, $height) {
$image12 = 'hello.jpeg';
$new_imag = imagecreatetruecolor($new_width, $new_height);
imagecopyresampled($new_imag, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height);
return imagejpeg($new_imag, $image12);
}
$filename = "panda.jpeg";
list($width, $height, $type) = getimagesize($filename);
$old_image = load_image($filename, $type);
$image_scaled = scale_image(0.8, $old_image, $width, $height);
?>
輸入影象:
輸出影象:
除此之外,PHP 有一個內建功能,允許你將影象縮放到指定的寬度和高度。
請參閱示例程式碼。
<?php
$out_image = "sca.jpeg";
$image_name ="panda.jpeg";
// Load image file
$image = imagecreatefromjpeg($image_name);
// Use imagescale() function to scale the image
$img = imagescale( $image, 500, 400 );
header("Content-type: image/jpeg");
imagejpeg($img,$out_image);
?>
輸入影象:
輸出_影象: