在 PHP 中比較日期的不同方法
-
用
strtotime()
和time()
比較日期 -
使用
Date()
函式和條件檢查比較日期 - 使用自定義函式比較日期
-
使用 PHP
Datetime
物件比較日期 -
Arsort()
和strtotime()
進行多個日期的比較
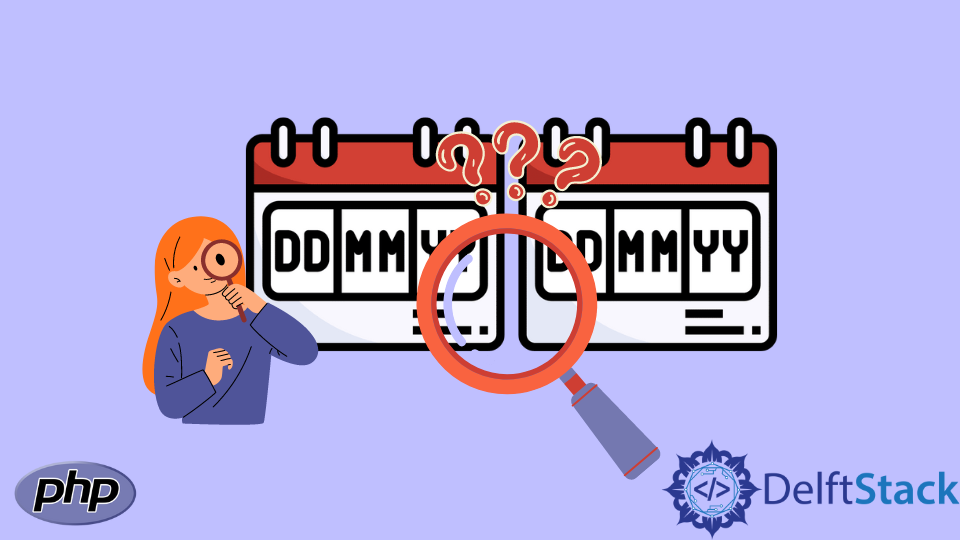
本文將教你如何使用 5 種不同的技術在 PHP 中比較日期。在這些技術中,有 4 種將以一種或另一種形式使用內建的 PHP 函式,如 strtotime()
、time()
和 date()
。最後一種技術將使用 PHP DateTime
物件。
用 strtotime()
和 time()
比較日期
strtotime()
是 PHP 中內建的時間函式。它的主要用途是將一串人類可讀的日期轉換為相等的 UNIX 時間戳。它可以解析各種字串並將它們轉換為適當的時間戳。此類字串的示例是 2 weeks ago
和 next week
。
PHP time()
函式返回當前時間。這個時間是自 UNIX 紀元以來的秒數。如果你需要將這些秒數轉換為當前日期,則需要 PHP 的內建 date()
函式。
結合這兩個功能時,你可以使用以下步驟比較日期。
首先,你向 strtotime()
提供一個日期字串。它將把它轉換成它的 UNIX 時間戳。
使用 time()
函式從當前時間中減去此 UNIX 時間戳。
你可以使用此計算的結果和條件檢查來比較日期。
<?php
$dateString = '2021-12-12 21:00:00';
// This will check if the time
// is in the last day
if ((time() - (60 * 60 * 24)) < strtotime($dateString)) {
echo "Date in the last 24hrs";
} else {
echo "Date is <b>NOT</b> in the last 24hrs";
}
?>
輸出:
Date is <b>NOT</b> in the last 24hrs
使用 Date()
函式和條件檢查比較日期
PHP 的 date()
函式允許你將時間戳格式化為你想要的格式。有問題的時間戳是當前時間和 UNIX 紀元之間的秒數。UNIX 紀元是從 1970 年 1 月 1 日午夜開始的時間。
date() 函式的值取決於你的 php.ini
檔案中設定的時區。
如果要使用 date()
函式比較給定日期,請執行以下操作。
- 將當前時間戳轉換成你想要的格式
- 在適當的字串中寫下你想要比較的日期
- 在日期字串和當前時間戳之間進行條件檢查
<?php
$todaysDate = date("Y-m-d H:i:s");
$dateString = "2021-10-12 10:00:00";
if ($dateString < $todaysDate) {
echo "The date you supplied is LESS than the current date";
} else {
echo "The date you supplied is NOT LESS than the current date";
}
?>
輸出:
The date you supplied is LESS than the current date
使用自定義函式比較日期
你使用函式來避免程式碼重複。本教程中的第一個示例展示瞭如何將日期與 strtotime()
和 time()
進行比較。
在這種情況下,你將建立一個函式。此函式將接受日期字串作為引數,然後將其傳遞給 strtotime()
。你將字串 "today"
傳遞給函式內的另一個 strtotime()
。
現在,你有兩件事可以比較,它們是,
- 你想比較的時間
- 字串
today
傳遞給另一個strtotime()
你可以使用嚴格比較來將日期與這些標準進行比較。你可以建立一個比較函式來檢查以下內容。
- 今天的日期
- 過去的日期
- 未來的日期
以下將檢查提供的日期字串是否為當前日期:
<?php
function checkToday($time) {
$convertToUNIXtime = strtotime($time);
$todayUNIXtime = strtotime('today');
return $convertToUNIXtime === $todayUNIXtime;
}
if (checkToday('2021-12-13')) {
echo "Yeah it's today";
} else {
echo "No, it's not today";
}
?>
輸出:
No, it's not today
檢查過去的日期。
<?php
function checkThePast($time) {
$convertToUNIXtime = strtotime($time);
return $convertToUNIXtime < time();
}
if (checkThePast('2021-12-13 22:00:00')) {
echo "The date is in the past";
} else {
echo "No, it's not in the past";
}
?>
輸出:
The date is in the past
你可以通過以下方式檢查未來的日期。
<?php
function checkTheFuture($time) {
$convertToUNIXtime = strtotime($time);
return $convertToUNIXtime > time();
}
if (checkTheFuture('2021-12-13 22:00:00')) {
echo "The date is in the future";
} else {
echo "No, it's not in the future";
}
?>
輸出:
No, it's not in the future
使用 PHP Datetime
物件比較日期
PHP DateTime
類為你提供了一種物件導向的方式來處理 PHP 中的日期字串。它有一套你可以使用的方法。它封裝了場景背後的一些邏輯,併為你提供了一個乾淨的介面來使用。
與 strtotime()
和 date()
相比,DateTime
具有以下優點:
- 可以處理更多的日期字串
- 使用起來更容易
- 它提供了日期的直接比較
當你打算將日期與 DateTime
進行比較時,請執行以下操作。
- 將日期字串解析為
DateTime
物件 - 將日期與小於 ( < )、大於 ( > ) 或等於 ( == ) 等運算子進行比較
<?php
$firstDate = new DateTime("2020-12-13");
$secondDate = new DateTime("2021-12-13");
// Compare the date using operators
if ($firstDate > $secondDate) {
echo "First date is GREATER than the second date";
} else if ($firstDate < $secondDate) {
echo "First date is LESS than the second date";
} else {
echo "First and second dates are EQUAL";
}
?>
輸出:
First date is LESS than the second date
Arsort()
和 strtotime()
進行多個日期的比較
如果要比較多個日期字串,可以將它們儲存在陣列中。
使用陣列中的日期,你可以使用合適的迴圈方法來處理陣列。例如,foreach
。
在 foreach
內的迴圈中,你可以使用 strtotime()
將每個日期轉換為它們的 UNIX 時間戳。之後,你可以使用 arsort()
對日期進行排序。
<?php
// Store the dates
$dateArray = ["2020-09-30", "2021-12-13", "2021-08-05"];
// Convert each date to a UNIX timestamp
// and store them in another array
$dateArrayTimestamp = [];
foreach ($dateArray as $date) {
$dateArrayTimestamp[] = strtotime($date);
}
// Sort the dates,
arsort($dateArrayTimestamp);
// Print the date starting with the
// current date
foreach ($dateArrayTimestamp as $key => $value) {
echo "$key - " . date("Y - m - d", $value) . "<br>";
}
?>
輸出:
1 - 2021 - 12 - 13<br>2 - 2021 - 08 - 05<br>0 - 2020 - 09 - 30<br>
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn