PHP 中已棄用的 Mysql_connect 的解決方案
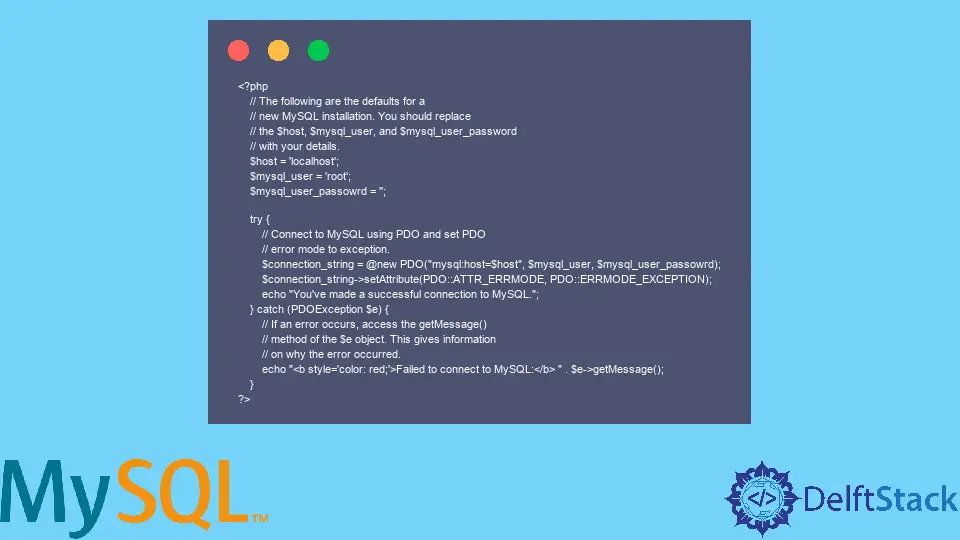
本文將教你解決 PHP 中已棄用的 mysql_connect
的解決方案。這些解決方案包括 mysqli_connect
(過程和 OOP)和 PHP 資料物件 (PDO)。
使用程式 Mysqli_connect
連線到 MySQL
mysqli_connect
允許你使用過程程式設計連線到你的 MySQL 資料庫。因此,你可以使用以下演算法:
-
定義資料庫連線詳細資訊。
-
使用
mysqli_connect
連線。 -
如果連線成功,則顯示成功訊息。
-
如果連線失敗,則顯示錯誤訊息。
在步驟 2 中發生錯誤之前,此演算法工作正常。此類錯誤可能是不正確的資料庫詳細資訊。
當 PHP 遇到這些不正確的細節時,它會丟擲一個 Fatal Error
異常。因此,程式碼不會進入第 3 步或第 4 步,你將在其中顯示錯誤訊息。
為了解決這個問題,我們必須關閉 MySQL 錯誤報告並禁止警告。通過這樣做,當發生錯誤時,你將阻止使用者看到敏感細節,同時,你可以顯示自定義錯誤訊息。
話雖如此,以下是修改後的演算法:
-
定義資料庫連線詳細資訊。
-
關閉 MySQL 錯誤報告。
-
使用
mysqli_connect
連線並抑制警告。 -
如果連線成功,則顯示成功訊息。
-
如果連線失敗,則顯示錯誤訊息。
下面是這個演算法的實現:
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
// Turn off error reports like "Fatal Errors".
// Such reports can contain too much sensitive
// information that no one should see. Also,
// turning off error reports allows us to handle
// connection error in an if/else statement.
mysqli_report(MYSQLI_REPORT_OFF);
// If there is an error in the connection string,
// PHP will produce a Warning message. For security
// and private reasons, it's best to suppress the
// warnings using the '@' sign
$connect_to_mysql = @mysqli_connect($host, $mysql_user, $mysql_user_passowrd);
if (!$connect_to_mysql) {
echo "<b style='color: red;'>Failed to connect to MySQL.</b>";
} else {
echo "You've made a successful connection to MySQL.";
}
?>
成功連線的輸出:
You've made a successful connection to MySQL.
連線失敗的輸出:
<b style='color: red;'>Failed to connect to MySQL.</b>
使用物件導向程式設計連線到 MySQL
使用 OOP 和 mysqli_connect
,你可以使用 new mysqli()
建立資料庫連線。與程式技術一樣,你必須將連線詳細資訊傳遞給 new mysqli()
。
但是,這些細節中可能存在錯誤,因此我們將使用 try...catch
塊進行錯誤處理。首先,我們將連線詳細資訊放在 try
塊中,如果發生錯誤,我們將在 catch
塊中捕獲它們。
在下文中,與 MySQL 的連線使用 OOP 版本的 mysqli_connect
。你還會注意到我們已經抑制了連線字串中的錯誤。
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to the database using the OOP style
// of mysqli.
$connection_string = @new mysqli($host, $mysql_user, $mysql_user_passowrd);
echo "You've made a successful connection to MySQL.";
} catch (Exception $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL :</b> " . $e->getMessage();
}
?>
成功連線的輸出:
You've made a successful connection to MySQL.
連線失敗的輸出:
<b style='color: red;'>Failed to connect to MySQL :</b> Access denied for user ''@'localhost' (using password: NO)
使用 PDO 連線到 MySQL
你可以使用 PDO 連線到 MySQL,並且你應該使用 try...catch
塊捕獲錯誤。後者的工作方式與你在 OOP 部分中學習的方式相同。
此外,當錯誤發生時,你的使用者不會看到敏感的錯誤訊息。現在,下面是連線 MySQL 的 PDO 版本:
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to MySQL using PDO and set PDO
// error mode to exception.
$connection_string = @new PDO("mysql:host=$host", $mysql_user, $mysql_user_passowrd);
$connection_string->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "You've made a successful connection to MySQL.";
} catch (PDOException $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL:</b> " . $e->getMessage();
}
?>
連線成功的輸出:
You've made a successful connection to MySQL.
連線失敗的輸出:
Failed to connect to MySQL: SQLSTATE[HY000] [1045] Access denied for user 'root'@'localhost' (using password: YES)
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn