如何使用 Matplotlib 實時繪製資料
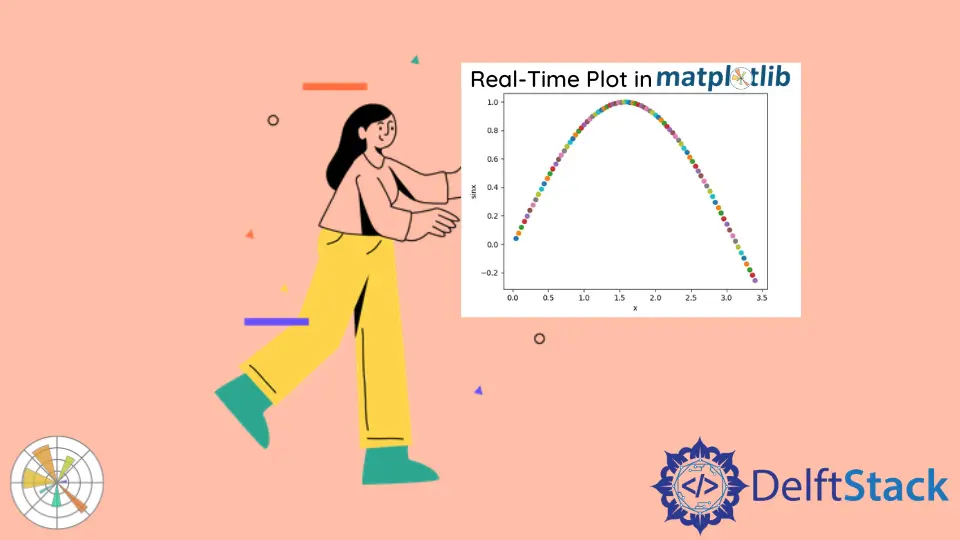
為了使用 Matplotlib 實時繪製資料或在 Matplotlib 中製作動畫,我們不斷地通過迴圈迭代然後繪製更新後的值來更新要繪製的變數。為了通過動畫實時檢視更新的繪圖,我們使用了各種方法,例如 FuncAnimation()
函式,canvas.draw()
和 canvas_flush_events()
。
FuncAnimation()
函式
我們可以通過更新變數 x
和 y
然後使用 matplotlib.animation.FuncAnimation
通過動畫顯示更新來實時更新圖。
語法:
matplotlib.animation.FuncAnimation(fig,
func,
frames=None,
init_func=None,
fargs=None,
save_count=None,
*,
cache_frame_data=True,
**kwargs)
程式碼:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
x = []
y = []
figure, ax = plt.subplots(figsize=(4, 3))
(line,) = ax.plot(x, y)
plt.axis([0, 4 * np.pi, -1, 1])
def func_animate(i):
x = np.linspace(0, 4 * np.pi, 1000)
y = np.sin(2 * (x - 0.1 * i))
line.set_data(x, y)
return (line,)
ani = FuncAnimation(figure, func_animate, frames=10, interval=50)
ani.save(r"animation.gif", fps=10)
plt.show()
ani = FuncAnimation(figure, func_animate, frames=10, interval=50)
figure
是圖形物件,其圖將被更新。
func_animate
是在每一幀都要呼叫的函式。它的第一個引數來自下一個值 frames
。
frames=10
等於 range(10)
。每幀將 0 到 9 的值傳遞給 func_animate
。我們還可以為 interframe 分配一個幀,例如列表 [0, 1, 3, 7, 12]
。
間隔是指以毫秒為單位的幀之間的延遲。
ani.save("animation.gif", fps=10)
我們可以使用諸如 fps
和 dpi
之類的引數將動畫儲存為 gif
或 mp4
。
canvas.draw()
和 canvas_flush_events()
我們可以通過使用 set_xdata()
和 set_ydata()
更新變數 x 和 y 來實時更新繪圖,然後使用 canvas.draw()
通過動畫顯示更新,它是 JavaScript 的方法。
import numpy as np
import time
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.cos(x)
plt.ion()
figure, ax = plt.subplots(figsize=(8, 6))
(line1,) = ax.plot(x, y)
plt.title("Dynamic Plot of sinx", fontsize=25)
plt.xlabel("X", fontsize=18)
plt.ylabel("sinX", fontsize=18)
for p in range(100):
updated_y = np.cos(x - 0.05 * p)
line1.set_xdata(x)
line1.set_ydata(updated_y)
figure.canvas.draw()
figure.canvas.flush_events()
time.sleep(0.1)
在這裡,x
和 y
的值被重複更新,並且圖也被實時更新。
plt.ion()
開啟互動模式。如果未呼叫該圖,則不會更新。
canvas.flush_events()
是一種基於 JavaScript 的方法,用於在每次迭代時清除圖形,以使後續圖形不會重疊。
Matplotlib 實時散點圖
但是,要畫實時分散圖,我們只需更新 x 和 y 的值並在每次迭代中新增分散點即可。在這種情況下,我們不需要清除每個圖形,因為散點圖通常表示平面中的一個不同點,並且這些點幾乎沒有重疊的機會。
import numpy as np
import matplotlib.pyplot as plt
x = 0
for i in range(100):
x = x + 0.04
y = np.sin(x)
plt.scatter(x, y)
plt.title("Real Time plot")
plt.xlabel("x")
plt.ylabel("sinx")
plt.pause(0.05)
plt.show()
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn