如何在 Matplotlib 中自動執行圖更新
Suraj Joshi
2023年1月30日
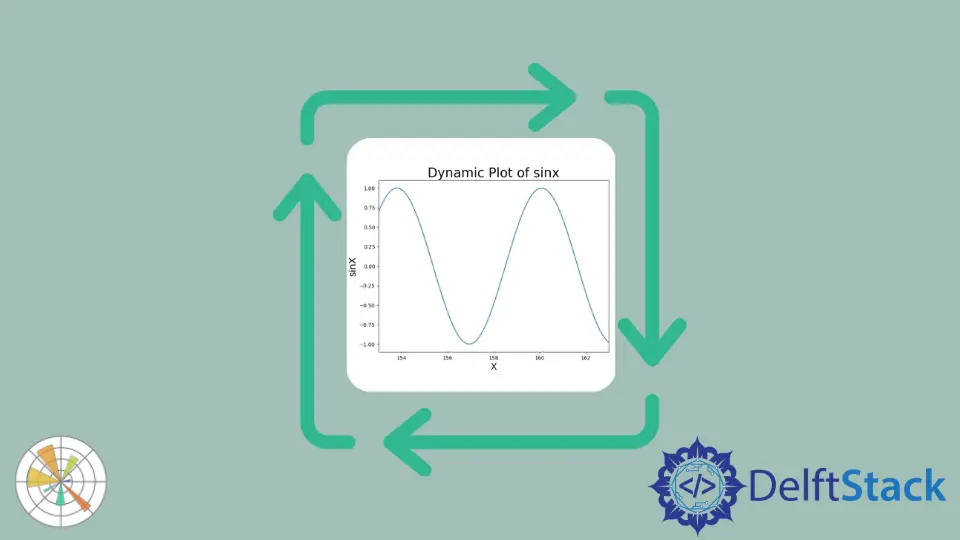
為了在 Matplotlib 中自動執行圖更新,我們將更新資料,清除現有圖,然後在迴圈中繪製更新的資料。為了清除現有的繪圖,我們使用了幾種方法,例如 canvas.draw()和 canvas_flush_events(),plt.draw()和 clear_output()。
canvas.draw()
和 canvas_flush_events()
我們需要配置圖形一次。然後,我們可以使用 set_xdata()
和 set_ydata()
更新繪圖物件的資料,最後使用 canvas.draw()
更新繪圖。
import numpy as np
import time
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.cos(x)
plt.ion()
figure, ax = plt.subplots(figsize=(8, 6))
(line1,) = ax.plot(x, y)
plt.title("Dynamic Plot of sinx", fontsize=25)
plt.xlabel("X", fontsize=18)
plt.ylabel("sinX", fontsize=18)
for p in range(100):
updated_y = np.cos(x - 0.05 * p)
line1.set_xdata(x)
line1.set_ydata(updated_y)
figure.canvas.draw()
figure.canvas.flush_events()
time.sleep(0.1)
plt.ion()
開啟互動模式。如果未呼叫,則該圖不會更新。
canvas.draw()
是一種基於 JavaScript 來顯示圖形的方法,而 canvas.flush_events()
也基於 JavaScript 來清除圖形。
plt.draw()
更新 Matplotlib 中的圖
我們使用 matplotlib.pyplot.draw()
函式來更新已更改的圖形,使我們能夠以互動方式工作。要更新圖,我們需要清除現有圖形可以使用 matplotlib.pyplot.clf()
和 matplotlib.axes.Axes.clear()
。
使用 plt.clf()
import numpy as np
import time
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.cos(x)
plt.ion()
figure, ax = plt.subplots(figsize=(8, 6))
(line1,) = ax.plot(x, y)
plt.title("Dynamic Plot of sinx", fontsize=25)
plt.xlabel("X", fontsize=18)
plt.ylabel("sinX", fontsize=18)
for p in range(100):
updated_y = np.cos(x - 0.05 * p)
line1.set_xdata(x)
line1.set_ydata(updated_y)
figure.canvas.draw()
figure.canvas.flush_events()
time.sleep(0.1)
輸出:
使用 fig.clear()
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.cos(x)
fig = plt.figure()
for p in range(50):
p = 3
updated_x = x + p
updated_y = np.cos(x)
plt.plot(updated_x, updated_y)
plt.draw()
x = updated_x
y = updated_y
plt.pause(0.2)
fig.clear()
輸出:
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn