在 Matplotlib 中建立彩色三角形
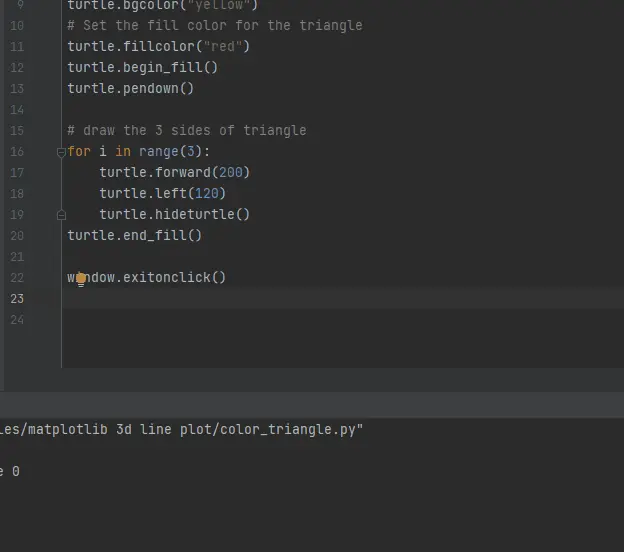
在本教程中,我們將使用 Python Matplotlib 程式碼為自己繪製一個簡單的彩色三角形。我們將使用 Python 附帶的 turtle
庫。
在 Matplotlib 中使用 Turtle 庫建立彩色三角形
為自己建立一個新的空白檔案,開始使用我們的彩色三角形。一旦我們建立了一個新的空白檔案,我們需要匯入 turtle
庫。
turtle
庫是由另一個程式編寫的程式碼,它使我們不必進行所有複雜的編碼以在螢幕上繪圖。匯入 turtle
庫後,我們將在螢幕上建立一個新的彈出視窗。
現在我們建立一個名為 window
的變數並訪問 turtle
庫以使用 Screen()
方法。
import turtle
# Set up the new blank window
window = turtle.Screen()
可以使用 turtle.bgcolor()
方法更改彈出螢幕的背景顏色。
turtle.bgcolor("yellow")
我們現在將使用 fillcolor()
方法為我們的三角形選擇填充顏色。之後,我們需要編寫 begin_fill()
方法來輸入我們將要繪製的需要用 fillcolor()
方法填充的形狀。
接下來,我們需要使用 pendown()
方法在螢幕上繪圖。
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
為了繪製這個三角形,我們將使用一個 for
迴圈。由於三角形有三個邊,我們將做 3 次。
forward()
方法將我們的 turtle 向前移動一段距離,我們可以在此方法中將距離作為整數傳遞。所以這是向前移動 200 畫素,然後使用 left()
方法將我們的 turtle 向左旋轉 120 度。
hideturtle()
方法幫助我們隱藏 turtle 的頭部。
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
接下來是我們跳出 for
迴圈,這樣我們就不再縮排了。我們將編寫 end_fill()
方法來停止用該顏色填充更多形狀。
最後,我們得到了由 turtle
庫繪製的三邊三角形。我們將編寫的最後一件事是當我們單擊視窗時退出視窗的 exitonclick()
方法。
turtle.end_fill()
window.exitonclick()
完整程式碼示例:
import turtle
# Set up the new blank window
window = turtle.Screen()
# Set the fill back ground color
turtle.bgcolor("yellow")
# Set the fill color for the triangle
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
turtle.end_fill()
window.exitonclick()
輸出:
點選這裡閱讀更多關於 turtle
庫的資訊。
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn