Kotlin 中的 Getter 和 Setter
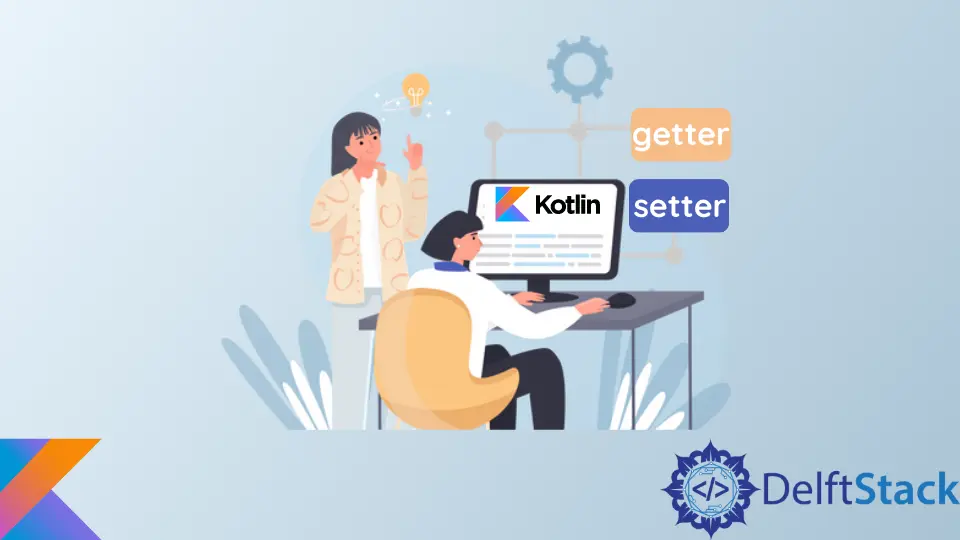
本文將介紹 Kotlin 中的 getter 和 setter 及其使用方法。我們還將演示一些示例來理解 getter 和 setter 的工作。
Kotlin 中的 Getter 和 Setter
在一般程式設計中,getter 幫助獲取屬性的值。同樣,設定屬性的值是使用 setter 完成的。
Kotlin 的 getter 和 setter 是自動生成的。因此,我們不需要為我們的程式單獨建立它們。
所以,如果我們寫,
class Student {
var Name: String = "John Doe"
}
上面的程式碼等價於下面的 Kotlin 程式。
class Student {
var Name: String = "John Doe"
get() = field
set(para) {
field = value
}
}
因此,當我們例項化一個類的物件時,如上例中的 Student
,並初始化一個屬性,setter
函式會自動將值設定為使用 getter
函式訪問的引數。
Kotlin 中的可變和不可變屬性
在 Kotlin 中,我們可以建立兩種型別的屬性:可變的和不可變的。
可變屬性使用由關鍵字 var
表示的可變變數宣告。不可變關鍵字使用由關鍵字 val
標記的不可更改變數來表示。
這些屬性之間的另一個區別是隻讀或不可變屬性不會建立設定器,因為它不需要更改任何內容。
這是一個基於上述語法在 Kotlin 中同時使用 get()
和 set()
函式的示例。
fun main(args: Array<String>) {
val v = Student()
v.Name = "John Doe"
println("${v.Name}")
}
class Student {
var Name: String = "defValue"
get() = field
set(defaultValue) {
field = defaultValue
}
}
輸出:
使用 Kotlin Set 作為私有修飾符
預設的 getter 和 setter 有一個 public 修飾符。如果我們想通過使用 private
關鍵字來使用帶有 private 修飾符的 setter,我們可以更改此設定。
通過宣告一個私有的 setter,我們只能通過類中的一個方法來設定一個值。
在本例中,我們將為 Student
類建立一個物件並訪問最初設定為 John Doe 的 Name 屬性。接下來,我們將通過類內的函式將名稱分配給 Jane Doe 並重新訪問它。
class Student () {
var Name: String = "John Doe"
private set
fun randFunc(n: String) {
Name = n
}
}
fun main(args: Array<String>) {
var s = Student()
println("Initially, name of the student is: ${s.Name}")
s.randFunc("Jane Doe")
println("Now, name of the student is: ${s.Name}")
}
輸出:
Kotlin 中的自定義 Getter 和 Setter
我們還可以在 Kotlin 中製作自定義的 getter 和 setter。這是一個示例,我們為屬性構建自定義的 get()
和 set()
方法。
class Student{
var Name: String = ""
get() {
println("We are in Name property's get()")
return field.toString()
}
set(defaultValue) {
println("We are in Name property's set()")
field = defaultValue
}
val ID: Int = 1013
get(){
println("We are in ID property's get()")
return field
}
}
fun main() {
val stu = Student()
stu.Name = "John Doe"
println("The age of ${stu.Name} is ${stu.ID}")
}
輸出:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn