在 JavaScript 中從字串中刪除子字串
Kirill Ibrahim
2023年1月30日
2021年1月22日
-
JavaScript
replace()
從字串中刪除特定子字串的方法 -
JavaScript
replace()
方法使用 Regex 從一個字串中刪除所有出現的特定子字串 -
從字串中提取特定子字串的 JavaScript
substr()
方法
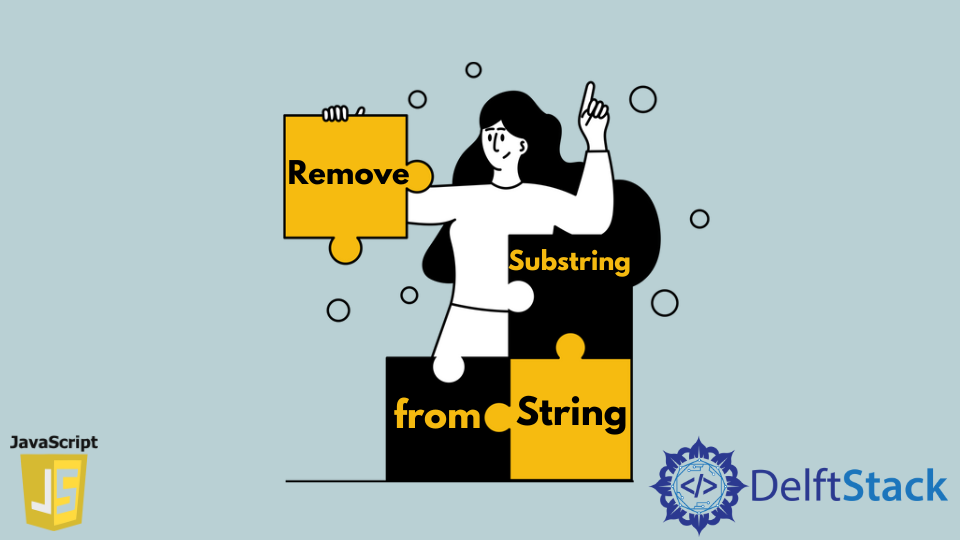
JavaScript 有兩種常用的方法來刪除字串中的子字串。下面的每個方法都會有一個程式碼示例,你可以在你的機器上執行。
JavaScript replace()
從字串中刪除特定子字串的方法
replace()
函式是 JavaScript 的一個內建函式。它用另一個字串或正規表示式替換給定字串的一部分。它從一個給定的字串中返回一個新的字串,並保持原來的字串不變。
replace()
語法
Ourstring.replace(Specificvalue, Newvalue)
Specificvalue
將被新的值-Newvalue
替換。
JavaScript replace()
方法示例
<!DOCTYPE html>
<html>
<head>
<title>
How to remove a substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStac for Software</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStac for Software';
ourNewWord = ourWord.replace('DelftStack', '');
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>
JavaScript replace()
方法使用 Regex 從一個字串中刪除所有出現的特定子字串
在全域性屬性中使用正規表示式代替 Specificvalue
。
例:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove to remove all occurrences of the specific substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStackforDelftStack</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStackforDelftStack';
ourNewWord = ourWord.replace(/DelftStack/g, '');
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>
從字串中提取特定子字串的 JavaScript substr()
方法
substr()
函式是 JavaScript 中的一個內建函式,用於從給定的字串中提取一個子字串或返回字串的一部分。它從指定的索引開始,並擴充套件給定數量的字元。
substr()
語法
string.substr(startIndex, length)
startIndex
是必需的。length
是可選的,是從該 Startindex
中選擇的字串長度,如果沒有指定,則提取到字串的其餘部分。
例:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove a substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStackforDelftStack</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStackforDelftStack';
ourNewWord = ourWord.substr(10,13);
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>